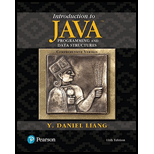
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.6, Problem 21.6.1CP
Program Plan Intro
Map:
Map is an interface which contains values on the basis of key and a value pair and represents a mapping between them. Each key and a value pair in the map are unique.
A map can be of three types: HashMaps, LinkedMaps and TreeMaps.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Information Security Risk and Vulnerability Assessment
1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset?
Please do not use AI and add refrence
Find the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714V
Resolver por superposicion
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 21.2 - Prob. 21.2.1CPCh. 21.2 - Prob. 21.2.2CPCh. 21.2 - Prob. 21.2.3CPCh. 21.2 - Prob. 21.2.4CPCh. 21.2 - Prob. 21.2.5CPCh. 21.2 - Suppose set1 is a set that contains the strings...Ch. 21.2 - Prob. 21.2.7CPCh. 21.2 - Prob. 21.2.8CPCh. 21.2 - What will the output be if lines 67 in Listing...Ch. 21.2 - Prob. 21.2.10CP
Ch. 21.3 - Prob. 21.3.1CPCh. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores a...Ch. 21.3 - Prob. 21.3.5CPCh. 21.3 - Prob. 21.3.6CPCh. 21.4 - Prob. 21.4.1CPCh. 21.4 - Prob. 21.4.2CPCh. 21.5 - Prob. 21.5.1CPCh. 21.5 - Prob. 21.5.2CPCh. 21.5 - Prob. 21.5.3CPCh. 21.6 - Prob. 21.6.1CPCh. 21.6 - Prob. 21.6.2CPCh. 21.6 - Prob. 21.6.3CPCh. 21.6 - Prob. 21.6.4CPCh. 21.7 - Prob. 21.7.1CPCh. 21.7 - Prob. 21.7.2CPCh. 21 - Prob. 21.1PECh. 21 - (Display nonduplicate words in ascending order)...Ch. 21 - Prob. 21.3PECh. 21 - (Count consonants and vowels) Write a program that...Ch. 21 - Prob. 21.6PECh. 21 - (Revise Listing 21.9, CountOccurrenceOfWords.java)...Ch. 21 - Prob. 21.8PECh. 21 - Prob. 21.9PE
Knowledge Booster
Similar questions
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
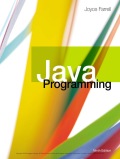
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
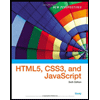
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
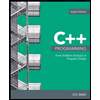
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
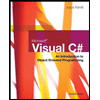
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage