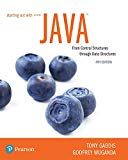
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 16.1, Problem 16.4CP
Explanation of Solution
Quick sort:
- This is sorting
algorithm in which it sorts the array by dividing the list into two sub lists. - After dividing the lists, choose the pivot element between the lists.
- After selecting the pivot element, the algorithm reorders the values in the array until all the elements in left sub list is lesser than the pivot.
- Next, it sorts all the elements in the right sub list which are greater than or equal to pivot.
- Then, this algorithm recursively repeat the steps on sub list 1 and sub list 2...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
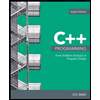
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
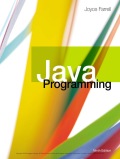
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT