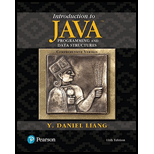
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.2, Problem 21.2.5CP
Program Plan Intro
Set:
Set is a data structure that is used for storing and processing non duplicate elements. It is an interface which extends collection and it is an unordered collection of objects.
Set is implemented by LinkedSet, HashSet or a TreeSet.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
There seems to be a lot of code needed to maintain the node count field in Node. Is it actually required? Why not use a single instance variable to build the size() client function that holds the total number of nodes in the tree?
What are the similarities and differences between a -HashSet and an ArrayList? Use the descriptions of Set, HashSet, List, and -ArrayList in the library documentation to find out, because HashSet is a -special case of a Set, and ArrayList is a special case of a List
Create a lazy elimination deletion function for the AVLTree class.There are several approaches you can take, but one of the simplest is to add a Boolean property to the Node class that indicates whether or not the node is designated for deletion. This variable must then be considered by your other techniques.
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 21.2 - Prob. 21.2.1CPCh. 21.2 - Prob. 21.2.2CPCh. 21.2 - Prob. 21.2.3CPCh. 21.2 - Prob. 21.2.4CPCh. 21.2 - Prob. 21.2.5CPCh. 21.2 - Suppose set1 is a set that contains the strings...Ch. 21.2 - Prob. 21.2.7CPCh. 21.2 - Prob. 21.2.8CPCh. 21.2 - What will the output be if lines 67 in Listing...Ch. 21.2 - Prob. 21.2.10CP
Ch. 21.3 - Prob. 21.3.1CPCh. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores a...Ch. 21.3 - Prob. 21.3.5CPCh. 21.3 - Prob. 21.3.6CPCh. 21.4 - Prob. 21.4.1CPCh. 21.4 - Prob. 21.4.2CPCh. 21.5 - Prob. 21.5.1CPCh. 21.5 - Prob. 21.5.2CPCh. 21.5 - Prob. 21.5.3CPCh. 21.6 - Prob. 21.6.1CPCh. 21.6 - Prob. 21.6.2CPCh. 21.6 - Prob. 21.6.3CPCh. 21.6 - Prob. 21.6.4CPCh. 21.7 - Prob. 21.7.1CPCh. 21.7 - Prob. 21.7.2CPCh. 21 - Prob. 21.1PECh. 21 - (Display nonduplicate words in ascending order)...Ch. 21 - Prob. 21.3PECh. 21 - (Count consonants and vowels) Write a program that...Ch. 21 - Prob. 21.6PECh. 21 - (Revise Listing 21.9, CountOccurrenceOfWords.java)...Ch. 21 - Prob. 21.8PECh. 21 - Prob. 21.9PE
Knowledge Booster
Similar questions
- You are implementing a binary search tree class from scratch, which, in additionto insert, find, and delete, has a method getRandomNode() which returns a random nodefrom the tree. All nodes should be equally likely to be chosen. Design and implement an algorithm for getRandomNode, and explain how you would implement the rest of the methods.arrow_forwardFor the AVLTree class, create a deletion function that makes use of lazy deletion.There are a number of methods you can employ, but one that is straightforward is to merely include a Boolean field in the Node class that indicates whether or not the node is designated for elimination. Then, your other approaches must take into consideration this field.arrow_forwardYou may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forward
- Sort edgeList in non-decreasing order based on the weights of each edge. You will need to write an appropriate comparator to achieve this. Please put this into c++. I only need help with this partarrow_forwardYou are creating from scratch a binary search tree class with the methods insert, find, and delete in addition to the method getRandomNode(), which retrieves a random node from the tree. There should be an equal chance of selecting each node. Create a getRandomNode algorithm, put it into practise, and describe how you'd develop the other functions.arrow_forwardYou've just finished training a random forest for spam classification, and it is getting abnormally bad performance on your validation set, but good performance on your training set. Your implementation has no bugs. What could be causing the problem? Your decision trees are too deep You are randomly sampling too many features when you choose a split You have too few trees in your ensemble Your bagging implementation is randomly sampling sample points without replacementarrow_forward
- Write a deletion method for the AVLTree class that utilizes lazy deletion.There are several techniques you can use, but a simple one is to simplyadd a Boolean field to the Node class that signifies whether or not the nodeis marked for deletion. Your other methods must then take this field intoaccount.arrow_forwardDesign a method random_key() that returns a random key from BST in time proportional to the tree height in the worst case. Please write pseudocode, explain, and state running time. (Note: a ‘size’ field is added to TreeNode class to help with this).arrow_forwardTo finish up the definition of the Node class, we need at least two constructor methods. We definitely want a default constructor that creates an emptyNode, with both the Element and Link members set to null. We also need aparameterized constructor that assigns data to the Element member and setsthe Link member to null.Write the code for the Node class:arrow_forward
- Need help with these true or false questions.arrow_forwardLooking at all four list implementations, which actions/methods tend to be less efficient in the Linked List implementation compared to the Array Implementation? Explain why for each action/method you specify.arrow_forwardPlease answer the question in the screenshot. The language used is Java.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
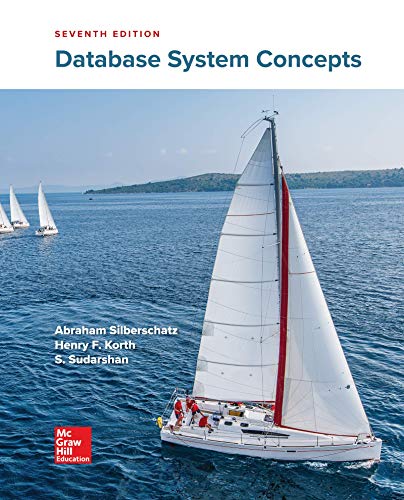
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
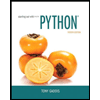
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
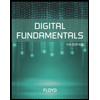
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
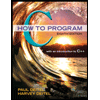
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
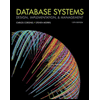
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
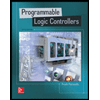
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education