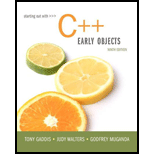
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 15RQE
Program Plan Intro
List or Linked list:
Linked list is a linear and dynamic data structure which is used to organize data; it contains sequence of elements which are connected together in memory to form a chain. The every element of linked list is called as a node.
List head:
List head is a pointer used to point the first node in the linked list and it is also called as “head pointer”; if the “head pointer” points to a “NULL” value, then it is considered that the “list is empty”.
Graphical representation of linked list:
The graphical representation of a linked list is as follows:
cout statement:
“cout” stands for standard output stream.
- It is the c++ stream used to access the standard output, which in this case is the output screen.
- “cout” statement is followed by the “<<” operator which is called the insertion operator.
- “<<” operator inserts the information that tails it into the stream and user can provide the required output.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
card_t * moveCardBack (card t *head);
The moveCardBack function will take the card in front of the pile and place it in the back. In coding
terms, you are taking the head of the linked list and moving it to the end. The function has one parameter
which is the head of the linked list. After moving the card to the back, the function returns the new head
of the linked list.
#include
using namespace std;
struct ListNode {
string data;
ListNode *next;
};
int main() {
ListNode *p, *list;
list = new ListNode;
list->data = "New York";
p new ListNode;
p->data = "Boston";
list->next = p;
p->next = new ListNode;
p->next->data = "Houston";
p->next->next = nullptr;
// new code goes here
Which of the following code correctly deletes the node with value "Boston" from the list when added at point of insertion
indicated above?
O list->next = p;
delete p;
O p = list->next;
%3D
list->next = p->next;
delete p;
p = list->next;
list = p->next;
delete p;
O None of these
O p = list->next;
%3D
list->next = p;
%3D
delete p;
The definition of linked list is given as follows:
struct Node {
ElementType Element ;
struct Node *Next ;
} ;
typedef struct Node *PtrToNode, *List, *Position;
If L is head pointer of a linked list, then the data type of L should be ??
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question 9 #include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *ptr, *list; list = new ListNode; list->data = "New York"; ptr = new ListNode; ptr->data = "Boston"; list->next = ptr; ptr->next = new ListNode; ptr->next->data = "Houston"; ptr->next->next = nullptr; // new code goes here Which of the following code correctly deletes the last node of the list when added at point of insertion indicated above? O delete ptr->next; ptr->next = nullptr;; O ptr = list; while (ptr != nullptr) ptr = ptr->next; delete ptr; O ptr = last; delete ptr; list->next->next = nullptr; delete ptr; O None of thesearrow_forwardIn C, using malloc to allocate memory for a linked list uses which memory allocation scheme? Heap allocation Static allocationarrow_forwardion C Lons Employee -id: int -name String - dob : Date -staff : ArrayList +setid(int): void +getld() : int +setName(String): void +setDob(Date) : void +getDob(): Date +addStaff(Employee) : void +getStaff() : ArrayListarrow_forward
- Topic: Linked List SHOW THE OUTPUT ONLYarrow_forwardstruct node{ int a; struct node * nextptr; }; Write two functions. One for inserting new values to a link list that uses the given node structure. void insert(struct node **head, int value); Second function is called to count the number of even numbers in the link list. It returns an integer that represents the number of even numbers. int countEvenNumbers(struct node *head); Write a C program that reads a number of integers from the user and insert those integers into a link list (use insert function). Later pass the head pointer of this link list to a function called countEvenNumbers. This function counts and returns the number of even numbers in the list. The returned value will be printed on the screen. Note 1: Do not modify the function prototypes. Sample Input1: Sample Output1: 45 23 44 12 37 98 33 35 -1 3 Sample Input2: Sample Output2: 11 33 44 21 22 99 123 122 124 77 -1 4arrow_forwardIn C++ Write the DLList member function DLLNode<T>* DLLidt<T>::get_at(int index) function that return the node at specific index in the list.arrow_forward
