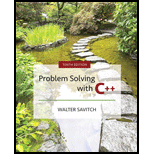
Give a definition for a class TitledEmployee that is a derived class of the base class SalariedEmployee given in Display 15.5. The class TitledEmployee has one additional member variable of type string called title. It also has two additional member functions: getTitle, which takes no arguments and returns a string; and setTitle, which is a void function that takes one argument of type string. It also redefines the member function setName. You do not need to give any implementations, just the class definition. However, do give all needed #include directives and all using namespace directives. Place the class TitledEmployee in the namespace employeessavitch.

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Artificial Intelligence: A Modern Approach
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Python (3rd Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Define a Pet class that stores the pet’s name, age, and weight. Add appropriateconstructors, accessor functions, and mutator functions. Also define a functionnamed getLifespan that returns a string with the value “unknown lifespan.” Next, define a Dog class that is derived from Pet. The Dog class should have aprivate member variable named breed that stores the breed of the dog. Add mutator and accessor functions for the breed variable and appropriate constructors.Redefine the getLifespan function to return “Approximately 7 years” if the dog’sweight is over 100 pounds and “Approximately 13 years” if the dog’s weight isunder 100 pounds. Next, define a Rock class that is derived from Pet. Redefine the getLifespanfunction to return “Thousands of years.” Finally, write a test program that creates instances of pet rocks and pet dogs thatexercise the inherited and redefined functions. use c++arrow_forwardwrite the definition of a class statistics containing: three data members x, y, and z of type integer. a member function called setValues that accepts three parameters and assigns them to x, y, and z the function returns no value. a member function called getSum that accepts no parameters and returns the sum of the member variables x, y, and z. a member function called getAvg that accepts no parameters and returns the average of the member variables x, y, and z (the average should be returnsed as a double value. to do this you can use a convert function or just divide by 3.0) a member function called getMin that accepts no parameters and returns the smallest member variable. a member function called getMax that accepts no parameters and returns the largest member variable.arrow_forwardCreate an Employee class that includes three private data members— firstName (type string), lastName (type string), and monthlySalary (type int ).It also includes several public member functions.1. A constructor initializes the three data members. 2. A setFirstName function accepts a string parameter and does not return any data. It sets the firstName.3. A getFirstName function does not accept any parameter and returns a string. It returns the firstName.4. A setLastName function accepts a string parameter and does not return any data. It sets the lastName.5. A getLastName function does not accept any parameter and returns a string. It returns the lastName.6. A setMonthlySalary function accepts an integer parameter and does not return any data. It sets the monthlySalary. If the monthly salary is less than or equal zero, set it to 1000 and it displays the employee’s first name, last name and the inputted salary with a statement “**==The salary is set to $1000.”7. A getMonthlySalary…arrow_forward
- PROGRAMMING LANGUAGE: C++ Write a program that has a base class named FlightCrew. This class shouldhave three data members: an integer to store the ID of the crew member, aninteger to store the number of years of service and another integer to storethe total salary of the member. Provide a parameterized constructor in theclass to set the values of the data members.Derive a class Pilot from FlightCrew to contain two additional data members,an integer to store the number of hours of flight and a boolean to storewhether the Pilot has military experience or not. Provide a paramete rizedconstructor in the Pilot class. Provide a function bonus() in the class wherethe bonus of a pilot is his number of flight hours times the 10% of his salary.Likewise, provide a function isEligible() in the class to find out if the pilot iseligible for promotion or not. A pilot is eligible for promotion to the nextrank if he has at least 5 years of experience and the number of total flighthours is greater…arrow_forwardProblem: Create a Fraction class with two private positive integer member variables numerator and denominator, one constructor with two integer parameters num and den with default values 0 and 1, one display function that print out a fraction in the format numerator/denominator in the proper form such as 2/3 or ½ . Note: 2/4 should be displayed as ½.arrow_forwardwrite the definition of the class Rectangle containing: length: a private data member of type double width: a private data member of type double a default constructor that assigns the values 20 and 10 length and width, respctively. an overloading constructor that accepts two parameters of type double and assigns them to length and width. an overloading constructor that accepts one parameter of type double and assigns it to both length and width setLength: a member function that accepts a double parameter and assigns it to length if it is positive(gerater than zero). setWidth: a member function that accepts a double parameter and assigns it to width if it is positive(gerater than 0) getLength: a member function that accepts no parameter and returns the value of length getWidth: a meber function that accepts no parameters and returns the value of width getAre: a member function that accepts no parameters and returns the area of the rectangle.arrow_forward
- Please solution in c++arrow_forwardWrite a class Box having three private data members (width, depth, height) The class has three constructors which are having no parameter – for setting values to zero or null. having three parameters for assigning values to height, width, depth respectively. Overload the above constructor and use this keyword to set the values of width, height & depth. Provide getters/setters for data members. Write a function calculateVolume() which calculates the volume of the box. Write test Application that demonstrates the Box class by calling all the three constructors and method, creating a Create Box object, and then displaying the Box’swidth , height, length and volume .arrow_forwardWrite a class named Patient that has member variables for first name, middle name, last name, phone number, name and phone number of emergency contact. The Patient class should have a constructor that accepts and argument for each member variable. The Patient class should also have accessor and mutator functions for each member variable. Write a class named Procedure that has member variables for name of procedure, date of procedure, name of physician, and charges for the procedure. This Procedure class represents a medical procedure that is performed on a patient. The Procedure class should have a constructor that accepts an argument for each member variable. The Procedure class should also have accessor and mutator functions for each member variable. Then write a program that creates an instance of the Patient class initialized with sample data that is entered by the user. Then, create an instance of the Procedure class for each procedure that is being…arrow_forward
- Write a class named Patient that has member variables for first name, middle name, last name, phone number, name and phone number of emergency contact. The Patient class should have a constructor that accepts and argument for each member variable. The Patient class should also have accessor and mutator functions for each member variable. Write a class named Procedure that has member variables for name of procedure, date of procedure, name of physician, and charges for the procedure. This Procedure class represents a medical procedure that is performed on a patient. The Procedure class should have a constructor that accepts an argument for each member variable. The Procedure class should also have accessor and mutator functions for each member variable. Then write a program that creates an instance of the Patient class initialized with sample data that is entered by the user. Then, create an instance of the Procedure class for each procedure that is being performed on the patient. There…arrow_forwardobjective of the project: Implement a class address. An address has a house number street optional apartment number city state postal code. All member variables should be private and the member functions should be public. Implement two constructors: one with an apartment number one without an appartment number. Implement a print function that prints the address with the street on one line and the city, state, and postal code on the next line. Implement a member function comesBefore that tests whether one address comes before another when the addresses are compared by postal code. Returns false if both zipcodes are equal. Use the provided main.cpp to start with. The code creates three instances of the Address class (three objects) to test your class. Each object will utilize a different constructor. You will need to add the class definition and implementation. The comesBefore function assumes one address comes before another based on zip code alone. The test will also return…arrow_forwardWrite a definition of a class named Point that might be used to store and manipulate the location of a point in the plane. You will need to declare and implement the following member functions:a. A member function set that sets the private data after an object of this class is created.b. A member function to move the point by an amount along the vertical and horizontal directions specified by the first and second arguments.c. A member function to rotate the point by 90 degrees clockwise around the origin.d. Two const inspector functions to retrieve the current coordinates of the point.Document these functions with appropriate comments. Embed your class in a test program that requests data for several points from the user, creates the points, then exercises the member functions.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
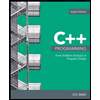
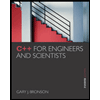
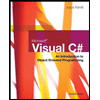