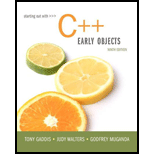
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 4PC
Program Plan Intro
Recursive Member Check
Program Plan:
- Include the required specifications into the program.
- Declare a class ListNode.
- Declare the member variables “value” and “*p” in structure named “ListNode”.
- The data value of node is stored in variable v and address to next pointer is stored in pointer p.
- Declare the constructor, destructor, and member functions in the class.
- Declare the structure variable “next” and a friend class Linked List.
- Declare a class LinkList.
- Function to insert elements into the linked list “void add(double n)” is defined.
- Function to check whether a particular node with a data value n is a part of linked list or not “bool isMember(double n)”.
- A destructor to check whether the data value entered is a member function or not is defined “bool isMember(double x){ return rIsMember(head, x);}~LinkedList()”.
- Declaration of structure variable head to store the first node of the list “ListNode * head” is defined.
- A function “void LinkedList::add(double n)” is defined which adds or inserts new nodes into the link list.
- A function “bool LinkedList::isMember(double n)” is defined which searches for a given data value within the nodes present in the link list.
- A destructor “LinkedList::~LinkedList()” deallocates the memory for the link list.
- A function “void LinkedList::print()” is used to print all the node data values present in the link list by traversing through each nodes in the link list.
- A recursive member function check is defined called “bool LinkedList::rIsMember(ListNode *pList,double x)” .
- If the data value entered is present within the link list, it returns true, else it returns false.
- Declare the main class.
- Create an empty list to enter the data values into the list.
- Copy is done using copy constructor.
- Input “5” numbers from user and insert the data values into the link list calling “void LinkedList::add(double n)” function.
- Print the data values of the nodes present in the link list.
- Check whether the data value entered is a member of the link list or not by calling “list1.isMember(x)” function.
- If the data value entered is present within the link list, it prints member, else it prints not member.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Need help making python code for this!
2.7 LAB: Smallest of two numbers
Instructor note:
Note: this section of your textbook contains activities that you will complete for points. To ensure your work is scored, please access this page from the assignment link provided in the CTU Virtual Campus. If you did not access this page via the CTU Virtual Campus, please do so now.
I help understanding this question
d'y + 4dy +3y = a, Initial Conditions: y(0) = 5 & y'(0)=0
Where a = 10
a) Find y(t) =yh(t) +yp(t) in time domainIs the system over-damped, under-damped, or critical?
b) Find y(t) using Laplace Transforms
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Given f(t)=a sin(ßt) a = 10 & ß = 23 Find the Laplace Transform using the definition F(s) = ∫f(t)e-stdtarrow_forwardPlease do not use any AI tools to solve this question. I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor. No AI-generated responses, please.arrow_forwardObtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).arrow_forward
- I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ? Cisco Packet Tracer 1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardusing r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forward
- using r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forwardusing r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forward
- A. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forwardCisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
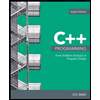
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
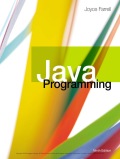
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
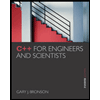
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
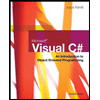
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
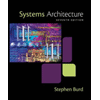
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning