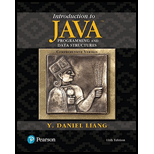
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.4, Problem 21.4.2CP
Program Plan Intro
Set:
Set is a data structure that is used for storing and processing non duplicate elements. It is an interface which extends collection and it is an unordered collection of objects.
Set is implemented by LinkedHashSet, HashSet or a TreeSet.
Hash Set:
In order to store its elements internally, the HashSet uses HashMaps and it implements the Set interface, backed by a hash table which is actually a HashMap instance.
ArrayList:
ArrayList provides dynamic arrays in Java and is a part of collection framework and is present in java.util package.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q: Convert this to sorted array
#include<iostream>
#include"Student.cpp"
class StudentList
{
private:
struct ListNode
{
Student astudent;
ListNode *next;
};
ListNode *head;
public:
StudentList();
~StudentList();
int IsEmpty();
void Add(Student newstudent); void Remove();
void DisplayList();
};
StudentList::StudentList() {
head=NULL;
};
StudentList::~StudentList()
{
cout <<"\nDestructing the objects..\n";
while(IsEmpty()!=0)
Remove();
if(IsEmpty()==0)
cout <<"All students have been deleted from a list\n"; };
int StudentList::IsEmpty()
{
if(head==NULL)
return 0;
else
return 1;
};
void StudentList::Add(Student newstudent) {
ListNode *newPtr=new ListNode;
if(newPtr==NULL)
cout <<"Cannot allocate memory";
else
{
newPtr->astudent=newstudent;
newPtr->next=head;
head=newPtr;
}
};
void StudentList::Remove() {
if(IsEmpty()==0)
cout <<"List empty on remove"; else
{
ListNode *temp=head;…
Remove Char
This function will be given a list of strings and a character. You must remove all occurrences of the character from each string in the list. The function should return the list of strings with the character removed.
Signature:
public static ArrayList<String> removeChar(String pattern, ArrayList<String> list)
Example:list: ['adndj', 'adjdlaa', 'aa', 'djoe']pattern: a
Output: ['dndj', 'djdl', '', 'djoe']
The last part of the code is not completed.
public static void displayArray(int[] list)
{
System.out.println("The sum resulting array is" + Arrays.toString(list));
}
}
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 21.2 - Prob. 21.2.1CPCh. 21.2 - Prob. 21.2.2CPCh. 21.2 - Prob. 21.2.3CPCh. 21.2 - Prob. 21.2.4CPCh. 21.2 - Prob. 21.2.5CPCh. 21.2 - Suppose set1 is a set that contains the strings...Ch. 21.2 - Prob. 21.2.7CPCh. 21.2 - Prob. 21.2.8CPCh. 21.2 - What will the output be if lines 67 in Listing...Ch. 21.2 - Prob. 21.2.10CP
Ch. 21.3 - Prob. 21.3.1CPCh. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores a...Ch. 21.3 - Prob. 21.3.5CPCh. 21.3 - Prob. 21.3.6CPCh. 21.4 - Prob. 21.4.1CPCh. 21.4 - Prob. 21.4.2CPCh. 21.5 - Prob. 21.5.1CPCh. 21.5 - Prob. 21.5.2CPCh. 21.5 - Prob. 21.5.3CPCh. 21.6 - Prob. 21.6.1CPCh. 21.6 - Prob. 21.6.2CPCh. 21.6 - Prob. 21.6.3CPCh. 21.6 - Prob. 21.6.4CPCh. 21.7 - Prob. 21.7.1CPCh. 21.7 - Prob. 21.7.2CPCh. 21 - Prob. 21.1PECh. 21 - (Display nonduplicate words in ascending order)...Ch. 21 - Prob. 21.3PECh. 21 - (Count consonants and vowels) Write a program that...Ch. 21 - Prob. 21.6PECh. 21 - (Revise Listing 21.9, CountOccurrenceOfWords.java)...Ch. 21 - Prob. 21.8PECh. 21 - Prob. 21.9PE
Knowledge Booster
Similar questions
- Question 6 The default capacity of ArrayList object is: 10 50 Capacity must be specified by the user. 100arrow_forwardT/F Suffix array can be created in O(nlogn) time.arrow_forwardion C Lons Employee -id: int -name String - dob : Date -staff : ArrayList +setid(int): void +getld() : int +setName(String): void +setDob(Date) : void +getDob(): Date +addStaff(Employee) : void +getStaff() : ArrayListarrow_forward
- Using C Sharp, Declare an array animals with 5 animals in it such as “dog”, “cat”, etc. After the declaration write the code to add another animal to the array. Write the code to add each animal to a list box called lstAnimals.arrow_forwardWhat distinguishes When will Array be preferred to Array List an array from an array list?arrow_forward2. ID: A Name: 2. A list of numbers is considered increasing if each value after the first is greater than or equal to the preceding value. The following procedure is intended to return true if numberList is increasing and return false otherwise. Assume that numberList contains at least two elements. Line 1: PROCEDURE isIncreasing (numberList) Line 2: { Line 3: count 2 Line 4: REPEAT UNTIL(count > LENGTH(numberList)) Line 5: Line 6: IF(numberList[count] =. YA198ICarrow_forward
- What happens when your program attempts to access a list element with an invalid index?arrow_forwardWhat happens when your program attempts to access an array element with an invalid index?arrow_forwardnarmts Save Answer Write a Java program that uses ArrayList and Iterator. It should input from user the names and ages of your few friends in a loop and add into ArrayList. Finally, it should use Iterator to display the data in a proper format. (Hint- Lecture 02: Slide 8) Sample output: List of my Friends Enter name and age [friend# oj Khalid Al-shamri 22.5 Do you want to add another friend (y/n)? y Enter name and age [friend# 1] Rahsed Al-anazi 21.1 Do you want to add another friend (y/n)? y Enter name and age [friend# 2] Salem Al-mutairi 23.7 Do you want to add another friend (y/n)? n Here is the data you entered: 0. Khalid Al-shamri, 22.5 1. Rahsed Al-anazi, 21.1 2. Salem Al-mutairi, 23.7arrow_forward
- Can enumerators loop across array elements? Why? Why not?arrow_forwardQuick Answer pleasearrow_forwardMatich the following statements with the correct data structure by clicking on the drop down arrow and selecting the correct choice.. You need to store a list of elements and the number of elements in the program is fixed. If most of operations on a list involve retrieving an element at a given index. You have to add or delete the elements at the beginning of a list. Linked List ArrayList Arrayarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
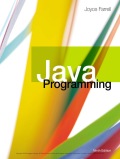
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT