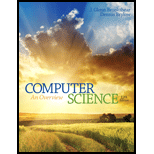
Explanation of Solution
Modified function for “MysteryWrite” function:
The modified function for “MysteryWrite” function is shown below:
#Function definition for "MysteryWrite"
def MysteryWrite(Last, Current):
#Check the value of variable "Current"
if(Current < 100):
#Add the value of "Current" and "Last" and store it variable "Temp"
Temp = Current + Last
#Call "MysteryWrite" function with arguments "Current" and "Temp"
MysteryWrite(Current, Temp)
#Display the numbers in reverse order
print(Current)
#Display the message
print('Reverse order')
#Call "MysteryWrite" function with the value for "Current" is "0" and "Last" is "1"
MysteryWrite(0,1)
Explanation:
The above code is used to print the sequence of numbers in reverse order using “MysteryWrite”

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Computer Science: An Overview (12th Edition)
- Perform in C#arrow_forwardWrite a function which takes two integer parameters for values to be added together and returns the result by value. The function may not print anything or read anything directly from the user (i.e. no cin/cout in the function). Assume that the values passed to the function will not be negative, but could be 0 or positive, and will both be integers. The function must implement addition recursively, and cannot use the standalone + operator (only ++) or call any other functions.arrow_forwardProvide Complete code , please don't spam itarrow_forward
- MULTIPLE FUNCTIONS AND RECURSIVE FUNCTIONS Use #include<stdio.h> Implement the picture shown below.arrow_forwardWrite a recursive function called draw_triangle() that outputs lines of '*' to form a right side up isosceles triangle. Function draw_triangle() has one parameter, an integer representing the base length of the triangle. Assume the base length is always odd and less than 20. Output 9 spaces before the first '*' on the first line for correct formatting. Hint: The number of '*' increases by 2 for every line drawn. Ex: If the input of the program is: 3 the function draw_triangle() outputs: * *** Ex: If the input of the program is: 19 the function draw_triangle() outputs: * *** ***** ******* ********* *********** ************* *************** ***************** ******************* Note: No space is output before the first '*' on the last line when the base length is 19. if __name__ == '__main__': base_length = int(input()) draw_triangle(base_length)arrow_forwardFibonacci numbers are a sequence of integers, starting with 1, where the value of each number is the sum of the two previous numbers, e.g. 1, 1, 2, 3, 5, 8, etc. Write a function called fibonacci that takes a parameter, n, which contains an integer value, and have it return the nth Fibonacci number. (There are two ways to do this: one with recursion, and one without.)arrow_forward
- Write a recursive function that displays a string reversely on the console using the following header: void reverseDisplay(const string& s) For example, reverseDisplay("abcd") displays dcba. Write a test program that prompts the user to enter a string and displays its reversal.arrow_forwardExercise 1: The number of combinations CR represents the number of subsets of cardi- nal p of a set of cardinal n. It is defined by C = 1 if p = 0 or if p = n, and by C = C+ C in the general case. An interesting property to nxC calculate the combinations is: C : Write the recursive function to solve this problem.arrow_forward‘Write a function nesting(), which takes an arbitrary number of parameters, and returns a list containing the arguments. Write another function unnesting(), which takes a list L as the parameter and retums a 1D list. Notice that, you should be able to use loops to solve thisproblem and should not use recursive functions (which we will not cover in this course). ‘Write assertions to test the two functions.For example nesting(1, nesting(2, 3, nesting(4, 5, [6])), [7, 8], 9)unnesting([1, [2, 3, [4, 5, [6]]], [7. 8], 9]) (1, [2, 3, [4, 5, [6]]], [7, 8], 9][1, 2,3, 4, 5, 6, 7, 8 9]arrow_forward
- Write recursive functions for the following equations:a. Harmonic number is defined by the equationb. Fibonacci numbers are defined by the formula:FN = FN-2 + FN-1, for N ≥ 2 with F0 = 0 and F1 = 1arrow_forwardCodeW X bFor fun X C Solved x b Answer + x https://codeworko... CodeWorkout X265: Recursion Programmlng Exercise: GCD The greatest common divisor (GCD) for a pair of numbers is the largest positive integer that divides both numbers without remainder. For function GCD , write the missing base case condition and action. This function will compute the greatest common divisor of x and y.You can assume that x and y are both positive integers and that x > y. Greatest common divisor is computed as follows: = x and GCD(x, y) = GCD(y, x % y). Examples: GCD (6, 4) -> 2 Your An swer: 1 public int GCD(int x, int y) { if > { 2. > 3. } else { 4. return GCD(y, x % y); 9. { 7. 1:09 AM 50°F Clear 1V 1. 12/4/2021 甲arrow_forwardWrite a recursive function that displays a string reversely on the console using the following header: def reverseDisplay(value):For example, reverseDisplay("abcd") displays dcba. Write a test programthat prompts the user to enter a string and displays its reversal.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
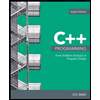