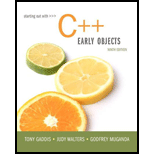
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 5PC
Program Plan Intro
List Member Deletion
Program Plan:
- Include the required specifications into the program.
- Declare a class ListNode.
- Declare the member variables “value” and “*p” in structure named “ListNode”.
- The data value of node is stored in variable v and address to next pointer is stored in pointer p
- Declare the constructor, destructor, and member functions in the class.
- Declare the structure variable “next” and a friend class Linked List
- Declare a class LinkList.
- Function to insert elements into the linked list “void add(double n)”is defined.
- Function to check whether a particular node with a data value n is a part of linked list or not “bool isMember(double n)”.
- A recursive print method is defined to print all the data values present in the link list “void rPrint()”.
- A destructor is called to delete the desired data value entered by the user called “LinkedList::~LinkedList( )”.
- A method to remove the element passed as a parameter from the link list is called “void LinkedList::remove(double x)”.
- Declaration of structure variable head to store the first node of the list “ListNode * head” is defined.
- A function “void LinkedList::add(double n)” is defined which adds or inserts new nodes into the link list.
- A function “bool LinkedList::isMember(double n)” is defined which searches for a given data value within the nodes present in the link list.
- A destructor “LinkedList::~LinkedList()” deallocates the memory for the link list.
- A function “void LinkedList::print()” is used to print all the node data values present in the link list by traversing through each nodes in the link list.
- A recursive member function check is defined called “bool LinkedList::rIsMember(ListNode *pList,double x)” .
- If the data value entered is present within the link list, it returns true, else it returns false.
- Declare the main class.
- Create an empty list to enter the data values into the list.
- Copy is done using copy constructor.
- Input “5” numbers from user and insert the data values into the link list calling “void LinkedList::add(double n)” function.
- Print the data values of the nodes present in the link list.
- Ask the user to enter an element to be removed.
- A loop is executed to traverse through the list and find the value entered.
- If the element is found, thelist1.remove (number) is called and the element is removed.
- The remaining list elements are printed.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
struct nodeType {
int infoData;
nodeType * next;
};
nodeType *first;
… and containing the values(see image)
Using a loop to reach the end of the list, write a code segment that deletes all the nodes in the list. Ensure the code performs all memory ‘cleanup’ functions.
Grocery shopping list (linked list: inserting at the end of a list)
Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4
Kale
Lettuce
Carrots
Peanuts
where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list.
The output is:
Kale
Lettuce
Carrots
Peanuts
True or False The objects of a class can be stored in an array, but not in a List.
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ function Linked list Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.arrow_forwardC++ Question You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the…arrow_forwardC++ Question You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the…arrow_forward
- C++ ProgrammingActivity: Deque Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "deque.h" #include "linkedlist.h" #include <iostream> using namespace std; class DLLDeque : public Deque { DoublyLinkedList* list; public: DLLDeque() { list = new DoublyLinkedList(); } void addFirst(int e) { list->addAt(e,1); } void addLast(int e) { list->addAt(e,size()+1); } int removeFirst() { return list->removeAt(1); } int removeLast() { return list->removeAt(size()); } int size(){ return list->size(); } bool isEmpty() { return list->isEmpty(); } // OPTIONAL: a helper method to help you debug void print() {…arrow_forward6. Suppose that we have defined a singly linked list class that contains a list of unique integers in ascending order. Create a method that merges the integers into a new list. Note the additional requirements listed below. Notes: ● . Neither this list nor other list should change. The input lists will contain id's in sorted order. However, they may contain duplicate values. For example, other list might contain id's . You should not create duplicate id's in the list. Important: this list may contain duplicate id's, and other list may also contain duplicate id's. You must ensure that the resulting list does not contain duplicates, even if the input lists do contain duplicates.arrow_forwardC++ Programming Language ::::::: Redo the same functions this time as nonmember functions please : NOTE: You can add only one function into the linked list class get_at_position which will return value of element at given position. 1) Insert before tail : Insert a value into a simply linked list, such that it's location will be before tail. So if a list contains {1, 2, 3}, insert before tail value 9 is called, the list will become {1, 2, 9, 3}. 2) Insert before value : Insert a value into a simply linked list, such that it's location will be before a particular value. So if a list contains {1, 2, 3}, insert before 2 value 9 is called, the list will become {1, 9, 2, 3}. 3)Count common elements : Count common values between two simply linked lists.So if a list1 contains {1, 2, 3, 4, 5}, and list2 contains {1, 3, 4, 6}, number of common elements is 3. 4) Check if sorted : Check if elements of simply linked lists are sorted in ascending order or not.So if a list contains {1, 3, 7, 8, 9}…arrow_forward
- class Node: def __init__(self, e, n): self.element = e self.next = n class LinkedList: def __init__(self, a): # Design the constructor based on data type of a. If 'a' is built in python list then # Creates a linked list using the values from the given array. head will refer # to the Node that contains the element from a[0] # Else Sets the value of head. head will refer # to the given LinkedList # Hint: Use the type() function to determine the data type of a self.head = None # To Do # Count the number of nodes in the list def countNode(self): # To Do # Print elements in the list def printList(self): # To Do # returns the reference of the Node at the given index. For invalid index return None. def nodeAt(self, idx): # To Doarrow_forwardCircular linked list is a form of the linked list data structure where all nodes are connected as in a circle, which means there is no NULL at the end. Circular lists are generally used in applications which needs to go around the list repeatedly. struct Node * insertTONull (struct Node *last, int data) // This function is only for empty list 11 5 15 struct Node insertStart (struct Node +last, int data) In this question, you are going to implement the insert functions of a circular linked list in C. The Node struct, print function and the main function with its output is given below: struct Node { int data; struct Node *next; }; struct Node insertEnd (struct Node *last, int data) void print(struct Node *tailNode) struct Node *p; if (tailNode -- NULL) struct Node * insertSubseq (struct Node *last, int data, int item) puts("Empty"); return; p - tailNode → next; do{ printf("%d ",p→data); p - p > next; while(p !- tailNode →next); void main(void) { struct Node *tailNode - NULL; tailNode -…arrow_forwardstruct remove_from_front_of_dll { // Function takes no parameters, removes the book at the front of a doubly // linked list, and returns nothing. void operator()(const Book& unused) { //// TO-DO (13) |||| // Write the lines of code to remove the book at the front of "my_dll", // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. ///// END-TO-DO (13) //// } std::list& my_dll; };arrow_forward
- An iterable is an object that is similar to a list and is created by the range function True or Falsearrow_forward@6 The Reference-based Linked Lists: Select all of the following statements that are true. options: As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forwardcard_t * moveCardBack (card t *head); The moveCardBack function will take the card in front of the pile and place it in the back. In coding terms, you are taking the head of the linked list and moving it to the end. The function has one parameter which is the head of the linked list. After moving the card to the back, the function returns the new head of the linked list.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
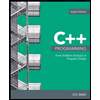
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning