Convert this Java code to C++ public class Problem4 { public static void main(String[] args) { // Scanner object for user input Scanner scanner = new Scanner(System.in); // user-menu while (true) { // prints the menu printMenu(); // user selection System.out.print("\nSelection: "); int selection = scanner.nextInt(); // printing appropriate definitions based on user selection switch (selection) { case 1: System.out.println("\nif: A conditional statement that, " + "if found true, runs the block of code inside it, else not.\n"); break; case 2: System.out.println("\nswitch: A control flow mechanism that allow variable/expression to change control flow of the program.\n"); break; case 3: System.out.println("\nfor: Allows us to iterate over a range of values.\n"); break; case 4: System.out.println("\nwhile: Runs the block of code till the specified condition is true.\n"); break; case 5: System.out.println("\ndo-while: It is just like a while loop with an additional do-statement which runs atleast once even when the while condition is evaluated to false.\n"); break; case 6: System.out.println("Thank you. Good bye."); return; default: System.out.println("Invalid selection. Try again..\n"); } } } // prints the user-menu public static void printMenu() { System.out.println("Java Help Menu"); System.out.println("Select Help or:"); System.out.println("1. If"); System.out.println("2. Switch"); System.out.println("3. For"); System.out.println("4. While"); System.out.println("5. Do-While"); System.out.println("6. Exit"); }}
Convert this Java code to C++
public class Problem4 {
public static void main(String[] args) {
// Scanner object for user input
Scanner scanner = new Scanner(System.in);
// user-menu
while (true) {
// prints the menu
printMenu();
// user selection
System.out.print("\nSelection: ");
int selection = scanner.nextInt();
// printing appropriate definitions based on user selection
switch (selection) {
case 1:
System.out.println("\nif: A conditional statement that, "
+ "if found true, runs the block of code inside it, else not.\n");
break;
case 2:
System.out.println("\nswitch: A control flow
break;
case 3:
System.out.println("\nfor: Allows us to iterate over a range of values.\n");
break;
case 4:
System.out.println("\nwhile: Runs the block of code till the specified condition is true.\n");
break;
case 5:
System.out.println("\ndo-while: It is just like a while loop with an additional do-statement which runs atleast once even when the while condition is evaluated to false.\n");
break;
case 6:
System.out.println("Thank you. Good bye.");
return;
default:
System.out.println("Invalid selection. Try again..\n");
}
}
}
// prints the user-menu
public static void printMenu() {
System.out.println("Java Help Menu");
System.out.println("Select Help or:");
System.out.println("1. If");
System.out.println("2. Switch");
System.out.println("3. For");
System.out.println("4. While");
System.out.println("5. Do-While");
System.out.println("6. Exit");
}
}

Step by step
Solved in 2 steps

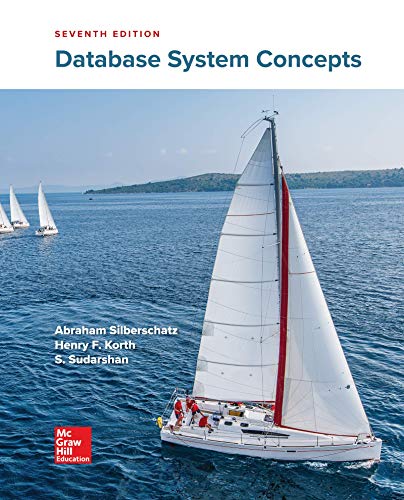
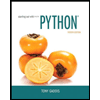
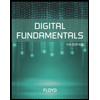
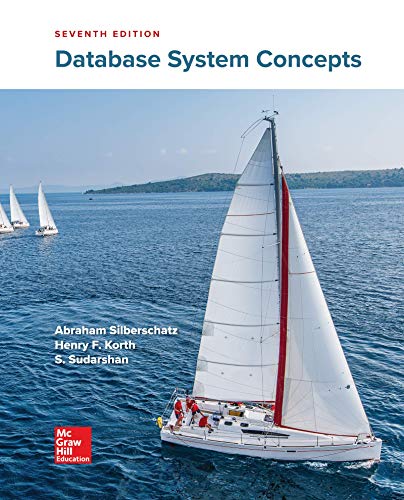
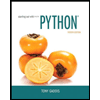
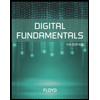
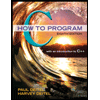
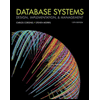
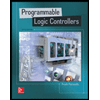