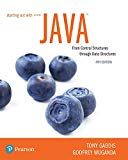
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 8PC
Program Plan Intro
Sorting Benchmarks
Program plan:
- Create the class “SortingBenchmarks”,
- Declare the required variables.
- Define the method “bubbleSort()”,
- Use “for” loop to sort the elements in the ascending order.
- Increment the count of bubble sort by 2 on each comparison and swaps on the elements.
- Return the count of bubble sort.
- Define the method “selectionSort()”,
- Use “for” loop to find the smallest value in the array and continue the process until the array becomes sorted.
- Increment the count of selection sort by 2 on each comparison and swaps on the elements.
- Return the count of selection sort.
- Define the method “insertionSort()”,
- Use “for” loop to insert the elements at the correct position and swap the elements based on comparisons.
- Increment the insertion sort by 1.
- Return the count of insertion sort.
- Define the method “quicksort()”,
- Call the method “doquicksort()” to sort the elements using the pivot value.
- Increment the quick sort by 1.
- Define the method “doquick_sort()”,
- Call the method “partition()” to partition the array values into two halves.
- Call the method “doquick_sort()” recursively to sort the left half of the values in the array.
- Call the method “doquick_sort()” recursively to sort the right half of the values in the array.
- Define the method “partition()”,
- Calculate the pivot value.
- Call the “swap()” method to swap the array values.
- Use “for” loop to scan the entire array and based on pivot value, call the “swap()” method to swap and arrange the values in order.
- Define the method “swap()”,
- Swap the values and increment the quick sort count by 2.
- Create the class “SortingBenchmarkTest”,
- Define the “main()” function,
- Use “for” loop to insert random of at least 20 integers for each sorting.
- Create the object for the “SortingBenhcmarkTest” class,
- Call the method “bubbleSort()” to sort the values in order.
- Call the method “selectionSort()” to sort the values in order.
- Call the method “insertionSort()” to sort the values in order.
- Call the method “quickSort()” to sort the values in order.
- Print the sorted elements of each sorting and then print the number of comparisons made by each sorting.
- Define the “main()” function,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Java:
8. Repetition Use Python Language
Write a method that takes in an array as a
parameter and counts the repetition of
each element. That is, if an element has
appeared in the array more than once, then
its 'repetition' is its number of occurrences.
The method returns true if there are at least
two elements with the same number of
'repetition'. Otherwise, return false.
Input: {4,5,6,6,4,3,6,4} Output: True
Explanation: Two numbers repeat in this
array: 4 and 6. 4 has a repetition of 3, 6
has a repetition of 3. Since two numbers
have the same repetition output is True.
Input: {3,4,6,3,4,7,4,6,8,6,6} Output: False
Explanation: Three numbers repeat in this
array:3,4 and 6 .3 has a repetition of 2, 4
has a repetition of 3, 6 has a repetition of 4.
Since no two numbers have the same
repetition output is False.
Task 3: Statistics using arrays:
by java programming
With the spread of COVID 19, the HR department in a company has decided to conduct some statistics
among the employees in order to determine the number of infections according to some conditions.
For each employee, they have to record the code, name, age, whether he/she was infected or no and
the remaining days of leaves for him/her.
You are requested to write the program that maintains the lists of details for the employees as
mentioned above using the concept of arrays. The program repeats the display of a menu of services
until the user decides to exit.
1. Start by initializing the employee details by reading them from the keyboard.
2. Repeat the display of a menu of 4 services, perform the required task according to the user’s
choice and asks the user whether he/she wants to repeat or no. You need to choose one service
from each category (‘A’,’B’,’C’,’D’)
a. A. Display the total number of employees that were infected
b. B.…
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Assignment Write a program that reads a 2D array of chars, checks, and corrects each char within this array, and print out the corrected 2D array. First the program will read the row count (rowCount) and column count (columnCount) of the 2D array, both as int values. Then, the chars constituting the 2D array will be read on a row-by-row basis. After reading the 2D array, the program will check and correct each char within the 2D array according to the rules below. This process MUST be done by using a void function by sending the appropriate cell of the 2D array with a call-by-reference fashion. • If the row index is an even number, a char can only be a lowercase English value. • If the row index is an odd number, a char can only be ?, 7', or X'. • Any incorrect value MUST be changed into Finally, the checked and corrected 2D array will be printed out to the screen. NOTE: Checking the correctness of a char and correcting it (if necessary) MUST be done in the function with a…arrow_forwardTrue or False. A simple variable, also called a scalar variable, is one that is unrelated to any other variable in the computer’s internal memory. The bubble sort algorithm gets its name from the fact that as the larger values drop to the bottom of the array, the smaller values rise, like bubbles, to the top. Programmers use arrays to temporarily store related data in the internal memory of the computer. Different variables in the same array may have different data types. The elements in an array can be used just like any other variables. When an array is sorted in ascending order, the first element contains the largest value and the last element contains the smallest value. When passing an array by reference in C++, you do not include the address-of operator before the formal parameter’s name in the function header. You distinguish one variable in a one-dimensional array from another variable in the same array using a unique integer,…arrow_forwardFinding the common members of two dynamic arrays: Write a program that first reads two arrays and then finds their common members (intersection). The program should first read an integer for the size of the array and then should create the two arrays dynamically (using new) and should read the numbers into array1 and array2 and then should find the common members and show it. Note: The list of the common members should be unique. That is no member should be listed more than once. Tip: A simple for loop is needed on one of the arrays (like array1) and one inner for loop for on array2. Then for each member of array1, if we find any matching member in array2, we record it. Sample run: Enter the number of elements of array 1: 7 Enter the elements of array 1: 10 12 13 10 14 15 12 Enter the number of elements of array 2: 5 Enter the elements of array 2: 17 12 10 19 12 The common members of array1 and array2 are: 12 10arrow_forward
- C Program/ C Language Make a C program for the array. Enter a bunch of integers then it should print the largest sum of a strictly ascending sequence of the array. A strictly ascending sequence is a sequence where the current number is always lesser than the next number.arrow_forwardCoding solutionarrow_forwardC LANGUAGE Randomly generate 50 integers within the range of 0 – 999. Store the integers in an array.Pass the array to different functions that perform the tasks below:i. Print out the randomly generated integers in 5 rows, each row having 10 integer.Input: arrayreturn: none Random integer generation: #include<stdlib.h> rand(); //generate random integer rand()%999; //generate random integer between 0-999 ii. Show the range of integers generated: Output the smallest and largest integer and their index in the array.Input: arrayreturn: none iii. Sort the integers in ascending orderInput: arrayreturn: none findsmallestnumber(int start, int end, array)input: start index of array, end index of array, arrayreturn: smallest number index or position in array iv. Generate another array of 50 integers. Compare the 2 arrays and count the similarity percentage of the 2 arrays.Input: Array1, Array2Return: none ** Use 2 for loops for this function,…arrow_forward
- Best Partition You are given an array of positive numbers of size N and an integer K. You need to partition the array into K continuous segments. For each segment, the sum of its elements needs to be calculated. The segment with the minimum sum is called the bestSegment and the sum of the elements of the bestSegment is called the bestSum. For all possible combinations of partitions of the array when divided into K segments, their bestSum needs to be calculated and the one among them with maximum value needs to be returned. Input Specification: input1: an array of N positive numbers input2: an integer N denoting the length of the array input3: an integer K Output Specification: Return an integer denoting the maximum value of all possible bestSum. Example 1: input1: (1,2,3,4} input2: 4 input3: 2 Output: 4 Explanation: You can partition the given array into 2 continuous segments in the following manner- • 123 14- the sum of individual segments is (6,4) and the bestSum is 4 • 12134- the…arrow_forwardARRAY RANDOMIZER Create a program that shuffels all the elements present in an array. To shuffle an array, you need to do at least 500 random swaps of any elements in the array given below. Print the shuffled array in the output. {12, 54, 22, 100, -3, 5, 10, -33, 78, 90, 29, -45, 77, -9, 19, 21} Language: CPParrow_forward1. Shift Left k Cells Use Python Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forward
- Computer sciencearrow_forwardparam data * @return true if all items of the array are positive (more than 0), false otherwise. * return false if the array is null. */ public boolean allPositives(int[] data) without using functionsarrow_forwardC++ program This assignment is about array operations. Create an array with 1000 items and fill it with random numbers from the range of -100, 100 (including -100 and 100). Then, your program asks user to enter a number. The program keeps running until user enters a out-of-range (-100,100) number. An example run is given below. Enter a number: -4Frequency of -4: 54Enter a number: 4Frequency of 4: 15Enter a number: 35Frequency of 35: 8Enter a number: 43Frequency of 43: 2Enter a number: 101Bye...arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
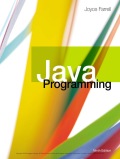
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
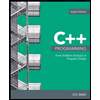
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
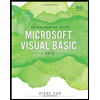
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage