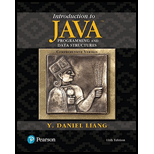
Concept explainers
Program:
//import the util package
import java.util.*;
//class Definition
public class Test {
// main method
public static void main(String[] args) {
//declare a LinkedHashMap
Map<Integer, String> map = new LinkedHashMap<>();
//insert keys and values into the map
map.put("123", "John Smith");
map.put("111", "George Smith");
map.put("123", "Steve Yao");
map.put("222", "Steve Yao");
//print elements present in the map
System.out.println("(1) " + map);
/*print elements in a new Treemap which implements map */
System.out.println("(2) " + new TreeMap<, String>(map));
//check if key equals 123 then print the value
map.forEach((k, v) -> {
if (k.equals("123"))
System.out.println(v);});
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- True or False: Given the sets F and G with F being an element of G, is it always ture that P(F) is an element of P(G)? (P(F) and P(G) mean power sets). Why?arrow_forwardCan you please simplify (the domain is not empty) ∃xF (x) → ¬∃x(F (x) ∨ ¬G(x)). Foarrow_forwardHistogramUse par(mfrow=c(2,2)) and output 4 plots with different argument settings.arrow_forward
- (use R language)Scatter plot(a). Run the R code example, and look at the help file for plot() function. Try different values for arguments:type, pch, lty, lwd, col(b). Use par(mfrow=c(3,2)) and output 6 plots with different argument settings.arrow_forward1. Draw flow charts for each of the following;a) A system that reads three numbers and prints the value of the largest number.b) A system reads an employee name (NAME), overtime hours worked (OVERTIME), hours absent(ABSENT) and determines the bonus payment (PAYMENT).arrow_forwardScenario You work for a small company that exports artisan chocolate. Although you measure your products in kilograms, you often get orders in both pounds and ounces. You have decided that rather than have to look up conversions all the time, you could use Python code to take inputs to make conversions between the different units of measurement. You will write three blocks of code. The first will convert kilograms to pounds and ounces. The second will convert pounds to kilograms and ounces. The third will convert ounces to kilograms and pounds. The conversions are as follows: 1 kilogram = 35.274 ounces 1 kilogram = 2.20462 pounds 1 pound = 0.453592 kilograms 1 pound = 16 ounces 1 ounce = 0.0283 kilograms 1 ounce = 0.0625 pounds For the purposes of this activity the template for a function has been provided. You have not yet covered functions in the course, but they are a way of reusing code. Like a Python script, a function can have zero or more parameters. In the code window you…arrow_forward
- make a screen capture showing the StegExpose resultsarrow_forwardWhich of the following is not one of the recommended criteria for strategic objectives? Multiple Choice a) realistic b) appropriate c) sustainable d) measurablearrow_forwardManagement innovations such as total quality, benchmarking, and business process reengineering always lead to sustainable competitive advantage because everyone else is doing them. a) True b) Falsearrow_forward
- Vision statements are more specific than strategic objectives. a) True b) Falsearrow_forwardThe three components of the __________ approach to corporate accounting include financial, environmental, and social performance measures. Multiple Choice a) stakeholder b) triple dimension c) triple bottom line d) triple efficiencyarrow_forwardCompetitors, as internal stakeholders, should be included in the stakeholder management consideration of a company and in its mission statement. a) True b) Falsearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
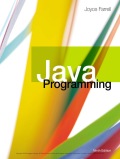
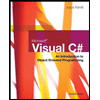
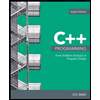
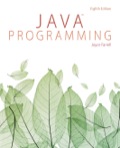