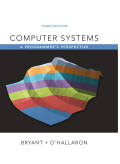
You just started working for a company that is implementing a set of procedures to operate on a data structure where 4 signed bytes are packed into a 32-bit unsigned. Bytes within the word are numbered from 0 (least significant) to 3
(most significant). You have been assigned the task of implementing a function for a machine using two’s-complement arithmetic and arithmetic right shifts with the following prototype:
/* Declaration of data type where 4 bytes are packed
into an unsigned */
typedef unsigned packed_t;
/* Extract byte from word. Return as signed integer */
int xbyte (packed_t word, int bytenum);
That is, the function will extract the designated byte and sign extend it to be a 32-bit int.
Your predecessor (who was fired for incompetence) wrote the following code:
/* Failed attempt at xbyte */
int xbyte (packed_t word, int bytenum)
{
return (word >>(bytenum <<3)) & 0xFF;
}
- A. What is wrong with this code?
- B. Give a correct implementation of the function that uses only left and right shifts, along with one subtraction.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
EBK COMPUTER SYSTEMS
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Concepts Of Programming Languages
Starting Out with C++ from Control Structures to Objects (9th Edition)
- In the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forward
- Here are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forwardI'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
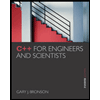
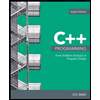
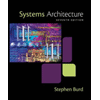
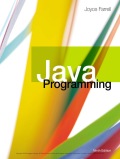
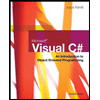