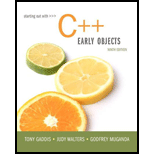
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 17, Problem 3RQE
Program Description Answer
User should set the pointer to its head to the value “NULL” to indicate that a linked list is empty.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
class Node: def __init__(self, e, n): self.element = e self.next = n
class LinkedList: def __init__(self, a): # Design the constructor based on data type of a. If 'a' is built in python list then # Creates a linked list using the values from the given array. head will refer # to the Node that contains the element from a[0] # Else Sets the value of head. head will refer # to the given LinkedList
# Hint: Use the type() function to determine the data type of a self.head = None # To Do # Count the number of nodes in the list def countNode(self): # To Do # Print elements in the list def printList(self): # To Do
# returns the reference of the Node at the given index. For invalid index return None. def nodeAt(self, idx): # To Do
struct node{
int a;
struct node * nextptr;
};
Write two functions. One for inserting new values to a link list that uses the given node structure.
void insert(struct node **head, int value);
Second function is called to count the number of even numbers in the link list. It returns an integer that represents the number of even numbers.
int countEvenNumbers(struct node *head);
Write a C program that reads a number of integers from the user and insert those integers into a link list (use insert function). Later pass the head pointer of this link list to a function called countEvenNumbers. This function counts and returns the number of even numbers in the list. The returned value will be printed on the screen.
Note 1: Do not modify the function prototypes.
Sample Input1:
Sample Output1:
45 23 44 12 37 98 33 35 -1
3
Sample Input2:
Sample Output2:
11 33 44 21 22 99 123 122 124 77 -1
4
C++ Programming Language ::::::: Redo the same functions this time as nonmember functions please :
NOTE: You can add only one function into the linked list class get_at_position which will return value of element at given position.
1) Insert before tail : Insert a value into a simply linked list, such that it's location will be before tail.
So if a list contains {1, 2, 3}, insert before tail value 9 is called, the list will become {1, 2, 9, 3}.
2) Insert before value : Insert a value into a simply linked list, such that it's location will be before a particular value.
So if a list contains {1, 2, 3}, insert before 2 value 9 is called, the list will become {1, 9, 2, 3}.
3)Count common elements : Count common values between two simply linked lists.So if a list1 contains {1, 2, 3, 4, 5}, and list2 contains {1, 3, 4, 6}, number of common elements is 3.
4) Check if sorted : Check if elements of simply linked lists are sorted in ascending order or not.So if a list contains {1, 3, 7, 8, 9}…
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- ASSUMING C LANGUAGE True or False: You can have the data portion of a Linked List be a Struct containing a Linked List itselfarrow_forwardnumUniqueValues ♡ Language/Type: Related Links: Java Set collections List Write a method named numUnique Values that accepts a List of integers as a parameter and returns the number of unique integer values in the list. For example, if a list named 1 contains the values [3, 7, 3, -1, 2, 3, 7, 2, 15, 15], the call of numUniqueValues (1) should return 5. If passed the empty list, you should return 0. Use a Set as auxiliary storage to help you solve this problem. Do not modify the list passed in. 6 7 8 9 10 Method: Write a Java method as described, not a complete program or class. 12345arrow_forward@6 The Reference-based Linked Lists: Select all of the following statements that are true. options: As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- card_t * moveCardBack (card t *head); The moveCardBack function will take the card in front of the pile and place it in the back. In coding terms, you are taking the head of the linked list and moving it to the end. The function has one parameter which is the head of the linked list. After moving the card to the back, the function returns the new head of the linked list.arrow_forwardstruct remove_from_front_of_dll { // Function takes no parameters, removes the book at the front of a doubly // linked list, and returns nothing. void operator()(const Book& unused) { //// TO-DO (13) |||| // Write the lines of code to remove the book at the front of "my_dll", // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. ///// END-TO-DO (13) //// } std::list& my_dll; };arrow_forwardC# Assume you have a LinkedList of Node objects. Both classes have all the normal operations shown below. Your job is to program the DeleteTail method of the LinkedList class. This method locates and deletes the last element of the linked list. You may not change its signature line. Keep your code clean, but no documentation is necessary. A good solution will be between 5 and 10 lines of code, not counting whitespace.arrow_forward
- struct nodeType { int infoData; nodeType * next; }; nodeType *first; … and containing the values(see image) Using a loop to reach the end of the list, write a code segment that deletes all the nodes in the list. Ensure the code performs all memory ‘cleanup’ functions.arrow_forwarddef small_index(items: list[int]) -> int:"""Return the index of the first integer in items that is less than its index,or -1 if no such integer exists in items.>>> small_index([2, 5, 7, 99, 6])-1>>> small_index([-5, 8, 9, 16])0>>> small_index([5, 8, 9, 0, 1, 3])3"""arrow_forwarda) Write a function to get the value of the Nu node in a Linked List. [Note: The first (N=1) item in the list means the item at index 0.] It takes two parameters: the list or its head, and N. Return False if the list has fewer than N elements. The Linked List structure supports the following function. def getlead(self): return selt.head # it points to a Node structure The Node structure supports the following functions. def getData(self): return self.data # it returns the value stored in the Node def getNext(self): return self next # it points to the next Node b) Write a function that counts the number of times a given integer occurs in a Linked List. Assume similar structures as defined in 1.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
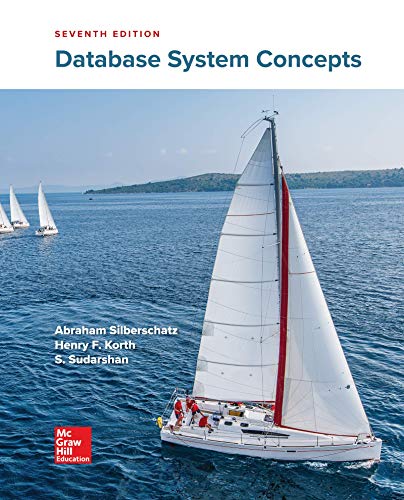
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
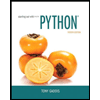
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
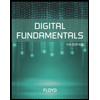
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
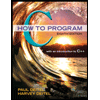
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
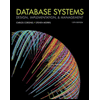
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
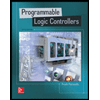
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education