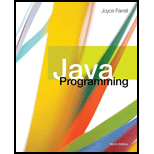
Explanation of Solution
a.
Program code:
Lease.java
//define the class Lease
public class Lease
{
//declare class members
private static final double PET_FEE = 10;
private String tenantName;
private int apartmentNumber;
private double monthlyRent;
private int leasePeriod;
//Default constructor
public Lease()
{
//initialize the class members
tenantName ="XXX";
apartmentNumber =0;
monthlyRent = 1000;
leasePeriod =12;
}
//getters and setters
//define a method getTenantName()
public String getTenantName()
{
//return the variable tenantName
return tenantName;
}
//define a method setTenantName()
public void setTenantName(String tenantName)
{
//set the value of tenantName
this.tenantName = tenantName;
}
//define a method getApartmentNumber()
public int getApartmentNumber()
{
//return the variable apartmentNumber
return apartmentNumber;
}
//define a method setApartmentNumber()
public void setApartmentNumber(int apartmentNumber)
{
//set the value of apartmentNumber
this.apartmentNumber = apartmentNumber;
}
//define a method getMonthlyRent()
public double getMonthlyRent()
{
//return the variable monthlyRent
return monthlyRent;
}
//define a method setMonthlyRent()
public void setMonthlyRent(double monthlyRent)
{
//set the value of monthlyRent
this.monthlyRent = monthlyRent;
}
//define a method getLeasePeriod()
public int getLeasePeriod()
{
//return the variable leasePeriod
return leasePeriod;
}
//define a method setLeasePeriod()
public void setLeasePeriod(int leasePeriod)
{
//set the value of leasePeriod
this.leasePeriod = leasePeriod;
}
//define a method addPetFee()
public void addPetFee()
{
//adds $10
monthlyRent+=PET_FEE;
}
//define a method explainPetPolicy()
public static void explainPetPolicy()
{
//print the statement
System.out.println("Add $10 to rent as pet fee.");
}
}
Explanation:
The above snippet of code is used create a class “Lease”. The class contain different static methods for store the details of a lease. In the code,
- Define a class “Lease”
- Declare the class members.
- Define the constructor “Lease()” method.
- Initialize the class members.
- Define the “getTenantName()” method.
- Return the value of the variable “tenantName”.
- Define the “setTenantName()” method.
- Set the value of the variable “tenantName”.
- Define the “getApartmentNumber()” method.
- Return the value of the variable “apartmentNumber”.
- Define the “setApartmentNumber()” method.
- Set the value of the variable “ApartmentNumber”.
- Define the “getMonthlyRent()” method.
- Return the value of the variable “MonthlyRent”.
- Define the “setMonthlyRent ()” method.
- Set the value of the variable “MonthlyRent”.
- Define the “getLeasePeriod()” method.
- Return the value of the variable “leasePeriod”.
- Define the “setLeasePeriod()” method.
- Set the value of the variable “leasePeriod”.
- Define the “addPetFree()” method.
- Set the value of “monthlyRent”.
- Define the “explainPetPolicy()” method.
- Print the statement.
b.
TestLease.java
//import the packages
import java.text.NumberFormat;
import java.util.Scanner;
//define a class TestLease
public class TestLease
{
//define main() method
public static void main(String[] args)
{
//declare the objects of the class Lease
Lease lease1 = new Lease();
Lease lease2 = new Lease();
Lease lease3 = new Lease();
Lease lease4 = new Lease();
//Call three times getdata()
lease1 = getData();
lease2 = getData();
lease3 = getData();
System.out.print("Display info of tenants\n\n");
//Print info
showValues(lease1);
showValues(lease2);
showValues(lease3);
showValues(lease4);
System...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming (MindTap Course List)
- Need help answering this question with a flowchart!arrow_forwardHW: a sewer carry flow = 600 l/s at 34 full at max WWF and 150 l/s at min DWF. Determine the diameter and minimum slope. Then get velocity and depth of sewage flow at max WWF and DWF. Use Vmin = 0.6m/s.arrow_forwardGeneral accountingarrow_forward
- JOB UPDATE Apply on- VinkJobs.com @ OR Search "Vinkjobs.com" on Google COMPANY JOB PROFILE JOB LOCATION INTELLIFLO APPLICATION DEVELOPER MULTIPLE CITIES GLOBAL LOGIC SOFTWARE ENGINEER/SDET DELHI NCR SWIGGY SOFTWARE DEVELOPMENT BENGALURU AVALARA SOFTWARE ENGINEER (WFH) MULTIPLE CITIES LENSKART FULL STACK DEVELOPER MULTIPLE CITIES ACCENTURE MEDPACE IT CUST SERVICE SOFTWARE ENGINEER MUMBAI MUMBAI GENPACT BUSINESS ANALYST DELHI NCR WELOCALIZE WORK FROM HOME MULTIPLE CITIES NTT DATA BPO ASSOCIATE DELHI NCRarrow_forward+is+how+many+tree+in+ipl&rlz=1C1GCEA_enIN1122IN1122&oq=1+dot+ball+is+how+many+tree+in Google 1 dot ball is how many tree in ipl All Images News Videos Short videos Shopping Web More 500 trees 4) हिन्दी में In English The step was a part of the Board of Control for Cricket in India's green initiative. The BCCI, having partnered with the Tata Group, has promised to plant as many as 500 trees for every dot ball bowled in the Indian Premier League. 25 Mar 2025 Sportstar https://sportstar.thehindu.com > Cricket IPL IPL News IPL 2025: Why are green tree symbols showing up for every ... A Translate to fo-d About featured snippets . Feedback Toolsarrow_forwardPastner Brands is a calendar-year firm with operations in several countries. As part of its executive compensation plan, at January 1, 2024, the company issued 480,000 executive stock options permitting executives to buy 480,000 shares of Pastner stock for $38 per share. One-fourth of the options vest in each of the next four years beginning at December 31, 2024 (graded vesting). Pastner elects to separate the total award into four groups (or tranches) according to the year in which they vest and measures the compensation cost for each vesting date as a separate award. The fair value of each tranche is estimated at January 1, 2024, as follows: Vesting Date Amount Fair Value Vesting per Option: December 31, 2024 25% $ 3.90 December 31, 2025 25% $ 4.40 25% $ 4.90 25% $ 5.40 December 31, 2026 December 31, 2027 Required: 1. Determine the compensation expense related to the options to be recorded each year 2024-2027, assuming Pastner allocates the compensation cost for each of the four…arrow_forward
- What is one benefit with regards to time complexity of using a Doubly Linked List as opposed to an Array when implementing a Deque?arrow_forwardWhat is one benefit with regards to space complexity of using a Doubly Linked List as opposed to an Array when implementing a Deque?arrow_forwardWhich basic data structure (Doubly Linked List, Singly Linked List, Array) would you use to implement a Stack? Why?arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
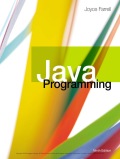
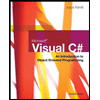
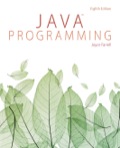
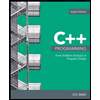
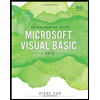