The Student Painters company paints dorm rooms. The rooms have one window and one door. The room is rectangular. The walls are always 8 feet tall. The doorway is 80 inches tall by 32 inches wide and the window is 4 feet by 5 feet. Student Painters charges $100 for labor plus the cost of the paint to paint a room. A gallon of paint costs $31.95 and will cover 300 square feet. All four walls (minus the window and door) and the ceiling are painted. Write a Paint Job class to model a paint job for Student Painters. The class has a constructor that takes the length and width of the room given as doubles in that order. Both length and width are in feet. • public Paint Job (double the Length, double theWidth) Do not do any calculations in the constructor. Just initialize the instance variables. Provide these methods: ● public double getLength () Gets the length of the room in feet. • public double getWidth () Gets the width of the room in feet. . public void setDimensions (double newLength, double newWidth) Sets a new length and width of the room in feet. • public double surfaceArea () Gets the surface area of the room in square feet, excluding the area of the door and the window. public double costOfPaint () Gets the cost of the paint for this job. Do not calculate surface area in this method. Call surfaceArea (). Charge for the fractional gallons used. The leftover paint can be used for another job. public double total JobCost () Gets the cost of this job. Do not calculate surface area or the cost of the paint in this method. Call methods to get the values. You will need to convert the square inches for the door to square feet. There are 144 square inches in a square foot. Here are some constants you must use public static final int SQ_INCHES_PER_SQ_FOOT = 144; public static final double WALL_HEIGHT_IN_FEET = 8; public static final double DOOR_HEIGHT_IN_INCHES = 80; public static final double DOOR_WIDTH_IN_INCHES = 32; public static final double WINDOW_HEIGHT_IN_FEET = 5; public static final double WINDOW WIDTH IN FEET = 4; You need to define and use constants for the cost of labor, the cost of a gallon of paint, and the number of square feet a gallon will cover. Make these constants accessible to any class. Do not use any magic numbers in the code, except number 2.
The Student Painters company paints dorm rooms. The rooms have one window and one door. The room is rectangular. The walls are always 8 feet tall. The doorway is 80 inches tall by 32 inches wide and the window is 4 feet by 5 feet. Student Painters charges $100 for labor plus the cost of the paint to paint a room. A gallon of paint costs $31.95 and will cover 300 square feet. All four walls (minus the window and door) and the ceiling are painted. Write a Paint Job class to model a paint job for Student Painters. The class has a constructor that takes the length and width of the room given as doubles in that order. Both length and width are in feet. • public Paint Job (double the Length, double theWidth) Do not do any calculations in the constructor. Just initialize the instance variables. Provide these methods: ● public double getLength () Gets the length of the room in feet. • public double getWidth () Gets the width of the room in feet. . public void setDimensions (double newLength, double newWidth) Sets a new length and width of the room in feet. • public double surfaceArea () Gets the surface area of the room in square feet, excluding the area of the door and the window. public double costOfPaint () Gets the cost of the paint for this job. Do not calculate surface area in this method. Call surfaceArea (). Charge for the fractional gallons used. The leftover paint can be used for another job. public double total JobCost () Gets the cost of this job. Do not calculate surface area or the cost of the paint in this method. Call methods to get the values. You will need to convert the square inches for the door to square feet. There are 144 square inches in a square foot. Here are some constants you must use public static final int SQ_INCHES_PER_SQ_FOOT = 144; public static final double WALL_HEIGHT_IN_FEET = 8; public static final double DOOR_HEIGHT_IN_INCHES = 80; public static final double DOOR_WIDTH_IN_INCHES = 32; public static final double WINDOW_HEIGHT_IN_FEET = 5; public static final double WINDOW WIDTH IN FEET = 4; You need to define and use constants for the cost of labor, the cost of a gallon of paint, and the number of square feet a gallon will cover. Make these constants accessible to any class. Do not use any magic numbers in the code, except number 2.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
/**
* A tester program for the PaintJob class.
*
public class PaintJobTester
{
public static void main(String[] args)
{
PaintJob job = new PaintJob(10.5, 9.0);
System.out.printf("Room length: %.1f%n", job.getLength());
System.out.println("Expected: 10.5");
System.out.printf("Room width: %.1f%n", job.getWidth());
System.out.println("Expected: 9.0");
System.out.printf("Surface area: %.2f%n", job.surfaceArea());
System.out.println("Expected: 368.72");
System.out.printf("Paint cost: %.2f%n", job.costOfPaint());
System.out.println("Expected: 39.27");
System.out.printf("Total cost: %.2f%n", job.totalJobCost());
System.out.println("Expected: 139.27");
job.setDimensions(15, 12);
System.out.printf("Room length: %.1f%n", job.getLength());
System.out.println("Expected: 15.0");
System.out.printf("Room width: %.1f%n", job.getWidth());
System.out.println("Expected: 12.0");
System.out.printf("Surface area: %.2f%n", job.surfaceArea());
System.out.println("Expected: 574.22");
System.out.printf("Paint cost: %.2f%n", job.costOfPaint());
System.out.println("Expected: 61.15");
System.out.printf("Total cost: %.2f%n", job.totalJobCost());
System.out.println("Expected: 161.15");
}
}
Here are some constants you must use
public static final int SQ_INCHES_PER_SQ_FOOT = 144;
public static final double WALL_HEIGHT_IN_FEET = 8;
public static final double DOOR_HEIGHT_IN_INCHES = 80;
public static final double DOOR_WIDTH_IN_INCHES = 32;
public static final double WINDOW_HEIGHT_IN_FEET = 5;
public static final double WINDOW_WIDTH_IN_FEET = 4;

Transcribed Image Text:The Student Painters company paints dorm rooms. The rooms have one window and one door.
The room is rectangular. The walls are always 8 feet tall. The doorway is 80 inches tall by 32
inches wide and the window is 4 feet by 5 feet. Student Painters charges $100 for labor plus the
cost of the paint to paint a room. A gallon of paint costs $31.95 and will cover 300 square feet.
All four walls (minus the window and door) and the ceiling are painted.
Write a PaintJob class to model a paint job for Student Painters.
The class has a constructor that takes the length and width of the room given as doubles in that
order. Both length and width are in feet.
Provide these methods:
●
●
public PaintJob (double the Length, double theWidth) Do not do any
calculations in the constructor. Just initialize the instance variables.
●
public double getLength () Gets the length of the room in feet.
public double getWidth () Gets the width of the room in feet.
public void setDimensions (double newLength, double
newWidth) Sets a new length and width of the room in feet.
public double surfaceArea () Gets the surface area of the room in square feet,
excluding the area of the door and the window.
public double costOfPaint () Gets the cost of the paint for this job. Do not
calculate surface area in this method. Call surfaceArea (). Charge for the fractional
gallons used. The leftover paint can be used for another job.
public double total JobCost () Gets the cost of this job. Do not calculate
surface area or the cost of the paint in this method. Call methods to get the values.
You will need to convert the square inches for the door to square feet. There are 144 square
inches in a square foot.
Here are some constants you must use
public static final int SQ_INCHES_PER_SQ_FOOT = 144;
public static final double WALL_HEIGHT_IN_FEET = 8;
public static final double DOOR_HEIGHT_IN_INCHES = 80;
public static final double DOOR_WIDTH_IN_INCHES = 32;
public static final double WINDOW_HEIGHT_IN_FEET = 5;
public static final double WINDOW_WIDTH_IN_FEET = 4;
You need to define and use constants for the cost of labor, the cost of a gallon of paint, and the
number of square feet a gallon will cover. Make these constants accessible to any class. Do not
use any magic numbers in the code, except number 2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
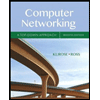
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
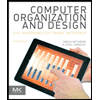
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
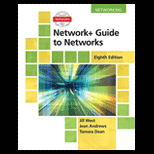
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
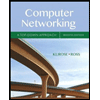
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
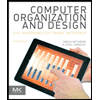
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
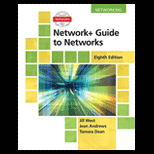
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
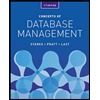
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
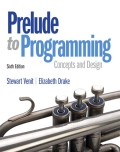
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
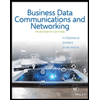
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY