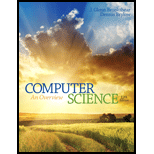
Computer Science: An Overview (12th Edition)
12th Edition
ISBN: 9780133760064
Author: Glenn Brookshear, Dennis Brylow
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 5.4, Problem 5QE
Program Plan Intro
“while” loop:
A while loop is a control flow statement whose basic purpose is to execute the block of statements repeatedly as long as the conditional statement is true.
- If the condition is false then it does not execute the block of statement.
Insertion sort
The algorithm is basically designed to organize and sort the array or list of elements in specific manner, that enables the system and user to get the final sorted array or a list of elements.
Generally, the insertion sort technique divides the list into two parts: sorted or unsorted list.
In the sorted list, the method assumes that one element of the list is already sorted and then it performs the operation on the unsorted list by inserting elements in its appropriate position in the sorted list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Downvote fir incorrect code and logic
Thank you
def eliminate_neighbours (items):
Given the sequence of integer items that are guaranteed to be some permutation of positive
integers from 1 to n where n is the length of the list, find the smallest number among those that still
remain in the list, and remove from the list both that number and whichever of its current
immediate neighbours is larger. The function should repeat this basic operation until the largest
number in the original list gets eliminated. Return the number of removal operations that were
needed to achieve this goal.
For example, given the list [5, 2, 1, 4, 6, 31, the operation would remove element 1 and its
current larger neighbour 4, resulting in the list [5, 2, 6, 3]. Applied again, that operation
would remove 2 and its current larger neighbour 6, thus reaching the goal in two steps.
items
Expected result
[1, 6, 4, 2, 5, 3]
1
[8, 3, 4, 1, 7, 2, 6, 5]
3
[8, 5, 3, 1, 7, 2, 6, 4]
4
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
5
range (1, 10001)
5000
[1000] + list(range (1, 1000))…
Q2: a. Write an algorithm that searches a sorted list of n items by dividing it into three sublists of almost n/3 items.
This algorithm finds the sublist that might contain the given item and divides it into three smaller sublists of
almost equal size. The algorithm repeats this process until it finds the item or concludes that the item is not in
the list. Dry run the above algorithm to find the value 240.
A[] = {10,15,20,60,65,110,150,220,240,245,260,290,300,460,470,501}
Chapter 5 Solutions
Computer Science: An Overview (12th Edition)
Ch. 5.1 - Prob. 1QECh. 5.1 - Prob. 2QECh. 5.1 - Prob. 3QECh. 5.1 - Suppose the insertion sort as presented in Figure...Ch. 5.2 - A primitive in one context might turn out to be a...Ch. 5.2 - Prob. 2QECh. 5.2 - The Euclidean algorithm finds the greatest common...Ch. 5.2 - Describe a collection of primitives that are used...Ch. 5.3 - Prob. 2QECh. 5.3 - Prob. 3QE
Ch. 5.3 - Prob. 4QECh. 5.4 - Modify the sequential search function in Figure...Ch. 5.4 - Prob. 2QECh. 5.4 - Some of the popular programming languages today...Ch. 5.4 - Suppose the insertion sort as presented in Figure...Ch. 5.4 - Prob. 5QECh. 5.4 - Prob. 6QECh. 5.4 - Prob. 7QECh. 5.5 - What names are interrogated by the binary search...Ch. 5.5 - Prob. 2QECh. 5.5 - What sequence of numbers would be printed by the...Ch. 5.5 - What is the termination condition in the recursive...Ch. 5.6 - Prob. 1QECh. 5.6 - Give an example of an algorithm in each of the...Ch. 5.6 - List the classes (n2), (log2n), (n), and (n3) in...Ch. 5.6 - Prob. 4QECh. 5.6 - Prob. 5QECh. 5.6 - Prob. 6QECh. 5.6 - Prob. 7QECh. 5.6 - Suppose that both a program and the hardware that...Ch. 5 - Prob. 1CRPCh. 5 - Prob. 2CRPCh. 5 - Prob. 3CRPCh. 5 - Select a subject with which you are familiar and...Ch. 5 - Does the following program represent an algorithm...Ch. 5 - Prob. 6CRPCh. 5 - Prob. 7CRPCh. 5 - Prob. 8CRPCh. 5 - What must be done to translate a posttest loop...Ch. 5 - Design an algorithm that when given an arrangement...Ch. 5 - Prob. 11CRPCh. 5 - Design an algorithm for determining the day of the...Ch. 5 - What is the difference between a formal...Ch. 5 - Prob. 14CRPCh. 