using System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); int randNo= r.Next(1,11); Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? "); int guess = Convert.ToInt32(Console.ReadLine()); if (guess == randNo) { Console.WriteLine("You guessed correctly!"); } else { Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again."); } } } this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?
using System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); int randNo= r.Next(1,11); Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? "); int guess = Convert.ToInt32(Console.ReadLine()); if (guess == randNo) { Console.WriteLine("You guessed correctly!"); } else { Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again."); } } } this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
using System;
class main
{
publicstaticvoid Main(string[] args)
{
Random r = new Random();
int randNo= r.Next(1,11);
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
if (guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
else
{
Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again.");
}
}
}
this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?
![### C# Program: Number Guessing Game
#### Code Explanation
This simple C# program generates a number guessing game. Let's break down the code and the output.
#### Code
```csharp
using System;
class main
{
public static void Main(string[] args)
{
Random r = new Random();
int randNo = r.Next(1, 11);
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
if (guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
else
{
Console.WriteLine("I was thinking of the number " + randNo + "\nSorry! Play again.");
}
}
}
```
#### Explanation
1. **Using Directive:**
```csharp
using System;
```
This statement includes the System namespace, which contains fundamental classes such as `Console`.
2. **Class Declaration:**
```csharp
class main
```
A class named `main` is declared. This is the blueprint for creating objects in C#.
3. **Main Method:**
```csharp
public static void Main(string[] args)
```
This is the entry point of the C# program. The `Main` method is where the program begins execution.
4. **Generating a Random Number:**
```csharp
Random r = new Random();
int randNo = r.Next(1, 11);
```
- `Random r = new Random();`: A `Random` object named `r` is created.
- `int randNo = r.Next(1, 11);`: The `Next` method generates a random number between 1 and 10 (inclusive), and the result is stored in `randNo`.
5. **Prompting User for Input:**
```csharp
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
```
This statement prompts the user to guess the number.
6. **Reading User Input:**
```csharp
int guess = Convert.ToInt32(Console.ReadLine());
```
- `Console](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a85ba4d-6f5c-41bf-97e5-182adb23b838%2Fe069ce75-da3c-4270-92a0-367044f75ce4%2Fnykeu_processed.png&w=3840&q=75)
Transcribed Image Text:### C# Program: Number Guessing Game
#### Code Explanation
This simple C# program generates a number guessing game. Let's break down the code and the output.
#### Code
```csharp
using System;
class main
{
public static void Main(string[] args)
{
Random r = new Random();
int randNo = r.Next(1, 11);
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
if (guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
else
{
Console.WriteLine("I was thinking of the number " + randNo + "\nSorry! Play again.");
}
}
}
```
#### Explanation
1. **Using Directive:**
```csharp
using System;
```
This statement includes the System namespace, which contains fundamental classes such as `Console`.
2. **Class Declaration:**
```csharp
class main
```
A class named `main` is declared. This is the blueprint for creating objects in C#.
3. **Main Method:**
```csharp
public static void Main(string[] args)
```
This is the entry point of the C# program. The `Main` method is where the program begins execution.
4. **Generating a Random Number:**
```csharp
Random r = new Random();
int randNo = r.Next(1, 11);
```
- `Random r = new Random();`: A `Random` object named `r` is created.
- `int randNo = r.Next(1, 11);`: The `Next` method generates a random number between 1 and 10 (inclusive), and the result is stored in `randNo`.
5. **Prompting User for Input:**
```csharp
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
```
This statement prompts the user to guess the number.
6. **Reading User Input:**
```csharp
int guess = Convert.ToInt32(Console.ReadLine());
```
- `Console
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
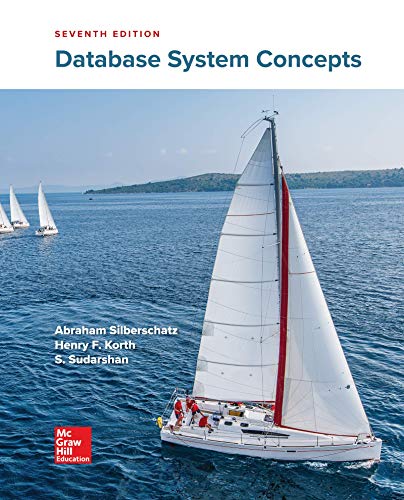
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
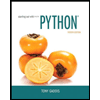
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
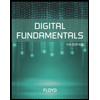
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
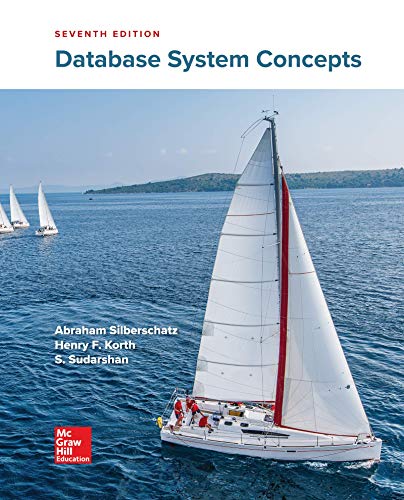
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
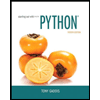
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
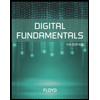
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
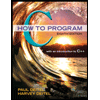
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
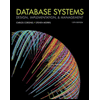
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
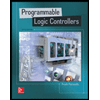
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education