import java.util.Scanner; public class Main { public static final double OVERTIME_RATE = 1.5; public static final double HOLD = .1; public static final double TAX = .2; public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Name: "); String name = sc.nextLine(); System.out.print("SSN: "); String ssn
import java.util.Scanner;
public class Main
{
public static final double OVERTIME_RATE = 1.5;
public static final double HOLD = .1;
public static final double TAX = .2;
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.print("Name: ");
String name = sc.nextLine();
System.out.print("SSN: ");
String ssn = sc.nextLine();
int regularHours;
System.out.print("Regular Hours: ");
regularHours = sc.nextInt();
int overtimeHours;
System.out.print("Overtime Hours: ");
overtimeHours = sc.nextInt();
double hourlypayRate;
System.out.print("Hourly pay rate: $");
hourlypayRate = sc.nextDouble();
double regularPay = regularHours * hourlypayRate;
double overtimeRate = hourlypayRate * OVERTIME_RATE;
double overtimePay = overtimeHours * OVERTIME_RATE * hourlypayRate;
double gross = regularPay + overtimePay;
double wh = gross * HOLD;
double fTax = (gross - wh) * TAX;
double net = gross - wh - fTax;
System.out.println("___________________________________________________"+ "_________________");
String format = "Name: %-37s SSN: %-11s\n";
System.out.printf(format, name, ssn);
format = "Regular Hours: %-8d Reg Rate: $%-8.2f Reg Pay: $%-8.2f\n";
System.out.printf(format, regularHours, hourlypayRate, regularPay);
format = "Overtime Hours: %-8dOT Rate: $%-8.2f OT Pay: $%-8.2f\n";
System.out.printf(format, overtimeHours, overtimeRate, overtimePay);
format = "Gross Pay: $%-8.2f\n";
System.out.printf(format, gross);
format = "SS Withholding: $%-8.2f\n";
System.out.printf(format, wh);
format = "Federal Tax: $%-8.2f\n";
System.out.printf(format, fTax);
format = "Net Pay: $%-8.2f\n";
System.out.printf(format, net);
System.out.println("___________________________________________________"+ "_________________");
}
}
Using the above code create a class. The class Activity2PayStub will have four methods in it. Create the three new methods below the main method i.e., after the brace that closes main like so:
public static void main(String[] args)
{
... code from the above code is in here now ...
... Don't modify the main yet...
}
public void getInput(Scanner keyboard)
{
}
public void calculate()
{
}
public void printPayStub()
{
}

// Activity2PayStub.java
import java.util.Scanner;
public class Activity2PayStub{
// constants
public static final double OVERTIME_RATE = 1.5;
public static final double SS_WITHHOLDING = .1;
public static final double FEDERAL_TAX = .2;
// fields
private String name, ssn;
private int regHours, overHours;
private double hourlyRate, regPay, overRate, overPay, grossPay, ssWith, fedTax, netPay;
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
// create an object of Activity2PayStub class
Activity2PayStub a2ps = new Activity2PayStub();
// get inputs
a2ps.getInput(keyboard);
// compute payments
a2ps.calculate();
// display information
a2ps.printPayStub();
}
/**
* method that takes inputs of name, ssn, regular hours, overtime hours
* and hourly rate and set the fields to the values input by the user
*/
public void getInput(Scanner keyboard)
{
System.out.print("Enter your name: ");
name = keyboard.nextLine();
System.out.print("Enter your SSN: ");
ssn = keyboard.nextLine();
System.out.print("Enter your regular hours: ");
regHours = keyboard.nextInt();
System.out.print("Enter your overtime hours: ");
overHours = keyboard.nextInt();
System.out.print("Hourly pay rate: $");
hourlyRate = keyboard.nextDouble();
}
/**
* method to calculate the regular pay, overtime rate and pay, gross pay
* ssWitholding, federal tax and net pay
*/
public void calculate()
{
regPay = regHours * hourlyRate;
overRate = hourlyRate * OVERTIME_RATE;
overPay = overHours * OVERTIME_RATE * hourlyRate;
grossPay = regPay + overPay;
ssWith = grossPay * SS_WITHHOLDING;
fedTax = (grossPay - ssWith) * FEDERAL_TAX;
netPay = grossPay - ssWith - fedTax;
}
/**
* method to display the details input and calculated
*/
public void printPayStub()
{
System.out.println("___________________________________________________"
+ "_________________");
String format = "Name: %-37s SSN: %-11s\n";
System.out.printf(format, name, ssn);
format = "Regular Hours: %-8d Reg Rate: $%-8.2f Reg Pay: $%-8.2f\n";
System.out.printf(format, regHours, hourlyRate, regPay);
format = "Overtime Hours: %-7d OT Rate: $%-8.2f OT Pay: $%-8.2f\n";
System.out.printf(format, overHours, overRate, overPay);
format = "Gross Pay: $%-8.2f\n";
System.out.printf(format, grossPay);
format = "SS Withholding: $%-8.2f\n";
System.out.printf(format, ssWith);
format = "Federal Tax: $%-8.2f\n";
System.out.printf(format, fedTax);
format = "Net Pay: $%-8.2f\n";
System.out.printf(format, netPay);
System.out.println("___________________________________________________"
+ "_________________");
}
}
// end of Activity2PayStub.java
Step by step
Solved in 2 steps with 1 images

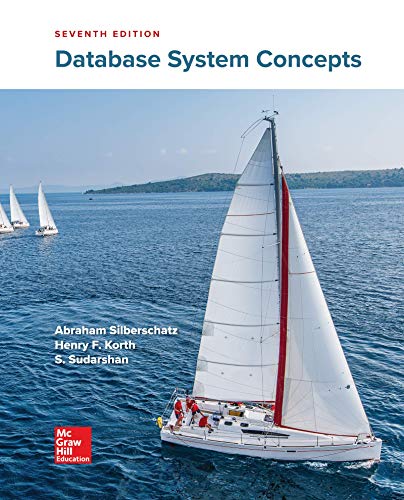
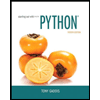
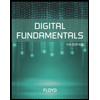
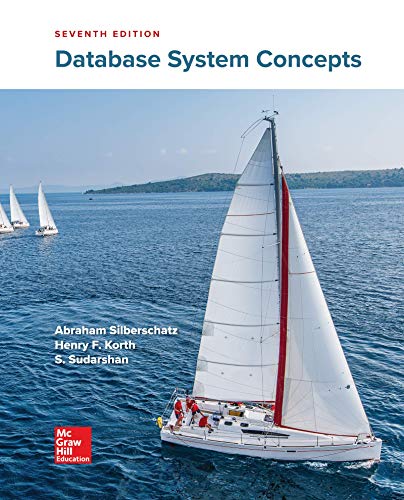
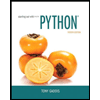
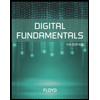
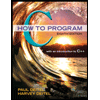
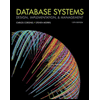
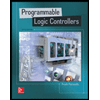