Here is a statement: class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; How do I write the definition of the member function set so that the instance variables are set according to the parameters?
Here is a statement:
class temporary
{
public:
void set(string, double, double);
void print();
double manipulate();
void get(string&, double&, double&);
void setDescription(string);
void setFirst(double);
void setSecond(double);
string getDescription() const;
double getFirst()const;
double getSecond()const;
temporary(string = "", double = 0.0, double = 0.0);
private:
string description;
double first;
double second;
};
How do I write the definition of the member function set so that the instance variables are set according to the parameters?

The question presents a programming task focused on defining a C++ class called temporary
. The objective is to create this class with specific member functions and attributes, and then provide a complete definition for one of the member functions.
Here's an introduction to the question:
Task Description:
You are tasked with designing and implementing a C++ class named temporary
. This class is intended to represent objects with a description, as well as two numeric values. The class should include member functions for setting and retrieving these attributes, among other functions.
Class Requirements:
The temporary
class should have the following attributes:
description
: A string representing a description for the object.first
: A double representing the first numeric value.second
: A double representing the second numeric value.
The class should include the following member functions:
- A constructor with default values for all attributes.
- A
set
function that sets thedescription
,first
, andsecond
attributes based on the provided arguments. - Functions for getting the
description
,first
, andsecond
attributes. - Additional member functions such as
print()
,manipulate()
, andget()
as needed.
Task:
Write the class definition for temporary
, including the constructor and the set
member function. The set
function should set the instance variables (description
, first
, and second
) according to the parameters provided.
Step by step
Solved in 3 steps

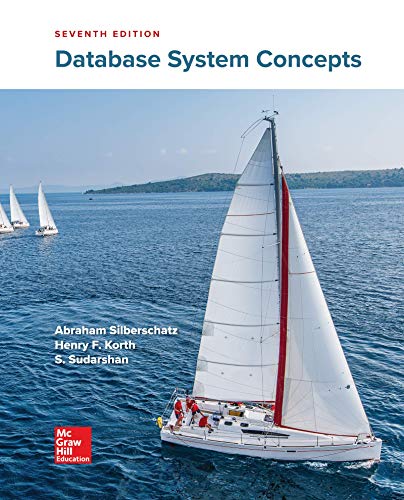
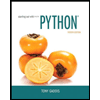
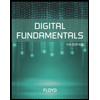
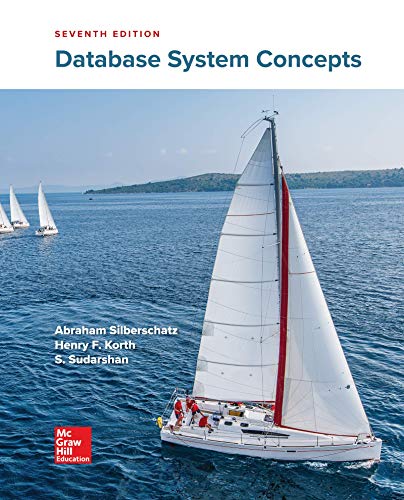
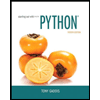
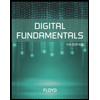
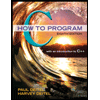
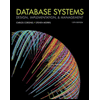
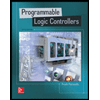