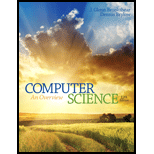
Computer Science: An Overview (12th Edition)
12th Edition
ISBN: 9780133760064
Author: Glenn Brookshear, Dennis Brylow
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 5, Problem 38CRP
The factorial of 0 is defined to be 1. The factorial of a positive integer is defined to be the product of that integer times the factorial of the next smaller nonnegative integer. We use the notation n! to express the factorial of the integer n. Thus the factorial of 3 (written 3!) is 3 × (2!) = 3 × (2 × (1!)) = 3 × (2 × (1 × (0!))) = 3 × (2 × (1 × (1))) = 6. Design a recursive
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Factorial of a number is defined as: n! = n(n-1)(n-2)(n-3)...(2)(1) For example, 4! = 4*3*2*1 The n! can be written in terms of (n-1)! as:
n! = n* (n-1)!
(n-1)! = (n-1)*(n-2)
! and so forth. Thus, in order to compute n!, we need (n-1)!, to have (n-1)!, we need (n-2)! and so forth. As you may immediately notice, the base case for factorial is 1 because 1! = 1. Write a program that uses a recursive function called factorial that takes an integer n as its argument and returns n! to the main.
C++ PLEASE
Given L = {w = {a, b}*: |w| is even}, the correct statements are:
(aa U ab Uba U bb)* is a regular expression that generates L.
(ab Uba)* is a regular expression that generates L.
aa U ab U ba U bb is a regular expression that generates L.
ab U ba is a regular expression that generates L.
The Fibonacci sequence is listed below: The first and second numbers both start at 1. After that, each number in the series is the sum of the two preceding numbers. Here is an example:
1, 1, 2, 3, 5, 8, 13, 21, ...
If F(n) is the nth value in the sequence, then this definition can be expressed as
F(1) = 1
F(2) = 1
F(3) = 2
F(4) = 3
F(5) = 5
F(6) = 8
F(7) = 13
F(8) = 21
F(n) = F(n - 1) + F(n - 2) for n > 2
Example:
Given n with a value of 4F(4) = F(4-1) + F(4-2)F(4) = F(3) + F(2)F(4) = 2 + 1F(4) = 3
The value of F at position n is defined using the value of F at two smaller positions. Using the definition of the Fibonacci sequence, determine the value of F(10) by using the formula and the sequence. Show the terms in the Fibonacci sequence and show your work for the formula.
Chapter 5 Solutions
Computer Science: An Overview (12th Edition)
Ch. 5.1 - Prob. 1QECh. 5.1 - Prob. 2QECh. 5.1 - Prob. 3QECh. 5.1 - Suppose the insertion sort as presented in Figure...Ch. 5.2 - A primitive in one context might turn out to be a...Ch. 5.2 - Prob. 2QECh. 5.2 - The Euclidean algorithm finds the greatest common...Ch. 5.2 - Describe a collection of primitives that are used...Ch. 5.3 - Prob. 2QECh. 5.3 - Prob. 3QE
Ch. 5.3 - Prob. 4QECh. 5.4 - Modify the sequential search function in Figure...Ch. 5.4 - Prob. 2QECh. 5.4 - Some of the popular programming languages today...Ch. 5.4 - Suppose the insertion sort as presented in Figure...Ch. 5.4 - Prob. 5QECh. 5.4 - Prob. 6QECh. 5.4 - Prob. 7QECh. 5.5 - What names are interrogated by the binary search...Ch. 5.5 - Prob. 2QECh. 5.5 - What sequence of numbers would be printed by the...Ch. 5.5 - What is the termination condition in the recursive...Ch. 5.6 - Prob. 1QECh. 5.6 - Give an example of an algorithm in each of the...Ch. 5.6 - List the classes (n2), (log2n), (n), and (n3) in...Ch. 5.6 - Prob. 4QECh. 5.6 - Prob. 5QECh. 5.6 - Prob. 6QECh. 5.6 - Prob. 7QECh. 5.6 - Suppose that both a program and the hardware that...Ch. 5 - Prob. 1CRPCh. 5 - Prob. 2CRPCh. 5 - Prob. 3CRPCh. 5 - Select a subject with which you are familiar and...Ch. 5 - Does the following program represent an algorithm...Ch. 5 - Prob. 6CRPCh. 5 - Prob. 7CRPCh. 5 - Prob. 8CRPCh. 5 - What must be done to translate a posttest loop...Ch. 5 - Design an algorithm that when given an arrangement...Ch. 5 - Prob. 11CRPCh. 5 - Design an algorithm for determining the day of the...Ch. 5 - What is the difference between a formal...Ch. 5 - Prob. 14CRPCh. 5 - Prob. 15CRPCh. 5 - The following is a multiplication problem in...Ch. 5 - Prob. 17CRPCh. 5 - Four prospectors with only one lantern must walk...Ch. 5 - Starting with a large wine glass and a small wine...Ch. 5 - Two bees, named Romeo and Juliet, live in...Ch. 5 - What letters are interrogated by the binary search...Ch. 5 - The following algorithm is designed to print the...Ch. 5 - What sequence of numbers is printed by the...Ch. 5 - Prob. 24CRPCh. 5 - What letters are interrogated by the binary search...Ch. 5 - Prob. 26CRPCh. 5 - Identity the termination condition in each of the...Ch. 5 - Identity the body of the following loop structure...Ch. 5 - Prob. 29CRPCh. 5 - Design a recursive version of the Euclidean...Ch. 5 - Prob. 31CRPCh. 5 - Identify the important constituents of the control...Ch. 5 - Identify the termination condition in the...Ch. 5 - Call the function MysteryPrint (defined below)...Ch. 5 - Prob. 35CRPCh. 5 - Prob. 36CRPCh. 5 - Prob. 37CRPCh. 5 - The factorial of 0 is defined to be 1. The...Ch. 5 - a. Suppose you must sort a list of five names, and...Ch. 5 - The puzzle called the Towers of Hanoi consists of...Ch. 5 - Prob. 41CRPCh. 5 - Develop two algorithms, one based on a loop...Ch. 5 - Design an algorithm to find the square root of a...Ch. 5 - Prob. 44CRPCh. 5 - Prob. 45CRPCh. 5 - Design an algorithm that, given a list of five or...Ch. 5 - Prob. 47CRPCh. 5 - Prob. 48CRPCh. 5 - Prob. 49CRPCh. 5 - Prob. 50CRPCh. 5 - Prob. 51CRPCh. 5 - Does the loop in the following routine terminate?...Ch. 5 - Prob. 53CRPCh. 5 - Prob. 54CRPCh. 5 - The following program segment is designed to find...Ch. 5 - a. Identity the preconditions for the sequential...Ch. 5 - Prob. 57CRPCh. 5 - Prob. 1SICh. 5 - Prob. 2SICh. 5 - Prob. 3SICh. 5 - Prob. 4SICh. 5 - Prob. 5SICh. 5 - Is it ethical to design an algorithm for...Ch. 5 - Prob. 7SICh. 5 - Prob. 8SI
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
When writing a program that performs an operation on a file, what two file associated names do you have to work...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
List the five major hardware components of a computer system.
Starting Out With Visual Basic (8th Edition)
The following statement subtracts 1 from x: x = x 1
Starting Out with Python (4th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Answer question 3.33, but do not consider any pet having the breed of Unknown.
Database Concepts (8th Edition)
For the circuit shown, find (a) the voltage υ, (b) the power delivered to the circuit by the current source, an...
Electric Circuits. (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Correct answer will be upvoted else Multiple Downvoted. Don't submit random answer. Computer science. Sasha likes exploring diverse mathematical articles, for instance, wizardry squares. However, Sasha comprehends that enchanted squares have as of now been examined by many individuals, so he sees no feeling of concentrating on them further. All things considered, he designed his own kind of square — a superb square. A square of size n×n is called prime if the accompanying three conditions are held all the while: all numbers on the square are non-negative integers not surpassing 105; there are no indivisible numbers in the square; amounts of integers in each line and every segment are indivisible numbers. Sasha has an integer n. He requests you to view as any great square from size n×n. Sasha is certain beyond a shadow of a doubt such squares exist, so help him! Input The principal line contains a solitary integer t (1≤t≤10) — the number of experiments. Every one…arrow_forwardCorrect answer will be upvoted else downvoted. Computer science. You are given an integer n. Check if n has an odd divisor, more noteworthy than one (does there exist such a number x (x>1) that n is separable by x and x is odd). For instance, assuming n=6, there is x=3. Assuming n=4, such a number doesn't exist. Input The primary line contains one integer t (1≤t≤104) — the number of experiments. Then, at that point, t experiments follow. Each experiment contains one integer n (2≤n≤1014). If it's not too much trouble, note, that the input for some experiments will not squeeze into 32-cycle integer type, so you should use no less than 64-digit integer type in your programming language. Output For each experiment, output on a different line: "Indeed" if n has an odd divisor, more noteworthy than one; "NO" in any case. You can output "YES" and "NO" regardless (for instance, the strings yEs, indeed, Yes and YES will be perceived as certain).arrow_forwardNOTE: The algorithm should be written in pseudo code, (explanation of the algorithm rather than code)arrow_forward
- Correct answer will be upvoted else Multiple Downvoted. Computer science. Polycarp has a most loved arrangement a[1… n] comprising of n integers. He worked it out on the whiteboard as follows: he composed the number a1 to the left side (toward the start of the whiteboard); he composed the number a2 to the right side (toward the finish of the whiteboard); then, at that point, as far to the left as could really be expected (yet to the right from a1), he composed the number a3; then, at that point, as far to the right as could be expected (however to the left from a2), he composed the number a4; Polycarp kept on going about too, until he worked out the whole succession on the whiteboard. The start of the outcome appears as though this (obviously, if n≥4). For instance, assuming n=7 and a=[3,1,4,1,5,9,2], Polycarp will compose a grouping on the whiteboard [3,4,5,2,9,1,1]. You saw the grouping composed on the whiteboard and presently you need to reestablish…arrow_forwardThe greatest common divisor of two positive integers, A and B, is the largest number that can be evenly divided into both of them. Euclid's algorithm can be used to find the greatest common divisor (GCD) of two positive integers. You can use this algorithm in the following manner: 1. Compute the remainder of dividing the larger number by the smaller number. 2. Replace the larger number with the smaller number and the smaller number with the remainder. 3. Repeat this process until the smaller number is zero. The larger number at this point is the GCD of A and B. Write a program that lets the user enter two integers and then prints each step in the process of using the Euclidean algorithm to find their GCD. An example of the program input and output is shown below: Enter the smaller number: 5 Enter the larger number: 15 The greatest common divisor is 5arrow_forwardGiven the following FSM M, the correct statements are: D 2 0 a 93 b a (a u ba)bb*a is a regular expression that generates L(M). (€ U b)a(bb*a)* is a regular expression that generates L(M). ba U ab*a is a regular expression that generate L(M). (a Uba)(bb*a)* is a regular expression that generate L(M).arrow_forward
- (b) The Fibonacci numbers for n 0, 1,...are defined as follows. fib (0)-0 fib (1) 1: fib (n) fib (n- 1) + fib (n - 2): Consider the following piece of code that calculates the Fibonacci numbers. int fib ( int n){ if (n is 0) return 0 else if (n is 1) return 1 else return fib (n- 1)+ fib (n- 2) What is the worst-case complexity of the above code?arrow_forwardThe “odd/even factorial” of a positive integer n is represented as n!! and is defined recursively as: a. (n)*(n-2)*(n-4)………*(2) if n is even. For example, 6!! = 6*4*2 = 48 b. (n)*(n-2)*(n-4)*…….*(5)*(3)*(1) if n is odd. For example, 7!! = 7*5*3*1 = 105. Come up with a recursive definition for n!! and use it to guide you to write a method definition for a method called “oddevenfact” that recursively calculates the odd/even factorial value of its single int parameter. The value returned by “oddevenfact” is a long.arrow_forwardIf n is an integer, what are the common divisors of n and 1? What are thecommon divisors of n and 0?arrow_forward
- HALP m + n = 2k + 2k = 4k therefore adding even numbers always gives an even number. The above is wrong because.... it doesn't consider the case when adding to odd numbers m and n are different so you cannot use 2k for both it doesn't consider what m and n are for particular values in the integers it doesn't consider the case when m is even and n is oddarrow_forwardBlackout Math is a math puzzle in which you are given an incorrect arithmetic equation. The goal of the puzzle is to remove two of the digits and/or operators in the equation so that the resulting equation is correct. For example, given the equation 6 - 5 = 15 ^ 4/2 we can remove the digit 5 and the / operator from the right-hand side in order to obtain the correct equality 6 - 5 = 1 ^ 42. Both sides of the equation now equal to 1. Observe how removing an operator between two numbers (4 and 2) causes the digits of the numbers to be concatenated (42). Here is a more complicated example: 288 / 24 x 6 = 18 x 13 x 8 We can remove digits and operators from either side of the equals sign (either both from one side, or one on each side). In this case, we can remove the 2 from the number 24 on the left-hand side and the 1 from the number 13 on the right-hand side to obtain the correct equality 288 / 4 x 6 = 18 x 3 x 8 Both sides of the equation now equal to 432. Here is another puzzle for you…arrow_forwardComputer Science You must count the number of strings of length 6 over the alphabet A = {x, y, z}. Any string of length 6 can be considered as a 6-tuple. For example, the string xyzy can be represented by the tuple (x,y,z,y). So the number of strings of length 6 over A equals the number of 6-tuples over A, which by product rule is N. Find N. (Use the following product rule for any finite set A and any natural number n : |A^n|=|A|^n)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
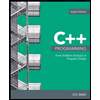
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
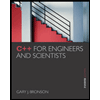
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7); Author: CS Dojo;https://www.youtube.com/watch?v=D6xkbGLQesk;License: Standard YouTube License, CC-BY