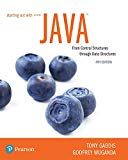
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 1PC
Program Plan Intro
Sorting Objects with the Insertion Sort
Program plan:
- Create the class “ObjectInsertionSorter”,
- Define the method “insertionSort()”,
- Declare the required variables.
- Execute “for” loop to iterate the elements in the array.
- Sort and insert the elements in the array.
- Create the class “ObjectInsertionTest”,
- Define the “main ()” function,
- Initialize the array values.
- Iterate “for” loop to print the original order of array elements.
- Iterate “for” loop to print the sorted order of array elements.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Recipe Program - Java ONLYI am looking to create a program that is a recipe holder. It needs to include at a minimum:
At least 1 loop
An Array or ArrayList
At least 3 Java classes
Use of methods
The program would allow the user to (as part of a menu selection):
Add a recipe
Include ingredient list
Instructions
List all recipes that are in the program (array)
Display a single recipe
Search Recipes (option to view recipe selected)
For example, searching for peanut butter cookie would bring up the recipe or multiple if the same name. The user could then select one of them to view the recipe.
Search Recipes with a single ingredient (option to view recipe selected)
For example: search for coconut and it would bring up coconut cream pie and German chocolate cake. The user could then select one of them to view the recipe.
Close application
Also need the program in a UML diagram.
Enhanced selection sort algorithm
SelectionSortDemo.java
package chapter7; /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue…
Movie.
Java Code
Chapter 16 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.2 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - Prob. 16.7CPCh. 16.2 - If a sequential search is performed on an array,...Ch. 16.3 - Prob. 16.9CPCh. 16.3 - Prob. 16.10CP
Ch. 16.3 - Prob. 16.11CPCh. 16.3 - Prob. 16.12CPCh. 16.3 - Prob. 16.13CPCh. 16.3 - Prob. 16.14CPCh. 16.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 16.3 - Prob. 16.16CPCh. 16.3 - Prob. 16.17CPCh. 16.3 - Prob. 16.18CPCh. 16 - Prob. 1MCCh. 16 - Prob. 2MCCh. 16 - Prob. 3MCCh. 16 - Prob. 4MCCh. 16 - Prob. 5MCCh. 16 - Prob. 6MCCh. 16 - Prob. 7MCCh. 16 - Prob. 8MCCh. 16 - Prob. 9MCCh. 16 - Prob. 10MCCh. 16 - True or False: If data is sorted in ascending...Ch. 16 - True or False: If data is sorted in descending...Ch. 16 - Prob. 13TFCh. 16 - Prob. 14TFCh. 16 - Assume this code is using the IntBinarySearcher...Ch. 16 - Prob. 1AWCh. 16 - Prob. 1SACh. 16 - Prob. 2SACh. 16 - Prob. 3SACh. 16 - Prob. 4SACh. 16 - Prob. 5SACh. 16 - Prob. 6SACh. 16 - Prob. 7SACh. 16 - Prob. 8SACh. 16 - Prob. 1PCCh. 16 - Sorting Objects with the Quicksort Algorithm The...Ch. 16 - Prob. 3PCCh. 16 - Charge Account Validation Create a class with a...Ch. 16 - Charge Account Validation Modification Modify the...Ch. 16 - Search Benchmarks Write an application that has an...Ch. 16 - Prob. 8PCCh. 16 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Bubble Sorting an Array of ObjectsCreate a Student class and you will need to do the following: Enter the number of students (must be greater than 5). Enter the student’s names and grades Make sure that you enter the names and grades in random order. Iterate through the array of students and using the bubble sort, order the array by grade.arrow_forwardEnhanced selection sort SelectionSortDemo.java package chapter7; /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue =…arrow_forward8. Grade Book A teacher has five students who have taken four tests. The teacher uses the following grading scale to assign a letter grade to a student, based on the average of his or her four test scores: Test Score Letter Grade 90–100 A 80-89 B 70-79 C 60-69 D 0-59 Write a class that uses a String array or an ArrayList object to hold the five students' names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles each to hold each student's set of test scores. The class should have methods that return a specific student's name, the average test score, and a letter grade based on the average. Demonstrate the class in a program that allows the user to enter each student's name and his or her four test scores. It should then display each student's average test score and letter grade. Input Validation: Do not accept test scores less than zero or greater than 100.arrow_forward
- Searching and sorting in Java programming: Write a method remove, that takes three parameters: an array of integers, the length of the array, and an integer, say removeItem. The method should find and delete the first occurrence of removeItem in the array. If the value does not exist or the array is empty, output an appropriate message. Note: after deleting the element, the array size is reduced by 1. You may assume that the array is unsorted. Thank you. I took Java in 2012 and 2013, and we didn't go too deep in this cocept..just a little bit in the Arrays chapter. i wanted to try something ne! Thank you!arrow_forwardRotate Right k cells (use python) Consider an array named source. Write a method/function named rotateRight( source, k) that rotates all the elements of the source array to the right by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] rotateRight(source,3) After calling rotateRight(source,3), printing the array should give the output as: [ 40, 50, 60, 10, 20, 30]arrow_forwardRooks on a rampage def safe_squares_rooks(n, rooks): A generalized n-by-n chessboard has been invaded by a parliament of rooks, each rook represented as a two-tuple (row, column) of the row and the column of the square that the rook is in. Since we are again computer programmers instead of chess players and other normal folks, our rows and columns are numbered from 0 to n - 1. A chess rook covers all squares that are in the same row or in the same column. Given the board size n and the list of rooks on that board, count the number of empty squares that are safe, that is, are not covered by any rook. To achieve this in reasonable time and memory, you should count separately how many rows and columns on the board are safe from any rook. Because permuting the rows and columns does not change the answer to this question, you can imagine all these safe rows and columns to have been permuted to form an empty rectangle at the top left corner of the board. The area of that safe rectangle is…arrow_forward
- 1. Shift Left k Cells Use Python Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forwardclass Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…arrow_forwarddont give the same solution again .10 min left fast Array Indexing: Knowing the basics of array indexing is important for analysing and manipulating the array object. NumPy offers many ways to do array indexing. O slicing: Just like lists in python, NumPy arrays can be sliced. As arrays can be multidimensional, you need to specify a slice for each dimension of the array. O Integer array indexing: In this method, lists are passed for indexing for each dimension. One to one mapping of corresponding elements is done to construct a new arbitrary array. O Boolean array indexing: This method is used when we want to pick elements from array which satisfy some conditionarrow_forward
- Fill in the blanksarrow_forwardsolve eith java:- Write code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age. Following is the class of Person: public class Person { private String Name; public int age; public void set(String Name, int age) {…arrow_forward1. An array is a container that holds a group of values of a 2. Each item in an array is called an 3. Each element is accessed by a numerical 4. The index to the first element of an array is 0 and the index to the last element of the array is the length of the 5. Given the following array declaration, determine which of the three statements below it are true. int [] autoMobile = new int [13]; i. autoMobile[0] is the reference to the first element in the array. ii. autoMobile[13] is the reference to the last element in the array. ii. There are 13 integers in the autoMobile array. 6. In java new is a which is used to allocate memory 7. Declare a one-dimensional array named score of type int that can hold9 values. 8. Declare and initialize a one-dimensional byte array named values of size 10 so that all entries contain 1. 9. Declare and initialize a one-dimensional array to store name of any 3 students. 10. Declare a two-dimensional array named mark of type double that can hold 5x3…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
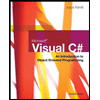
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,