TASK 5. Methods Overloading. Review Methods, Implement the following code, test it with different input, make sure it runs without errors.
TASK 5. Methods Overloading. Review Methods, Implement the following code, test it with different input, make sure it runs without errors.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
TASK 5. Methods Overloading. Review Methods, Implement the following code, test it with different input, make sure it runs without errors.
![3 import java.util.Scanner;
4
5 public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
60
// Prompt the user to enter the number of integers
System.out.println("Enter the number of integers you wil1 provide: ");
int num =
9
10
sc. nextInt();
11
12
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
13
14
15
int a =
sc. nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
16
17
18
19
if (num == 3) {
// Prompt the user to enter the third integer
System.out.println("Enter the value for 'c' and press Enter: ");
int c = sc.nextInt();
// Display the result
System.out.printf( "Provided Integers: %4d and %4d and %4d, the total is %5d\n", a, b, c, sum(a, b, c));
20
21
22
23
24
25
26
else {
// Display the result
System.out.printf( "Provided Integers: %4d and %4d, the total is %5d\n", a, b, sum(a, b));
}
}
public static int sum(int num1, int num2){
return num1 + num2;
}
public static int sum(int num1, int num2, int num3){
return num1 + num2 + num3;
29
30
31
320
33
34
35e
36
}
38 }
37
39
Our client just contacted us and said that they are planning from time to time provide us four integers instead
of two and three
Hint: What would be a universal/generic solution for dealing with such clients? Should we be proactive and
write methods with 5, 6, 7, etc. parameters in advance?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ec814c7-90f3-4435-9bb8-fcbab3f733ac%2F9ec55c54-996c-42f7-9508-80decfe00e97%2Fs2hl12_processed.png&w=3840&q=75)
Transcribed Image Text:3 import java.util.Scanner;
4
5 public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
60
// Prompt the user to enter the number of integers
System.out.println("Enter the number of integers you wil1 provide: ");
int num =
9
10
sc. nextInt();
11
12
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
13
14
15
int a =
sc. nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
16
17
18
19
if (num == 3) {
// Prompt the user to enter the third integer
System.out.println("Enter the value for 'c' and press Enter: ");
int c = sc.nextInt();
// Display the result
System.out.printf( "Provided Integers: %4d and %4d and %4d, the total is %5d\n", a, b, c, sum(a, b, c));
20
21
22
23
24
25
26
else {
// Display the result
System.out.printf( "Provided Integers: %4d and %4d, the total is %5d\n", a, b, sum(a, b));
}
}
public static int sum(int num1, int num2){
return num1 + num2;
}
public static int sum(int num1, int num2, int num3){
return num1 + num2 + num3;
29
30
31
320
33
34
35e
36
}
38 }
37
39
Our client just contacted us and said that they are planning from time to time provide us four integers instead
of two and three
Hint: What would be a universal/generic solution for dealing with such clients? Should we be proactive and
write methods with 5, 6, 7, etc. parameters in advance?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 5 images

Recommended textbooks for you
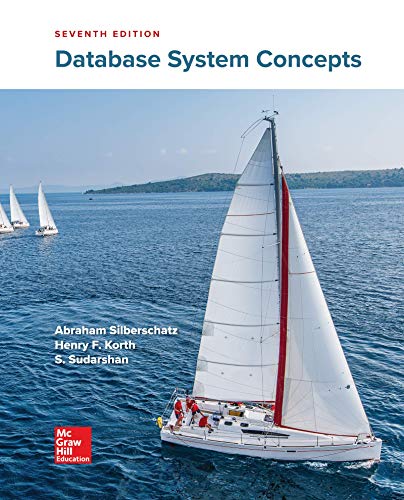
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
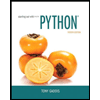
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
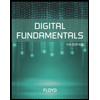
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
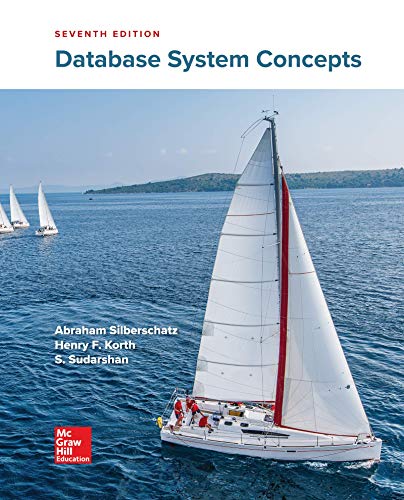
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
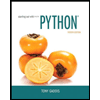
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
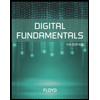
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
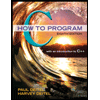
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
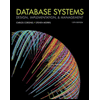
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
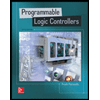
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education