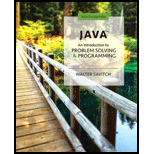
Explanation of Solution
a.
Method heading for each method:
- Method heading for project rating is “public void rateProject()”.
- Method heading for total rating is “public int totalRating()”...
Explanation of Solution
b.
Preconditions and postconditions of each method:
- Precondition and postcondition of “public void rateProject()” method.
- Precondition: None.
- Postcondition: Ratings were attained from user for each category and then assign it to the variable.
- Precondition and postcondition of “totalRating()” method.
- Precondition: None.
- Postcondition: This method returns total rating for each category...
Explanation of Solution
c.
Java statement for testing the class:
//Create an object for "ScienceFairProjectRating" class
ScienceFairProjectRating joesProject = new ScienceFairProjectRating();
ScienceFairProjectRating suesProject = new ScienceFairProjectRating();
//Call "initialize()" method to initialize the variables
joesProject.initialize("Weather and You", "U1256", "Joe Minnow");
suesProject...
Explanation of Solution
d.
Implementation of class:
ScienceFairProjectRating.java:
//Import the java package
import java.util.Scanner;
//Define the class
public class ScienceFairProjectRating
{
//Declare the required variables
private String projectName;
private String identifier;
private String submitter;
//Create an object for "RatingScore" class
private RatingScore creativeRating;
private RatingScore thoughtRating;
private RatingScore thoroughnessRating;
private RatingScore technicalRating;
private RatingScore clarityRating;
//Define the "initialize()" method
public void initialize(String name, String id, String person)
{
//Initialize the required variables
projectName = name;
identifier = id;
submitter = person;
creativeRating = new RatingScore();
thoughtRating = new RatingScore();
thoroughnessRating = new RatingScore();
technicalRating = new RatingScore();
clarityRating = new RatingScore();
creativeRating.initialize("How creative was this project", 30);
thoughtRating.initialize("Was scientific thought displayed in the project", 30);
thoroughnessRating.initialize("Did the project cover the material thoroughly", 15);
technicalRating.initialize("Were appropriate technical skill displayed", 15);
clarityRating.initialize("Was the presentation of the project clear", 10);
}
//Define the "rateProject()" method
public void rateProject()
{
//Display the message to get the rating
System.out.println("Please enter ratings for the project " + projectName + "(" + identifier + ")");
/*Call "inputRating()" method using objects of RatingScore class. */
creativeRating.inputRating();
thoughtRating.inputRating();
thoroughnessRating.inputRating();
technicalRating.inputRating();
clarityRating.inputRating();
}
//Define the "totalRating()" method
public int totalRating()
{
//Calculate the total rating and then return it
return creativeRating.getRating() + thoughtRating.getRating() + thoroughnessRating.getRating() + technicalRating.getRating() + clarityRating.getRating();
}
//Define the "maxRating()" method
public int maxRating()
{
//Calculate the maximum rating and then return it
return creativeRating.getMaxRating() + thoughtRating.getMaxRating() + thoroughnessRating.getMaxRating() + technicalRating.getMaxRating() + clarityRating.getMaxRating();
}
//Define the "getRatingString()" method
public String getRatingString()
{
//Calculate the project rating and then return it
return "Project " + projectName + "(" + identifier + ") by " + submitter + " was given the score "
+ totalRating() + "/" + maxRating();
}
//Define the "main()" method
public static void main(String[] args)
{
/*Create an object for "ScienceFairProjectRating" class. */
ScienceFairProjectRating joesProject = new ScienceFairProjectRating();
ScienceFairProjectRating suesProject = new ScienceFairProjectRating();
/*Call initialize() method to initialize the variables. */
joesProject.initialize("Weather and You", "U1256", "Joe Minnow");
suesProject.initialize("Hot Air", "U1275", "Susan Shark");
//Call "ratePorject()" to rate the project
joesProject.rateProject();
suesProject.rateProject();
System.out.println("Judging is completed: ");
/*Call "getRatingString()" method to get the project rating. */
System.out.println(joesProject.getRatingString());
System.out.println(suesProject.getRatingString());
}
}
RatingScore.java:
//Import the java package
import java.util.Scanner;
//Define the class
public class RatingScore
{
//Declare the required variables
private String description;
private int maximumRating;
private int theRating;
//Define the "initialize()" method
public void initialize(String desc, int max)
{
//Initialize the required variables
description = desc;
maximumRating = max;
theRating = -1;
}
//Define "inputRating()" method
public void inputRating()
{
//Read the maximum rating value from user
System...

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Can the answer be in more classes then one where the classes will have relationships with other classes through the personarrow_forwardWrite classes that could be used in a system that manages vehicles in a public transportation system. You will write classes to represent a TransitVehicle, Bus, and SubwayCar. The information about the classes is below. Step 1: Plan Your Class Design Before you start to code, decide how to design your classes. Which class should be the parent? the child? What variables or methods belong in each class? Step 2: Write the Core Class Components Write the three classes. Each class must have all of the following. Important: a class can have a method directly (meaning written into the code) or indirectly (meaning inherited from a parent). Either of these counts to meet the requirement. instance data variables transit vehicles are described by an id that contains letters and numbers and a description of the route buses are described by an id, route, and the number of miles per gallon (without decimals) subway cars are described by an id, route, and whether or not the car goes underground…arrow_forwardDesign and implement a class called Bug, which represents a bug moving along a horizontal wire. The bug can only move for one unit of distance at a time, in the direction it is facing. The bug can also turn to reverse direction. For your design, create a UML Class diagram . Note that you need to include the constructor in the methods section if you code a constructor. Bug will require a toString method to return the current position and which direction the bug is facing to the driver so it can be output Write an interactive test driver that instantiates a Bug, then allows the user to manipulate it with simple commands like Output (to see the position and direction), Move, Turn, Exit ... single letters work just fine. All output should be via the driver not methods within Bug. You should use this driver to create screenshot exhibits for a number of scenarios (e.g., output original position, move a few times, output, move a few more times, output, turn, output, move, output, etc.).…arrow_forward
- A company plans to create a system for managing of a sound recording company. The sound recording company has a name, address, owner and performers. Each performer has name, nickname and created albums. Albums are described with name, genre, year of creation, number of sold copies and list of songs. The songs are described with name and duration. Design a set of classes with relationships between each other, which models the data of the record company. Implement a test class, which demonstrates the work of rest of the classes. c sharparrow_forwardDesign and implement a set of classes that keep track of varioussports statistics. Have each low-level class represent a specificsport. Tailor the services of the classes to the sport in question,and move common attributes to the higher-level classes as appropriate. Create a driver class to instantiate and exercise several ofthe classesarrow_forwardA company plans to create a system for managing of a movie store. The movie store has a name, address and phone number. Movies are described with title , genre, year of creation, number of sold copies and list of songs. The songs are described with name and duration. Design a set of classes and relationship between each other, which models the data of the record company. Implement a test class, which demonstrates the work of rest of the classesarrow_forward
- Design a system to simulate a social media platform, such as Twitter or Facebook. The system should include classes to represent users, posts, comments, and likes. The User class should have attributes for the user's name, username, password, email, and a list of their posts. Users should be able to follow and unfollow other users and view their posts on their timelines. Users should also be able to send and receive private messages. The Post class should have attributes for the post's author, content, date and time, and a list of comments and likes. Users should be able to create new posts, delete their own posts, and comment on and like other users' posts. The Comment class should have attributes for the comment's author, content, date and time, and the post it belongs to. Users should be able to add and delete comments on a post. The Like class should have attributes for the user who liked the post and the post that was liked. Users should be able to like and unlike posts. To make…arrow_forwardGiven a base class with the following UML class diagram. Create a Person base class for the following. You have to create a NetBeans project for this question. Person - name: String + setName(String newname): void + getName( ): String + writeOutput ( ): void + equals (Person otherPerson): boolean You have to use the base class Person you have created to derive a class named as Student that can be used for the objects for students in a university. The employee record will inherit a student's name from the class person. A student record will contain the GPA score represented as a single value of type double, a starting date that gives the year that student started the course as a single value of type int, and a student identification number that is a value of type long. You have to provide your solution with the following guidelines. • Provide the Person Base Class (2 marks) • You have to provide the derived Student class from Person class and take care of the following. • Provide…arrow_forwardIn a university there are different classrooms, offices and departments. A department has a name and it contains many offices. A person working at the university has a unique ID and can be a professor or an employee. "A professor can be a full, associate or assistant professor and he/she is enrolled in one department. •Offices and classrooms have a number ID, and a classroom has a number of seats. • Every employee works in an office. 1. Draw the class diagram for the scenario abovearrow_forward
- Consider the class Movie that contains information about a movie. The class has the following attributes: . The movie name • The SA Film and Publication Board (FPB) rating (for example, A, PG, 7-9 PG, 10-12 PG, 13, 16, 18, X18, XX) 1 • The number of people that have rated this movie as 1 (Terrible) • The number of people that have rated this movie as 2 (Bad) • The • The • The number of people that have rated this movie as 3 (OK) number of number of people that have rated this movie as 4 (Good) people that have rated this movie as 5 (Great) The class Movie you created, can be implemented more efficiently if we use an array instead of 5 different member variables to count the number of people rating a movie at a specific score. Adjust your class Movie to use an array to count the score ratings for a movie, and test it with the same main function.arrow_forwardThis is the question: Design and implement a class called Bug, which represents a bug moving along a horizontal wire. The bug can only move for one unit of distance at a time, in the direction it is facing. The bug can also turn to reverse direction. For your design, create a UML Class diagram similar to Figure 5.5 on page 180 of the textbook. Note that you need to include the constructor in the methods section if you code a constructor. Bug will require a toString method to return the current position and which direction the bug is facing to the driver so it can be output.Hint: Remember that a horizontal line has a zero position in the middle with positive to the right and negative to theleft. Consider that a bug will land on the wire at some point before starting along the wire.Write an interactive test driver that instantiates a Bug, then allows the user to manipulate it with simple commands like Output (to see the position and direction), Move, Turn, Exit ... single letters work…arrow_forwardPlease prepare the case diagram and the class diagramarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
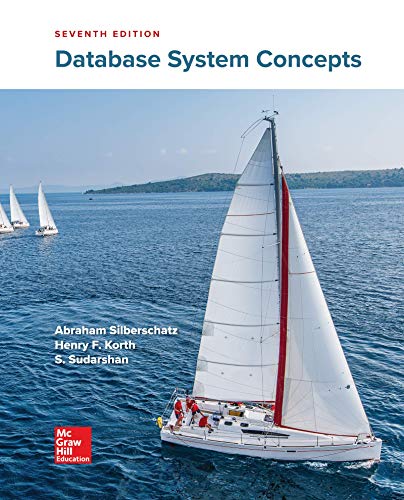
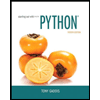
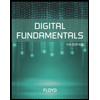
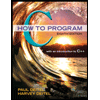
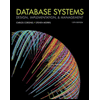
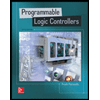