Please create a flowchart for the following program: // Import Scanner class import java.util.Scanner; // Create a class Triangle public class Main { // Method used to check given three sides form a valid triangle or not public static boolean isTriangle(double a, double b, double c) { // If sum of any two sides is greater than third side if((a+b > c) && (a+c > b) && (b+c > a)) { // Print triangle is valid System.out.println("Three sides form a valid triangle."); // Return true return true; } // If any of the above condition is false else { // Print triangle is invalid System.out.println("Three sides form a invalid triangle.\n"); // Return false return false; } } // Method used to compute the area of triangle public static double triArea(double a, double b, double c) { // Compute s double s = (a + b + c) / 2; // Compute the area of triangle double area = Math.sqrt(s*(s - a)*(s - b)*(s - c)); // Return the area return area; } // Main method public static void main(String[] args) { // Create an object of Scanner class Scanner scan = new Scanner(System.in); // Loop till user enter while(true) { // Take three sides of triangle System.out.print("Enter three sides of triangle (or 0, 0, 0 to end): "); double a = scan.nextDouble(); double b = scan.nextDouble(); double c = scan.nextDouble(); // If the user enter 0,0,0 if(a == 0 && b == 0 && c == 0) // Exit from loop break; // If user enter any other value else { // Call methdo to check triangle is valid or not if(isTriangle(a, b, c)) { // Call function to find the area of triangle & print it System.out.printf("The area of triangle is: %.2f\n\n", triArea(a, b, c)); } } } } }
Please create a flowchart for the following
// Import Scanner class
import java.util.Scanner;
// Create a class Triangle
public class Main
{
// Method used to check given three sides form a valid triangle or not
public static boolean isTriangle(double a, double b, double c)
{
// If sum of any two sides is greater than third side
if((a+b > c) && (a+c > b) && (b+c > a))
{
// Print triangle is valid
System.out.println("Three sides form a valid triangle.");
// Return true
return true;
}
// If any of the above condition is false
else
{
// Print triangle is invalid
System.out.println("Three sides form a invalid triangle.\n");
// Return false
return false;
}
}
// Method used to compute the area of triangle
public static double triArea(double a, double b, double c)
{
// Compute s
double s = (a + b + c) / 2;
// Compute the area of triangle
double area = Math.sqrt(s*(s - a)*(s - b)*(s - c));
// Return the area
return area;
}
// Main method
public static void main(String[] args)
{
// Create an object of Scanner class
Scanner scan = new Scanner(System.in);
// Loop till user enter
while(true)
{
// Take three sides of triangle
System.out.print("Enter three sides of triangle (or 0, 0, 0 to end): ");
double a = scan.nextDouble();
double b = scan.nextDouble();
double c = scan.nextDouble();
// If the user enter 0,0,0
if(a == 0 && b == 0 && c == 0)
// Exit from loop
break;
// If user enter any other value
else
{
// Call methdo to check triangle is valid or not
if(isTriangle(a, b, c))
{
// Call function to find the area of triangle & print it
System.out.printf("The area of triangle is: %.2f\n\n", triArea(a, b, c));
}
}
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

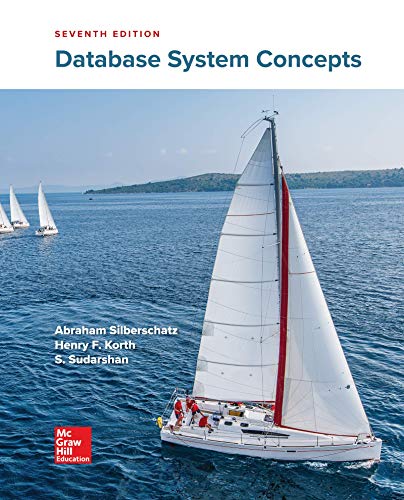
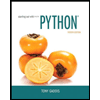
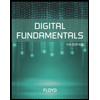
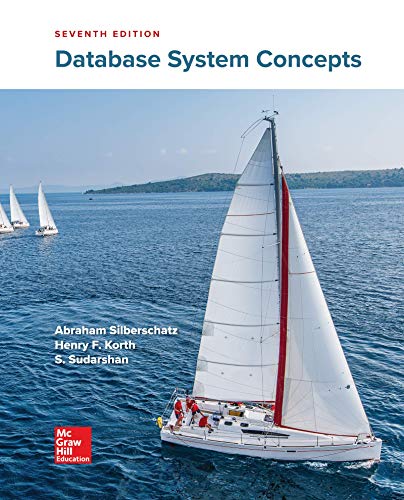
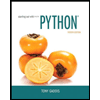
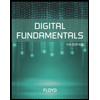
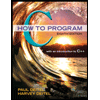
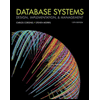
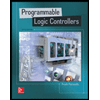