- Q: Convert this to sorted array #include<iostream> #include"Student.cpp" class StudentList { private: struct ListNode { Student astudent; ListNode *next; }; ListNode *head; public: StudentList(); ~StudentList(); int IsEmpty(); void Add(Student newstudent); void Remove(); void DisplayList(); }; StudentList::StudentList() { head=NULL; }; StudentList::~StudentList() { cout <<"\nDestructing the objects..\n"; while(IsEmpty()!=0) Remove(); if(IsEmpty()==0) cout <<"All students have been deleted from a list\n"; }; int StudentList::IsEmpty() { if(head==NULL) return 0; else return 1; }; void StudentList::Add(Student newstudent) { ListNode *newPtr=new ListNode; if(newPtr==NULL) cout <<"Cannot allocate memory"; else { newPtr->astudent=newstudent; newPtr->next=head; head=newPtr; } }; void StudentList::Remove() { if(IsEmpty()==0) cout <<"List empty on remove"; else { ListNode *temp=head;…arrow_forwarddef animals(animal_dict, target): """ Filter animals based on the target category. :param animal_dict: A dictionary mapping animals to their categories. :type animal_dict: dict :param target: The target category to filter animals. :type target: str :return: A list of animals belonging to the target category. :rtype: list animals_dict = {"dogs": "mammals", "snakes": "reptiles", \ "dolphins": "mammals", "sharks": "fish"} >>> target = "mammals" >>> animals(animals_dict, target) ['dogs', 'dolphins'] >>> animals_dict = {True: "mammals"} >>> animals(animals_dict, target) # This will raise an AssertionError Traceback (most recent call last): ... AssertionError: Target should be provided and not None """ assert target is not None, "Target should be provided and not None" assert isinstance(animal_dict, dict) assert all(isinstance(animal, str) for animal in animal_dict.keys())…arrow_forwarddef animals(animal_dict, target): """ Filter animals based on the target category. :param animal_dict: A dictionary mapping animals to their categories. :type animal_dict: dict :param target: The target category to filter animals. :type target: str :return: A list of animals belonging to the target category. :rtype: list animals_dict = {"dogs": "mammals", "snakes": "reptiles", \ "dolphins": "mammals", "sharks": "fish"} >>> target = "mammals" >>> animals(animals_dict, target) ['dogs', 'dolphins'] >>> animals_dict = {True: "mammals"} >>> animals(animals_dict, target) # This will raise an AssertionError Traceback (most recent call last): ... AssertionError """ assert target is not None, "Target should be provided and not None" assert isinstance(animal_dict, dict) assert all(isinstance(animal, str) for animal in animal_dict.keys()) assert all(isinstance(category, str) for…arrow_forward
- IntSet unionWith(const IntSet& otherIntSet) const; //Unions the current set with the passed set. IntSet::unionWith(const IntSet& otherIntSet){ for (int i = 0; i < otherIntSet.used; ++i) //For each element in the passed set. { add(otherIntSet.data[i]); //Add it to current set. }}arrow_forwardConcatenate Map This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map. You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps. Signature: public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList) Example: INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93} INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43} INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…arrow_forwardin cpp please AnyList.h #ifndef ANYLIST_H#define ANYLIST_H #include<iostream>#include <string> //Need to include for NULL class Node{public: Node() : data(0), ptrToNext(nullptr) {} Node(int theData, Node *newPtrToNext) : data(theData), ptrToNext(newPtrToNext){} Node* getPtrToNext() const { return ptrToNext; } int getData( ) const { return data; }void setData(int theData) { data = theData; } void setPtrToNext(Node *newPtrToNext) { ptrToNext = newPtrToNext; } ~Node(){}private:int data; Node *ptrToNext; //pointer that points to next node}; class AnyList{public: AnyList(); void insertFront(int); void print() const; void destroyList(); ~AnyList(); private: Node *ptrToFirst; //pointer to point to the first node in the list int count; //keeps track of number of nodes in the list}; #endif AnyList.cpp #include "AnyList.h" using namespace std; //constructorAnyList::AnyList(){ ptrToFirst = nullptr; count =…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
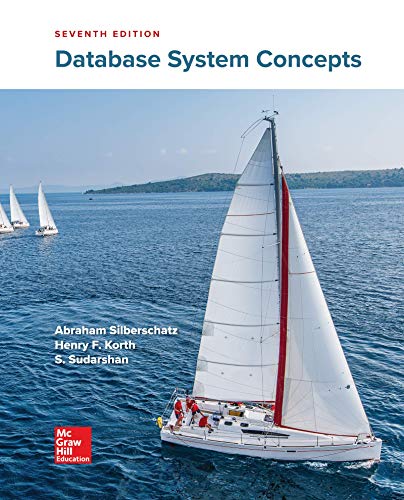
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
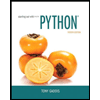
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
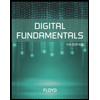
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
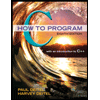
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
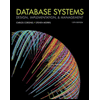
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
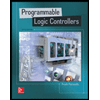
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education