how do I fix: cannot access MidtermProblems bad source file: ./MidtermProblems.java import java.util.Scanner; public class ./MidtermProblems; { public static String replaceFirst(String Str,char c) { if(Str == null) return null; else if(Str == "") return ""; else { Str = Str.substring(0,0) + c + Str.substring(0 + 1);// modified string return Str; } } public static void main(String[] args) { //Here Str.substring(0,0) will remove the first charcter //And the c + Str.substring(0 + 1) will add the character c before the string (sub string starting from index 1 to last index) //Output - "casant" System.out.print( replaceFirst("basant",'c')); //New line character System.out.print("\n"); //Here null indicates no value (blank) and letter 't' will be added in that blank space System.out.print( replaceFirst(null,'t')); System.out.print("\n"); //If we put null in double quotes then it will be considered as a string //So again Str.substring(0,0) will remove the first charcter //And the c + Str.substring(0 + 1) will add the character c (value 't') before the string (sub string starting from index 1 to last index) // We will get "tull" as output System.out.print( replaceFirst("null",'t')); System.out.print("\n"); //Here Str.substring(0,0) will remove the first charcter //And the c + Str.substring(0 + 1) will add the character c (value 'm') before the string (sub string starting from index 1 to last index) //Output - "mice" System.out.print( replaceFirst("lice",'m')); System.out.print("\n"); //Here again Str.substring(0,0) will remove the first charcter //And the c + Str.substring(0 + 1) will add the character c ('t') before the string (sub string starting from index 1 to last index) //And here no characters are there after Index 0 in string "a" //Output - "t" System.out.print( replaceFirst("a",'t')); } }
how do I fix:
cannot access MidtermProblems
bad source file: ./MidtermProblems.java
import java.util.Scanner;
public class ./MidtermProblems;
{
public static String replaceFirst(String Str,char c)
{
if(Str == null)
return null;
else if(Str == "")
return "";
else
{
Str = Str.substring(0,0) + c + Str.substring(0 + 1);// modified string
return Str;
}
}
public static void main(String[] args)
{
//Here Str.substring(0,0) will remove the first charcter
//And the c + Str.substring(0 + 1) will add the character c before the string (sub string starting from index 1 to last index)
//Output - "casant"
System.out.print( replaceFirst("basant",'c'));
//New line character
System.out.print("\n");
//Here null indicates no value (blank) and letter 't' will be added in that blank space
System.out.print( replaceFirst(null,'t'));
System.out.print("\n");
//If we put null in double quotes then it will be considered as a string
//So again Str.substring(0,0) will remove the first charcter
//And the c + Str.substring(0 + 1) will add the character c (value 't') before the string (sub string starting from index 1 to last index)
// We will get "tull" as output
System.out.print( replaceFirst("null",'t'));
System.out.print("\n");
//Here Str.substring(0,0) will remove the first charcter
//And the c + Str.substring(0 + 1) will add the character c (value 'm') before the string (sub string starting from index 1 to last index)
//Output - "mice"
System.out.print( replaceFirst("lice",'m'));
System.out.print("\n");
//Here again Str.substring(0,0) will remove the first charcter
//And the c + Str.substring(0 + 1) will add the character c ('t') before the string (sub string starting from index 1 to last index)
//And here no characters are there after Index 0 in string "a"
//Output - "t"
System.out.print( replaceFirst("a",'t'));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

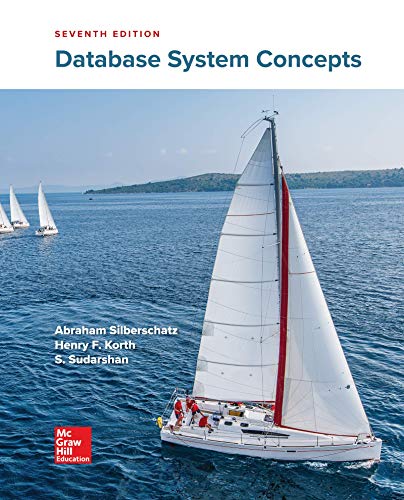
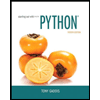
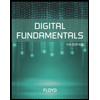
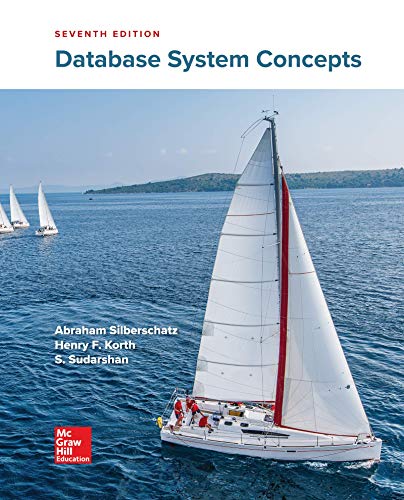
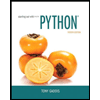
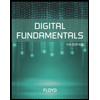
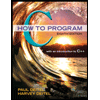
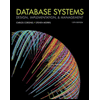
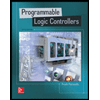