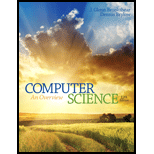
Computer Science: An Overview (12th Edition)
12th Edition
ISBN: 9780133760064
Author: Glenn Brookshear, Dennis Brylow
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 6CRP
Program Plan Intro
An algorithm is a step by step representation of any process in which the step should be executable.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).
I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ?
Cisco Packet Tracer
1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.
Chapter 5 Solutions
Computer Science: An Overview (12th Edition)
Ch. 5.1 - Prob. 1QECh. 5.1 - Prob. 2QECh. 5.1 - Prob. 3QECh. 5.1 - Suppose the insertion sort as presented in Figure...Ch. 5.2 - A primitive in one context might turn out to be a...Ch. 5.2 - Prob. 2QECh. 5.2 - The Euclidean algorithm finds the greatest common...Ch. 5.2 - Describe a collection of primitives that are used...Ch. 5.3 - Prob. 2QECh. 5.3 - Prob. 3QE
Ch. 5.3 - Prob. 4QECh. 5.4 - Modify the sequential search function in Figure...Ch. 5.4 - Prob. 2QECh. 5.4 - Some of the popular programming languages today...Ch. 5.4 - Suppose the insertion sort as presented in Figure...Ch. 5.4 - Prob. 5QECh. 5.4 - Prob. 6QECh. 5.4 - Prob. 7QECh. 5.5 - What names are interrogated by the binary search...Ch. 5.5 - Prob. 2QECh. 5.5 - What sequence of numbers would be printed by the...Ch. 5.5 - What is the termination condition in the recursive...Ch. 5.6 - Prob. 1QECh. 5.6 - Give an example of an algorithm in each of the...Ch. 5.6 - List the classes (n2), (log2n), (n), and (n3) in...Ch. 5.6 - Prob. 4QECh. 5.6 - Prob. 5QECh. 5.6 - Prob. 6QECh. 5.6 - Prob. 7QECh. 5.6 - Suppose that both a program and the hardware that...Ch. 5 - Prob. 1CRPCh. 5 - Prob. 2CRPCh. 5 - Prob. 3CRPCh. 5 - Select a subject with which you are familiar and...Ch. 5 - Does the following program represent an algorithm...Ch. 5 - Prob. 6CRPCh. 5 - Prob. 7CRPCh. 5 - Prob. 8CRPCh. 5 - What must be done to translate a posttest loop...Ch. 5 - Design an algorithm that when given an arrangement...Ch. 5 - Prob. 11CRPCh. 5 - Design an algorithm for determining the day of the...Ch. 5 - What is the difference between a formal...Ch. 5 - Prob. 14CRPCh. 5 - Prob. 15CRPCh. 5 - The following is a multiplication problem in...Ch. 5 - Prob. 17CRPCh. 5 - Four prospectors with only one lantern must walk...Ch. 5 - Starting with a large wine glass and a small wine...Ch. 5 - Two bees, named Romeo and Juliet, live in...Ch. 5 - What letters are interrogated by the binary search...Ch. 5 - The following algorithm is designed to print the...Ch. 5 - What sequence of numbers is printed by the...Ch. 5 - Prob. 24CRPCh. 5 - What letters are interrogated by the binary search...Ch. 5 - Prob. 26CRPCh. 5 - Identity the termination condition in each of the...Ch. 5 - Identity the body of the following loop structure...Ch. 5 - Prob. 29CRPCh. 5 - Design a recursive version of the Euclidean...Ch. 5 - Prob. 31CRPCh. 5 - Identify the important constituents of the control...Ch. 5 - Identify the termination condition in the...Ch. 5 - Call the function MysteryPrint (defined below)...Ch. 5 - Prob. 35CRPCh. 5 - Prob. 36CRPCh. 5 - Prob. 37CRPCh. 5 - The factorial of 0 is defined to be 1. The...Ch. 5 - a. Suppose you must sort a list of five names, and...Ch. 5 - The puzzle called the Towers of Hanoi consists of...Ch. 5 - Prob. 41CRPCh. 5 - Develop two algorithms, one based on a loop...Ch. 5 - Design an algorithm to find the square root of a...Ch. 5 - Prob. 44CRPCh. 5 - Prob. 45CRPCh. 5 - Design an algorithm that, given a list of five or...Ch. 5 - Prob. 47CRPCh. 5 - Prob. 48CRPCh. 5 - Prob. 49CRPCh. 5 - Prob. 50CRPCh. 5 - Prob. 51CRPCh. 5 - Does the loop in the following routine terminate?...Ch. 5 - Prob. 53CRPCh. 5 - Prob. 54CRPCh. 5 - The following program segment is designed to find...Ch. 5 - a. Identity the preconditions for the sequential...Ch. 5 - Prob. 57CRPCh. 5 - Prob. 1SICh. 5 - Prob. 2SICh. 5 - Prob. 3SICh. 5 - Prob. 4SICh. 5 - Prob. 5SICh. 5 - Is it ethical to design an algorithm for...Ch. 5 - Prob. 7SICh. 5 - Prob. 8SI
Knowledge Booster
Similar questions
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
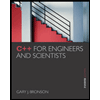
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
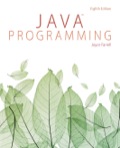
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
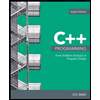
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
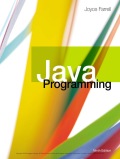
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
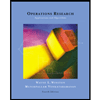
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole