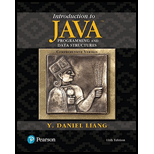
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21.2, Problem 21.2.10CP
Program Plan Intro
Program:
// Java code for adding elements in Set
//import util package
import java.util.*;
//class definition
public class Example_1 {
// main method
public static void main(String[] args)
{
/*Create a new TreeSet and use comparator to compare string length*/
Set<String> set = new TreeSet<>(
Comparator.comparing(String::length));
//add element ABC into the set
set.add("ABC");
//add element and into the set
set.add("ABD");
//print elements present in the set
System.out.println(set);
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 21.2 - Prob. 21.2.1CPCh. 21.2 - Prob. 21.2.2CPCh. 21.2 - Prob. 21.2.3CPCh. 21.2 - Prob. 21.2.4CPCh. 21.2 - Prob. 21.2.5CPCh. 21.2 - Suppose set1 is a set that contains the strings...Ch. 21.2 - Prob. 21.2.7CPCh. 21.2 - Prob. 21.2.8CPCh. 21.2 - What will the output be if lines 67 in Listing...Ch. 21.2 - Prob. 21.2.10CP
Ch. 21.3 - Prob. 21.3.1CPCh. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores a...Ch. 21.3 - Prob. 21.3.5CPCh. 21.3 - Prob. 21.3.6CPCh. 21.4 - Prob. 21.4.1CPCh. 21.4 - Prob. 21.4.2CPCh. 21.5 - Prob. 21.5.1CPCh. 21.5 - Prob. 21.5.2CPCh. 21.5 - Prob. 21.5.3CPCh. 21.6 - Prob. 21.6.1CPCh. 21.6 - Prob. 21.6.2CPCh. 21.6 - Prob. 21.6.3CPCh. 21.6 - Prob. 21.6.4CPCh. 21.7 - Prob. 21.7.1CPCh. 21.7 - Prob. 21.7.2CPCh. 21 - Prob. 21.1PECh. 21 - (Display nonduplicate words in ascending order)...Ch. 21 - Prob. 21.3PECh. 21 - (Count consonants and vowels) Write a program that...Ch. 21 - Prob. 21.6PECh. 21 - (Revise Listing 21.9, CountOccurrenceOfWords.java)...Ch. 21 - Prob. 21.8PECh. 21 - Prob. 21.9PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
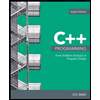
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
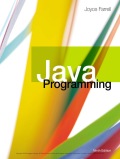
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
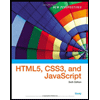
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
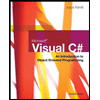
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
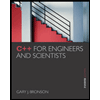
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr