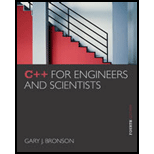
(General math) a. Write, compile, and run a C++
b. Check the result of your program written for Exercise 7a by using the following test data:
After finishing your verification, use your program to complete the following chart
a.

Program plan:
- The variable a, b and c of type float is used to accept the coefficient of second order polynomial.
- The variable x of type float is used to accept the value of x of second order polynomial.
- The variable p of type float calculates the value of second order polynomial.
Program description:
The main purpose of the program is to accept the coefficient of x and value of x and then calculate and display the value of second order polynomial.
Explanation of Solution
Program
#include <iostream> #include <math.h> using namespace std; int main() { // declaration of variables float a, b, c; float x; float p; // Input values cout<<"Input values to calculate value of the second-order polynomial :: ax^2 + bx +c"; cout<<"\nEnter the coefficient of the x-squared term(a) :: "; cin>>a; cout<<"\nEnter the coefficient of the x term (b) :: "; cin>>b; cout<<"\nEnter the coefficient of the term (c) :: "; cin>>c; cout<<"\nEnter the value of x :: "; cin>>x; // Calculate value of the second-order polynomial p = a * x * x + b * x + c ; cout<<"\nThe value of the second-order polynomial is :: "<<p; return 0; }
Explanation:
In the above program a, b and c accepts the coefficient of x for second order polynomial. The variable x accepts the value of x and then second order polynomial is calculated and displayed.
Sample Output:
Enterthecoefficientofthex-squaredterm(a)::0
Enterthecoefficientofthexterm(b)::0
Enterthecoefficientoftheterm(c)::22
Enterthevalueofx::56
Thevalueofthesecond-orderpolynomialis::22
b.

To check the given program for the test values as follows:
Test data set 1: a = 0, b = 0, c = 22, x = 56
Test data set 2: a = 0, b = 22, c = 0, x = 2
Test data set 3: a = 22, b = 0, c = 0, x = 2
Test data set 4: a = 2, b = 4, c = 5, x = 2
To complete the following chart:
a | b | c | x | Polynomial Value |
2.0 | 17.0 | -12.0 | 1.3 | |
3.2 | 2.0 | 15.0 | 2.5 | |
3.2 | 2.0 | 15.0 | -2.5 | |
-2.0 | 10.0 | 0.0 | 2.0 | |
-2.0 | 10.0 | 0.0 | 4.0 | |
-2.0 | 10.0 | 0.0 | 5.0 | |
-2.0 | 10.0 | 0.0 | 6.0 | |
5.0 | 22.0 | 18.0 | 8.3 | |
4.2 | -16.0 | -20.0 | -5.2 |
Explanation of Solution
Given Program
#include <iostream> #include <math.h> using namespace std; int main() { // declaration of variables float a, b, c; float x; float p; // Input values cout<<"Input values to calculate value of the second-order polynomial :: ax^2 + bx +c"; cout<<"\nEnter the coefficient of the x-squared term(a) :: "; cin>>a; cout<<"\nEnter the coefficient of the x term (b) :: "; cin>>b; cout<<"\nEnter the coefficient of the term (c) :: "; cin>>c; cout<<"\nEnter the value of x :: "; cin>>x; // Calculate value of the second-order polynomial p = a * x * x + b * x + c ; cout<<"\nThe value of the second-order polynomial is :: "<<p; return 0; }
Explanation:
Test data set 1:
a = 0, b = 0, c = 22, x = 56
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enterthecoefficientofthex-squaredterm(a)::0
Enterthecoefficientofthexterm(b)::0
Enterthecoefficientoftheterm(c)::22
Enterthevalueofx::56
Thevalueofthesecond-orderpolynomialis:: 22
Test data set 2:
a = 0, b = 22, c = 0, x = 2
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 0
Enter the coefficient of the x term (b) :: 22
Enter the coefficient of the term (c) :: 0
Enter the value of x :: 2
The value of the second-order polynomial is :: 44
Test data set 3:
a = 22, b = 0, c = 0, x = 2
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 22
Enter the coefficient of the x term (b) :: 0
Enter the coefficient of the term (c) :: 0
Enter the value of x :: 2
The value of the second-order polynomial is :: 88
Test data set 4:
a = 2, b = 4, c = 5, x = 2
Sample Output
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 2
Enter the coefficient of the x term (b) :: 4
Enter the coefficient of the term (c) :: 5
Enter the value of x :: 2
The value of the second-order polynomial is :: 21
Chart
Following chart displays the polynomial value for different values of a, b, c and x.
a | b | c | x | Polynomial Value |
2.0 | 17.0 | -12.0 | 1.3 | 13.480 |
3.2 | 2.0 | 15.0 | 2.5 | 40.000 |
3.2 | 2.0 | 15.0 | -2.5 | 30.000 |
-2.0 | 10.0 | 0.0 | 2.0 | 12.000 |
-2.0 | 10.0 | 0.0 | 4.0 | 8.000 |
-2.0 | 10.0 | 0.0 | 5.0 | 0.000 |
-2.0 | 10.0 | 0.0 | 6.0 | -12.000 |
5.0 | 22.0 | 18.0 | 8.3 | 545.050 |
4.2 | -16.0 | -20.0 | -5.2 | 176.768 |
Want to see more full solutions like this?
Chapter 3 Solutions
C++ for Engineers and Scientists
- Need help, has to be done with <stdio.h> and <math.h>arrow_forward(C++ OOP) We have a house with 3 rooms.We need the user to enter 1 integer as the window number, 2 doubles as depth and width of the room, 1 double as the useless area, 1 boolean as the door's opening direction (e.g. 0 is outward).Program will compute and print the air condition as a string and the total useful area of the house as a double. Calculating the air condition: Name it air_c. Divide the useful area by the window number. If air_c >20, output "bad". If air_c <11 output "good". If 11<=air_c<=20, output "ideal". Attention: If the window number is 0, air condition is bad. Calculating the useful area of room: Width*Depth-(useless area)if the door is inward;Width*Depth-(useless area)-0.8The worst air condition of the 3 is the final air condition of house. --- 1)The information about the home must be all private within a class.2)The class must get these inputs within constructor func.3)Calculating the useful area of a room must be done within a function of a class.4)Air…arrow_forward(c++) Write a program that takes user input describing a playing card with the following shorthand: A = Ace 2...10 card values J = Jack Q = Queen, etc. D = Diamonds S = Spades. etc. Ex. if the user enters QS then your program responds with Queen of Spadesarrow_forward
- (C PROGRAMMING ONLY) 1. Dealing With Monthsby CodeChum Admin We're done dealing with days. It's time to step up and now deal with months! Instructions: In the code editor, you are provided with a code in the main() which has 12 printf's. Each printf prints a month with its corresponding value. For this program, January is 1, February is 2, March is 3, and so on. When you run the initial code, you will encounter errors because these month names that have been printed don't exist yet.Your task is to create an enum data type which contains these month names as values so that when we run the code, there will be no more error.Do not edit anything in the main(). Output January = 1February = 2March = 3April = 4May = 5June = 6July = 7August = 8September = 9October = 10November = 11December = 12arrow_forward12. (Data processing) Write, run, and verify a C++ program that accepts three numbers as input, and then sorts the three numbers and displays them in ascending order, from lowest to highest. For example, if the input values are 7 5 1, the program should display them in the numerical macorder 1 5 7.arrow_forward(C++)Write a program that will allow a student to compute for his equivalent semestral grade. The program should ask the user should input his/her first name, last name, middle initial, and student number. After, inputting everything above, the output will clear the current screen and a new screen should open. A sample program that would clear a screen is included below for your reference. On the new screen, the user will then be greeted "Welcome, <firstname>! (i.e. Welcome, Louie!)" The program will then ask for the prelim grade, midterm grade and final grade of the student. The program should only accept grades 100 and below. After getting the three grades, the program should compute for the semestral grade. The computation of the semestral grade is as follows: 30%*PrelimGrade + 30%*midtermgrade + 40%finalgrade After inputting all the grades, the screen should clear again and will proceed to another screen. The new screen should output should output the following (this is…arrow_forward
- (c program only) 2. Jack `N Poyby CodeChum Admin Jack `N Poy is a very common game since our childhood days. Just thinking about it makes me reminisce how I crushed my cousin in this game until he cried ? I want to feel the glory again of being very good at something. Could you help me recreate the game? It's really simple. In Jack `N Poy, there are two players who both select either one of the following options: RockPaperScissors The winner is selected depending on the following rules: Rock beats ScissorsScissors beats PaperPaper beats RockIf both players chose the same option, then it's a tie Instructions: In the code editor, you are provided with an enum called option which contains three possible named values:ROCK - 'r'PAPER - 'p'SCISSORS - 's'Your task is to ask two users for there chosen options. And then based on their options, determine the winner.Input 1. Option selected by Player 1 2. Option selected by Player 2 Output If Player 1 wins, print the message "Player 1…arrow_forward(MIPS/MARS programming) Write a Program that initialize 4 by 4 Table and find the Maximum number in the table and prints the value, the row number and the column number of that maximum number.arrow_forward(software quality engineering)Derive the test cases using boundary value analysisarrow_forward
- (QUESTION) Using the required programming language (python, matlab, etc.) plot the variation of pressure on the piston surface as a function of time until the piston moves 9 m, with the help of the following commands below. This problem will be solved for U_p =1, 4, 16, 64, 256 m/s. We'll assume that the time starts at t = 0 when the piston starts moving. (PLEASE TAKE A SCREENSHOT OF THE PLOTTING AND OTHER RESULTS.) Note: Since we don't have the exact time intervals or the rate at which the piston moves, we'll assume a constant speed and divide the distance by the speed to get the time taken. (QUESTION) COMMANDS import matplotlib.pyplot as plt import numpy as np # Given parameters diameter = 0.1 # meters length = 10 # meters initial_pressure = 10e3 # Pascals initial_temperature = 288 # Kelvin # Convert diameter to radius radius = diameter / 2 # Calculate initial and final volumes initial_volume = np.pi * radius**2 * length final_volume = np.pi * radius**2 * (length - 9) # Calculate…arrow_forward(c++) Ask the user to provide a single alphabetic character. Print Vowel or Consonant depending on the input. If the u not a letter (between a and z or A and Z) or is a string of length > 1, print an error.arrow_forward(Heat transfer) The energy radiated from the surface of the sun or a planet in the solar system can be calculated by using Stefan-Boltzmann's Law: E = o x T4 E is the energy radiated. o is Stefan-Boltzmann's constant (5.6697 x 10-8 watts/m2K4). Tis the surface temperature in degrees Kelvin (°K = °C + 273). a. Determine the units of E by calculating the units resulting from the right side of the formula. b. Determine the energy radiated from the sun's surface, given that the sun's average temperature is approximately 6000°K.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
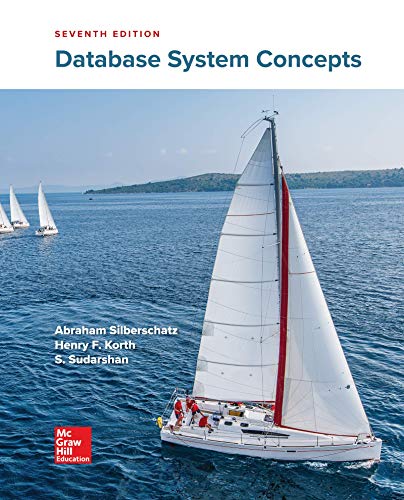
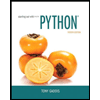
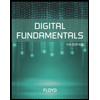
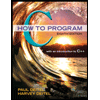
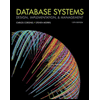
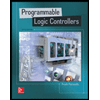