In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays (PrimeList, Palindrome List, Palindromic PrimesList) which will record all the founded numbers from the 3 categories. When any of the threads starts executing, it will print its number (0 to T-1), and then the range of numbers that it is operating on. When all threads are done, the main thread will print the total count of numbers found from each of the 3 categories, in addition to printing all these numbers. You should write two versions of the program: The first one doesn't consider race conditions, and the other one is thread-safe. The input will be provided in an input file (in.txt), and the output should be printed to an output file (out.txt). The number of worker threads will be passed through the command line, as mentioned earlier. The input will be read from a file (in.txt) and will simply have two numbers rangeStart and rangeEnd, which are the beginning and end of the numbers to check for numbers from each category, inclusive. The list of prime numbers will be written to the output file (out.txt), all the other output lines (e.g., prints from threads) will be printed to the standard output terminal (STDOUT). Tasks: In this assignment, you will submit your source code files for the thread-safe and thread-unsafe versions, in addition to a report (PDF file). The report should show the following: 1. Screenshot of the main code. 2. Screenshot of the thread function(s). 3. Screenshot highlighting the parts of the code that were added to make the code thread-safe, with explanations on the need for them. 4. Screenshot of the output of the two versions of your code (thread-safe vs. non- thread-safe), when running passing the following number of threads (T): 1, 4, 16, 64, 256, 1024. 5. Based on your code, how many computing units (e.g., cores, hyper-threads) does your machine have? Provide screenshots of how you arrived at this conclusion, and a screenshot of the actual properties of your machine to validate your conclusion. It is OK if your conclusion doesn't match the actual properties, as long as your conclusion is reasonable. Hints: 1. Plan well before coding, especially on how to divide the range over worker threads. How to synchronize accessing the variables/lists. 2. For passing the number of threads (T) to the code, you will need to use argc and argv as parameters for the main function. For example, the Linux command for running your code with two worker threads (i.e., T=4) will be something like: ./a.out 4. 3. The number of threads (T) and the length of the range can be any number (i.e., not necessarily a power of 2). Your code should try to achieve as much load balancing as possible between threads. 4. For answering Task #5 regarding the number of computing units (e.g., cores, hyper- threads) in your machine, search about "diminishing returns." You also might need to use the Linux command "time" while answering Task #4, and use input with a range of large numbers (e.g., millions).
In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays (PrimeList, Palindrome List, Palindromic PrimesList) which will record all the founded numbers from the 3 categories. When any of the threads starts executing, it will print its number (0 to T-1), and then the range of numbers that it is operating on. When all threads are done, the main thread will print the total count of numbers found from each of the 3 categories, in addition to printing all these numbers. You should write two versions of the program: The first one doesn't consider race conditions, and the other one is thread-safe. The input will be provided in an input file (in.txt), and the output should be printed to an output file (out.txt). The number of worker threads will be passed through the command line, as mentioned earlier. The input will be read from a file (in.txt) and will simply have two numbers rangeStart and rangeEnd, which are the beginning and end of the numbers to check for numbers from each category, inclusive. The list of prime numbers will be written to the output file (out.txt), all the other output lines (e.g., prints from threads) will be printed to the standard output terminal (STDOUT). Tasks: In this assignment, you will submit your source code files for the thread-safe and thread-unsafe versions, in addition to a report (PDF file). The report should show the following: 1. Screenshot of the main code. 2. Screenshot of the thread function(s). 3. Screenshot highlighting the parts of the code that were added to make the code thread-safe, with explanations on the need for them. 4. Screenshot of the output of the two versions of your code (thread-safe vs. non- thread-safe), when running passing the following number of threads (T): 1, 4, 16, 64, 256, 1024. 5. Based on your code, how many computing units (e.g., cores, hyper-threads) does your machine have? Provide screenshots of how you arrived at this conclusion, and a screenshot of the actual properties of your machine to validate your conclusion. It is OK if your conclusion doesn't match the actual properties, as long as your conclusion is reasonable. Hints: 1. Plan well before coding, especially on how to divide the range over worker threads. How to synchronize accessing the variables/lists. 2. For passing the number of threads (T) to the code, you will need to use argc and argv as parameters for the main function. For example, the Linux command for running your code with two worker threads (i.e., T=4) will be something like: ./a.out 4. 3. The number of threads (T) and the length of the range can be any number (i.e., not necessarily a power of 2). Your code should try to achieve as much load balancing as possible between threads. 4. For answering Task #5 regarding the number of computing units (e.g., cores, hyper- threads) in your machine, search about "diminishing returns." You also might need to use the Linux command "time" while answering Task #4, and use input with a range of large numbers (e.g., millions).
Chapter11: Operating Systems
Section: Chapter Questions
Problem 14VE
Related questions
Question

Transcribed Image Text:In this assignment, you will implement a multi-threaded program (using C/C++) that will
check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome
numbers are numbers that their decimal representation can be read from left to right and
from right to left (e.g. 12321, 5995, 1234321).
The program will create T worker threads to check for prime and palindrome numbers in the
given range (T will be passed to the program with the Linux command line). Each of the
threads works on a part of the numbers within the range.
Your program should have some global shared variables:
•
numOfPrimes: which will track the total number of prime numbers found by all
threads.
numOfPalindroms: which will track the total number of palindrome numbers found
by all threads.
numOfPalindromic Primes: which will count the numbers that are BOTH prime and
palindrome found by all threads.
TotalNums: which will count all the processed numbers in the range.
In addition, you need to have arrays (PrimeList, Palindrome List, Palindromic PrimesList)
which will record all the founded numbers from the 3 categories.
When any of the threads starts executing, it will print its number (0 to T-1), and then the
range of numbers that it is operating on. When all threads are done, the main thread will
print the total count of numbers found from each of the 3 categories, in addition to printing
all these numbers.
You should write two versions of the program:
The first one doesn't consider race conditions, and the other one is thread-safe.
The input will be provided in an input file (in.txt), and the output should be printed to an
output file (out.txt). The number of worker threads will be passed through the command
line, as mentioned earlier.
The input will be read from a file (in.txt) and will simply have two numbers rangeStart and
rangeEnd, which are the beginning and end of the numbers to check for numbers from
each category, inclusive.

Transcribed Image Text:The list of prime numbers will be written to the output file (out.txt), all the other output lines
(e.g., prints from threads) will be printed to the standard output terminal (STDOUT).
Tasks: In this assignment, you will submit your source code files for the thread-safe and
thread-unsafe versions, in addition to a report (PDF file). The report should show the
following:
1. Screenshot of the main code.
2. Screenshot of the thread function(s).
3. Screenshot highlighting the parts of the code that were added to make the code
thread-safe, with explanations on the need for them.
4. Screenshot of the output of the two versions of your code (thread-safe vs. non-
thread-safe), when running passing the following number of threads (T): 1, 4, 16, 64,
256, 1024.
5. Based on your code, how many computing units (e.g., cores, hyper-threads) does
your machine have? Provide screenshots of how you arrived at this conclusion, and
a screenshot of the actual properties of your machine to validate your conclusion. It
is OK if your conclusion doesn't match the actual properties, as long as your
conclusion is reasonable.
Hints:
1. Plan well before coding, especially on how to divide the range over worker threads.
How to synchronize accessing the variables/lists.
2. For passing the number of threads (T) to the code, you will need to use argc and argv
as parameters for the main function. For example, the Linux command for running
your code with two worker threads (i.e., T=4) will be something like: ./a.out 4.
3. The number of threads (T) and the length of the range can be any number (i.e., not
necessarily a power of 2). Your code should try to achieve as much load balancing
as possible between threads.
4. For answering Task #5 regarding the number of computing units (e.g., cores, hyper-
threads) in your machine, search about "diminishing returns." You also might need
to use the Linux command "time" while answering Task #4, and use input with a
range of large numbers (e.g., millions).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
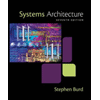
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
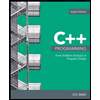
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
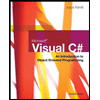
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
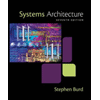
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
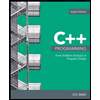
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
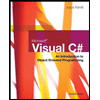
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
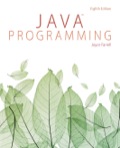
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
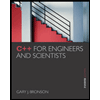
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr