Hello! This program is in C# and it's a guessing game. The user must choose a number between 1-10 and if the guess is wrong it will say if the guess is too high or too low. How can it be modified to guess a color on the rainbow (ROYGBIV)?
Hello! This program is in C# and it's a guessing game. The user must choose a number between 1-10 and if the guess is wrong it will say if the guess is too high or too low. How can it be modified to guess a color on the rainbow (ROYGBIV)?
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
using System;
class main
{
publicstaticvoid Main(string[] args)
{
Random r = new Random();
int randNo= r.Next(1,11);
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
do
{
if(guess<randNo)
{
Console.WriteLine("Your guess is too low.please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if(guess>randNo)
{
Console.WriteLine("Your guess is too high.please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if(guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
}while(guess!=randNo);
}
}
Hello! This program is in C# and it's a guessing game. The user must choose a number between 1-10 and if the guess is wrong it will say if the guess is too high or too low. How can it be modified to guess a color on the rainbow (ROYGBIV)?
![**Title: Simple Guessing Game in C#**
**Introduction:**
This tutorial demonstrates a simple number guessing game using C#. In this program, the computer generates a random number between 1 and 10, and the user tries to guess the number. The program provides hints if the guess is too high or too low until the correct number is guessed.
**Code Explanation:**
**1. Using Directives:**
```csharp
using System;
```
This line includes the System namespace, which contains fundamental classes for common functionalities.
**2. Class and Main Method:**
```csharp
class main
{
public static void Main(string[] args)
{
Random r = new Random();
int randNo = r.Next(1,11);
```
A class named `main` is defined with a `Main` method, which is the entry point of the program. Within this method, a `Random` object is created to generate random numbers. The `Next` method is used to generate a random number between 1 and 10.
**3. User Prompt:**
```csharp
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
```
The program prompts the user to guess the number, and the user's input is read and converted to an integer.
**4. Guessing Logic:**
```csharp
do
{
if (guess < randNo)
{
Console.WriteLine("Your guess is too low, please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if (guess > randNo)
{
Console.WriteLine("Your guess is too high, please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if (guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
} while (guess != randNo);
}
}
```
The guessing logic is implemented using a `do-while` loop. The loop continues until the user guesses the correct number. Inside the loop:
- If the guess is less than the random number, the program informs the user that the guess is too low and prompts for another guess.
- If the guess is greater than the random number, the program informs the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a85ba4d-6f5c-41bf-97e5-182adb23b838%2F4a4aa39f-1c01-4177-a1ca-070fc154b866%2Ftacrrwj_processed.png&w=3840&q=75)
Transcribed Image Text:**Title: Simple Guessing Game in C#**
**Introduction:**
This tutorial demonstrates a simple number guessing game using C#. In this program, the computer generates a random number between 1 and 10, and the user tries to guess the number. The program provides hints if the guess is too high or too low until the correct number is guessed.
**Code Explanation:**
**1. Using Directives:**
```csharp
using System;
```
This line includes the System namespace, which contains fundamental classes for common functionalities.
**2. Class and Main Method:**
```csharp
class main
{
public static void Main(string[] args)
{
Random r = new Random();
int randNo = r.Next(1,11);
```
A class named `main` is defined with a `Main` method, which is the entry point of the program. Within this method, a `Random` object is created to generate random numbers. The `Next` method is used to generate a random number between 1 and 10.
**3. User Prompt:**
```csharp
Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? ");
int guess = Convert.ToInt32(Console.ReadLine());
```
The program prompts the user to guess the number, and the user's input is read and converted to an integer.
**4. Guessing Logic:**
```csharp
do
{
if (guess < randNo)
{
Console.WriteLine("Your guess is too low, please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if (guess > randNo)
{
Console.WriteLine("Your guess is too high, please enter another number");
guess = Convert.ToInt32(Console.ReadLine());
}
if (guess == randNo)
{
Console.WriteLine("You guessed correctly!");
}
} while (guess != randNo);
}
}
```
The guessing logic is implemented using a `do-while` loop. The loop continues until the user guesses the correct number. Inside the loop:
- If the guess is less than the random number, the program informs the user that the guess is too low and prompts for another guess.
- If the guess is greater than the random number, the program informs the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 2 images

Recommended textbooks for you
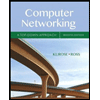
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
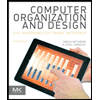
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
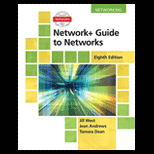
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
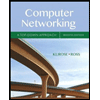
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
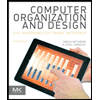
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
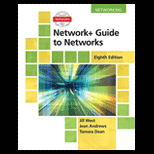
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
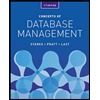
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
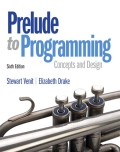
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
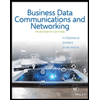
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY