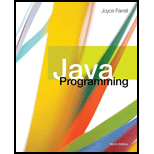
Explanation of Solution
a.
Program code:
NumbersDemo.java
//define the method NumbersDemo
public class NumbersDemo
{
//define the main() method
public static void main(String args[])
{
//create two integer variable
int n1 = 10;
int n2 = 7;
//call the static methods
displayTwiceTheNumber(n1);
displayNumberPlusFive(n1);
displayNumberSquared(n1);
//print new line
System.out.println();
//call the static methods
displayTwiceTheNumber(n2);
displayNumberPlusFive(n2);
displayNumberSquared(n2);
}
//define the method displayTwiceTheNumber()
public static void displayTwiceTheNumber(int n)
{
//multiply n by 2 and print it
System.out.println(2 * n);
}
//define the method displayNumberPlusFive()
public static void displayNumberPlusFive(int n)
{
//add 5 to n and dispaly it
System...
Explanation of Solution
b.
Program code:
NumbersDemo2.java
//import the package
import java.util.Scanner;
//define the method NumbersDemo
public class NumbersDemo2
{
//define the main() method
public static void main(String args[])
{
//create the object of scanner
Scanner in = new Scanner(System.in);
//prompt the user to enter two numbers
System.out.println("Enter the numbers");
//scan for the values
int n1 = in.nextInt();
int n2 = in.nextInt();
//print new line
System.out.println();
//call the static methods
displayTwiceTheNumber(n1);
displayNumberPlusFive(n1);
displayNumberSquared(n1);
//print new line
System.out.println();
//call the static methods
displayTwiceTheNumber(n2);
displayNumberPlusFive(n2);
displayNumberSquared(n2);
}
//define the method displayTwiceTheNumber()
public static void displayTwiceTheNumber(int n)
{
//multiply n by 2 and print it
System...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming (MindTap Course List)
- Please use Java as programming language Create a class whose main() holds two integer variables. Assign values to the variables. Create two methods named sum() and difference, that compute the sum and difference between the two variables respectively. Each method should perform the computation and display the results. In turn, call the two methods passing the values of the 2 variables. Create another method named product. The method should compute the product of the 2 numbers but will not display the answer. Instead, the method should return the answer to the calling main() which displays the answer. Provide your own screen display and class name.arrow_forwardThere are 12 inches in a foot and 3 feet in a yard. Create a class named InchConversion. Its main() method accepts a value in inches from a user at the keyboard, and in turn passes the entered value to two methods. One converts the value from inches to feet, and the other converts the same value from inches to yards. Each method displays the results with appropriate explanation. Save the application as InchConversion.java.arrow_forwardCreating Enumerations In this section, you create two enumerations that hold colors and car model types. You will use them as field types in a Car class and write a demonstration program that shows how the enumerations are used. 1. Open a new file in your text editor, and type the following Color enumeration: enum Color {BLACK, BLUE, GREEN, RED, WHITE, YELLOW}; 2. Save the file as Color.java. 3. Open a new file in your text editor, and create the following Model enumeration: enum Model {SEDAN, CONVERTIBLE, MINIVAN}; 4. Save the file as Model.java. Next, open a new file in your text editor, and start to define a Car class that holds three fields: a year, a model, and a color. public class Car { private int year; private Model model; private Color color; 5. Add a constructor for the Car class that accepts parameters that hold the values for year, model, and color as follows: public Car(int yr, Model m, Color c) { year = yr; model = m; color = c; } 6. Add a display()…arrow_forward
- This assigment wants me to: Assume that a gallon of paint covers about 350 square feet of wall space. Create an application with a main() method that prompts the user for the length, width, and height of a rectangular room. Pass these three values to a method that does the following: Calculates the wall area for a room Passes the calculated wall area to another method that calculates and returns the number of gallons of paint needed Displays the number of gallons needed Computes the price based on a paint price of $32 per gallon, assuming that the painter can buy any fraction of a gallon of paint at the same price as a whole gallon Returns the price to the main() method The main() method displays the final price. For example: You will need 2.0 gallons The price to paint the room is $64.0 here is my code and this is all i have so far help please ! import java.util.Scanner; public class PaintCalculator { public static void main (String args[]) { int AreaPerGalllon =…arrow_forward- Declare and create the following two Recipe objects then invoke the printRecipe method to print their info to the console: 1) Recipe name: Beef-Stuffed Peppers Preparation time: 55 minutes Servings: 4 Ingredients: 1 tablespoon olive oil 1 pound lean ground beef 1% cups water 1 (6 ounce) can tomato paste 4 green bell peppers, tops and seeds removed 2) Lemonade Preparation time: 15 minutes Servings: 10 Ingredients: Sugar Water Lemon juice Icearrow_forwardHi, I need help with Java code. Please see attached screenshot. Thank you!arrow_forward
- USE JAVA IDE Create a class that has three methods. One method is to input three numbers. The second method will return the largest number between the three numbers. The third method will return the smallest number between the three numbers. Since the largest and smallest number are both returned values, display these values in the main method.arrow_forwarda - Create a FitnessTracker class that includes data fields for a fitness activity, the number of minutes spent participating, and the date. The class includes methods to get each field. In addition, create a default constructor that automatically sets the activity to running, the minutes to 0, and the date to January 1 of the current year. Save the file as FitnessTracker.java. Create an application that demonstrates each method works correctly, and save it as TestFitnessTracker.java. b - Create an additional overloaded constructor for the FitnessTracker class you created in Exercise 3a. This constructor receives parameters for each of the data fields and assigns them appropriately. Add any needed statements to the TestFitnessTracker application to ensure that the overloaded constructor works correctly, save it, and then test it. c - Modify the FitnessTracker class so that the default constructor calls the three-parameter constructor. Save the class as FitnessTracker2.java. Create an…arrow_forwardPlease use Java as programming language Create a class named Commission that includes 3 variables: a double sales figure, a double commission rate and an integer commission rate. Create 2 overloaded methods named computeCommission(). The first method takes 2 double arguments representing sales and rate, multiplies them and then displays the results. The second method takes 2 arguments: a double sales figure and an integer commission rate. This method must divide the commission rate by 100.0 before multiplying by the sales figure and displaying the commission. Supply appropriate values for the variables. Test each overloaded methods.arrow_forward
- Modify the Percentages application whose main() method holds two double variables. Assign values to the variables. Pass both variables to a method named computePercent() that displays the two values and the value of the first number as a percentage of the second one. For example, if the numbers are 2.0 and 5.0, the method should display a statement similar to “2.0 is 40.0 percent of 5.0.” Then call the method a second time, passing the values in reverse order. For testing use the values 2.0 and 5.0. An example of the program is shown below: 2.0 is 40.0 percent of 5.0 5.0 is 250.0 percent of 2.0arrow_forwardModify the Percentages application whose main() method holds two double variables. Assign values to the variables. Pass both variables to a method named computePercent() that displays the two values and the value of the first number as a percentage of the second one. For example, if the numbers are 2.0 and 5.0, the method should display a statement similar to “2.0 is 40.0 percent of 5.0.” Then call the method a second time, passing the values in reverse order. For testing use the values 2.0 and 5.0. An example of the program is shown below: 2.0 is 40.0 percent of 5.0 5.0 is 250.0 percent of 2.0arrow_forwardVariables name: Choose meaningful and descriptive names. No x, y … etc. Use lowercase. If the name consists of several words, concatenate all in one, uselowercase for the first word, and capitalize the first letter of each subsequent word inthe name. For example, the variables radius and area, and the method computeArea.3. File type: only JAVA file is allowed. Name it as Assignment2. Java5. Please add the appropriate comments for each step you add. In the top of the program, addyour name, time of starting writing the code and title of the assignment. Extra informationwould be greatarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
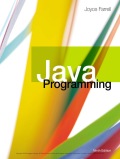
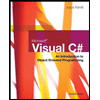
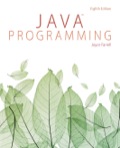