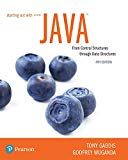
Explanation of Solution
The following
Pseudocode:
Input: Two integer arrays “a[]” and “b[]” of size “n”.
Size of Input: The size specifies the number “n” of array entries.
Output: The condition is “true” of each element of “a[]” is also an element of “b[]” otherwise, it display “false”.
The following is the pseudocode for given data,
Boolean Contains =true
int i=0
While Contains && i<n do
Contains=Linearsearch(a[i],b)
i++;
End while
Print Contains
Explanation:
- In the given code, set the Boolean variable “Contains” is equal to “true”.
- Execute the while loop if the condition is true,
- The condition must be “i” less than “n” and the variable “Contains” must be “true”.
- If it is true, then it calls for Linearsearch() function.
- Increment the value of “i”.
- Thus, the while loop is executed until the condition satisfies and then finally it print the Boolean value either “True” or “False”.
Pseudocode for LinearSearch:
Input: An integer “X” and an array “b[]” of size “n”.
Size of input: The size specifies the number “n” of array entries.
Output: It returns true if “X” contains in “b[]”, otherwise “false”.
The following is the pseudocode for linear search,
Boolean LinSearch(int x, int[] b)
Begin
int k=0;
Boolean found =false;
While !found && k<n do
if(X==b[k])
found =true;
else
K++
End If
End While
Return found
End LinSearch
Explanation:
- The given code performs the linear search algorithm concept which process to find an item in an array...

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Find the error: daily_sales = [0.0, 0,0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': ')arrow_forwardFind the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(6): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': '))arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it and normalize it? Give two references with your answer.arrow_forward
- What are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Consider that the database offline is not allowed since people are connected to it and personal data might be bridged and not secured. Provide three refernces with you answer.arrow_forwardShould software manufacturers should be tolerant of the practice of software piracy in third-world countries to allow these countries an opportunity to move more quickly into the information age? Why or why not?arrow_forwardI would like to know about the features of Advanced Threat Protection (ATP), AMD-V, and domain name space (DNS).arrow_forward
- I need help to resolve the following activityarrow_forwardModern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningPrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage Learning
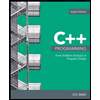
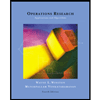
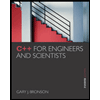
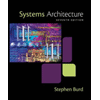
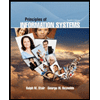