5 - Prob. 15CRPCh. 5 - The following is a multiplication problem in...Ch. 5 - Prob. 17CRPCh. 5 - Four prospectors with only one lantern must walk...Ch. 5 - Starting with a large wine glass and a small wine...Ch. 5 - Two bees, named Romeo and Juliet, live in...Ch. 5 - What letters are interrogated by the binary search...Ch. 5 - The following algorithm is designed to print the...Ch. 5 - What sequence of numbers is printed by the...Ch. 5 - Prob. 24CRPCh. 5 - What letters are interrogated by the binary search...Ch. 5 - Prob. 26CRPCh. 5 - Identity the termination condition in each of the...Ch. 5 - Identity the body of the following loop structure...Ch. 5 - Prob. 29CRPCh. 5 - Design a recursive version of the Euclidean...Ch. 5 - Prob. 31CRPCh. 5 - Identify the important constituents of the control...Ch. 5 - Identify the termination condition in the...Ch. 5 - Call the function MysteryPrint (defined below)...Ch. 5 - Prob. 35CRPCh. 5 - Prob. 36CRPCh. 5 - Prob. 37CRPCh. 5 - The factorial of 0 is defined to be 1. The...Ch. 5 - a. Suppose you must sort a list of five names, and...Ch. 5 - The puzzle called the Towers of Hanoi consists of...Ch. 5 - Prob. 41CRPCh. 5 - Develop two algorithms, one based on a loop...Ch. 5 - Design an algorithm to find the square root of a...Ch. 5 - Prob. 44CRPCh. 5 - Prob. 45CRPCh. 5 - Design an algorithm that, given a list of five or...Ch. 5 - Prob. 47CRPCh. 5 - Prob. 48CRPCh. 5 - Prob. 49CRPCh. 5 - Prob. 50CRPCh. 5 - Prob. 51CRPCh. 5 - Does the loop in the following routine terminate?...Ch. 5 - Prob. 53CRPCh. 5 - Prob. 54CRPCh. 5 - The following program segment is designed to find...Ch. 5 - a. Identity the preconditions for the sequential...Ch. 5 - Prob. 57CRPCh. 5 - Prob. 1SICh. 5 - Prob. 2SICh. 5 - Prob. 3SICh. 5 - Prob. 4SICh. 5 - Prob. 5SICh. 5 - Is it ethical to design an algorithm for...Ch. 5 - Prob. 7SICh. 5 - Prob. 8SI
Knowledge Booster
Similar questions
- Sort the following list using the Selection Sort algorithm . Show the list after each iteration of the outer for loop (after each complete pass through the list) IMPORTANT: Separate each value by a comma and only one space after each comma and no space after the last value. You will have seven iterations of the list for your answer. 38, 60, 43, 5, 70, 58, 15, 10arrow_forwardin phython language Create a list that consists of all the numbers between 5 and 15. Use a for or while loop. (Create an empty list first, then append the numbers to the list in each iteration of a loop.) Insert the number -5 in index position 4 Delete the number at index 9 Append the number -7 to the list Sort the list Create another list that contains the first 5 elements of the first list, deleting this elements from the first list. Print the listsarrow_forward# This function takes a list of points and returns a new list of points, # beginning with the first point and then at each stage, moving to the closest point that has not yet been visited.# Note: the function should return a new list, not modify the existing list.# Hint: use a while loop to repeat while the list of remaining points is not emptyarrow_forward
- Fix the code below properly with no errors: Module.py #Defination to sort the list def sort(listNum): sortedList = [] #While loop will run until the listNum don't get null while(len(listNum) != 0 ): #Set the min as first element in list min = listNum[0] #iterate over the list to compare every element with num for ele in listNum: #If element is less than min if ele < min: #Then set min as element min = ele #append the sorted element in list sortedList.append(min) #Remove the sorted element from the list listNum.remove(min) return sortedList #Function to find the sum of all elements in list def SumOfList(listNum): #Set the sum as zero sum =0 #Iterate over the list to get every element for ele in listNum: #Keep adding the element to the sum variable sum = sum + ele #returnt the sum return sum…arrow_forwardTrue/False 9. The add method can be used to add an item to the end of a list.arrow_forwardSplit - Filter - Sort romeo = 'But soft what light through yonder window breaks It is the east and Juliet is the sun Arise fair sun and kill the envious moon Who is already sick and pale with grief Split the long string romeo into a list of words using the split function. For each word, check to see if the word is already in a list. If the word is not in the list, add it to the list. When the program completes, sort and print the resulting words in alphabetical order. The result will be like this: ['Arise', 'But', 'It', 'Juliet', "Who', 'already', 'and', 'breaks', 'east', 'envious', 'fair', 'grief', 'is', 'kill', "'ight', 'moon', 'pale', 'sick', 'soft', 'sun', 'the', 'through', 'what', 'window', 'with', 'yonder']arrow_forward
- python help Q5: Compose Write the procedure composed, which takes in procedures f and g and outputs a new procedure. This new procedure takes in a number x and outputs the result of calling f on g of x. (define (composed f g) 'YOUR-CODE-HERE ) Q6: Remove Implement a procedure remove that takes in a list and returns a new list with all instances of item removed from lst. You may assume the list will only consist of numbers and will not have nested lists. Hint: You might find the filter procedure useful. (define (remove item lst) 'YOUR-CODE-HERE ) ;;; Tests (remove 3 nil) ; expect () (remove 3 '(1 3 5)) ; expect (1 5) (remove 5 '(5 3 5 5 1 4 5 4)) ; expect (3 1 4 4)arrow_forwardList operations: Add something to the end of a list, remove something from the end of a list, add something/remove something from the middle of the list, get/set values by indexing into the list. How long does each of these operations take for each kind of list? Find the size of a list, check if it is empty Repeatedly remove the last value in a list until the list is empty Print out each element of the list Swap every pair of values in the list Which data structure is best for • random access • insertion/removal at a cursor? when a cursor needs to move forward and backward? • insertion/removal at the front? • insertion removal at the end?arrow_forwardPerform a selection sort on the list 7, 4, 2, 9, 6. Show the list after each exchange that has an effect on the list ordering.arrow_forward
- **Cenaage Python** Question: A sequential search of a sorted list can halt when the target is less than a given element in the list. Modify the program to stop when the target becomes less than the current value being compared. In a sorted list, this would indicate that the target is not in the list and searching the remaining values is unnecessary. CODE: def sequentialSearch(target, lyst): """Returns the position of the target item if found, or -1 otherwise. The lyst is assumed to be sorted in ascending order.""" position = 0 while position < len(lyst): if target == lyst[position]: return position position += 1 return -1 def main(): """Tests with three lists.""" print(sequentialSearch(3, [0, 1, 2, 3, 4])) print(sequentialSearch(3, [0, 1, 2])) # Should stop at second position. print(sequentialSearch(3, [0, 4, 5, 6])) if __name__ == "__main__": main()arrow_forwardThis chapter's bubble sort method is less efficient than it could be. If a pass through the list is done without swapping any components, the list is sorted and there is no reason to continue. Change this algorithm to terminate as soon as it recognises that the list is sorted. A break statement should not be used.This chapter's bubble sort method is less efficient than it could be. If a pass through the list is done without swapping any components, the list is sorted and there is no reason to continue. Change this algorithm to terminate as soon as it recognises that the list is sorted. A break statement should not be used.This chapter's bubble sort method is less efficient than it could be. If a pass through the list is done without swapping any components, the list is sorted and there is no reason to continue. Change this algorithm to terminate as soon as it recognises that the list is sorted. A break statement should not be used.This chapter's bubble sort method is less efficient…arrow_forwardWrite the following function that returns true if the list is already sorted in increasing order:def isSorted(lst):Write a test program that prompts the user to enter a list and displays whether the list is sorted or not.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
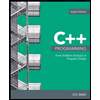
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
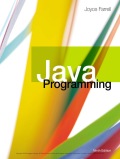
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT