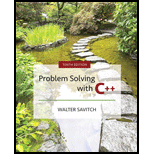
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 15.1, Problem 2STE
Explanation of Solution
Program:
//include library
#include<iostream>
using namespace std;
//class definition
class SmartBut : public Smart
{
//access specifier
public:
// declaration of constructors
SmartBut( );
SmartBut(int newA, int newB, bool newCrazy);
bool isCrazy( ) const;
//access...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2. Consider the following pseudocode for partition:
function partition (A,L,R)
pivotkey = A [R]
t = L
for i
L to R-1 inclusive:
if A[i] A[i]
t = t + 1
end if
end for
A [t] A[R]
return t
end function
Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the
indicated instances.
Initial A
After i=0 ends
After 1 ends
After i 2 ends
After i = 3 ends
After i = 4 ends
After final swap
10 19 285
[12 pts]
.NET Interactive
Solving Sudoku using Grover's Algorithm
We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules:
•No column may contain the same value twice
•No row may contain the same value twice
If we assign each square in our sudoku to a variable like so:
1
V V₁
V3
V2
we want our circuit to output a solution to this sudoku.
Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the
conversion of classical decision problems into oracles for Grover's algorithm.
Turning the Problem into a Circuit
We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit
that…
using r language
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Ch. 15.1 - Is the following program legal (assuming...Ch. 15.1 - Prob. 2STECh. 15.1 - Is the following a legal definition of the member...Ch. 15.1 - The class SalariedEmployee inherits both of the...Ch. 15.1 - Give a definition for a class TitledEmployee that...Ch. 15.1 - Give the definitions of the constructors for the...Ch. 15.2 - You know that an overloaded assignment operator...Ch. 15.2 - Suppose Child is a class derived from the class...Ch. 15.2 - Give the definitions for the member function...Ch. 15.2 - Define a class called PartFilledArrayWMax that is...
Ch. 15.3 - Prob. 11STECh. 15.3 - Why cant we assign a base class object to a...Ch. 15.3 - What is the problem with the (legal) assignment of...Ch. 15.3 - Suppose the base class and the derived class each...Ch. 15 - Write a program that uses the class...Ch. 15 - Listed below are definitions of two classes that...Ch. 15 - Solution to Programming Project 15.1 Give the...Ch. 15 - Create a base class called Vehicle that has the...Ch. 15 - Define a Car class that is derived from the...Ch. 15 - Prob. 4PPCh. 15 - Consider a graphics system that has classes for...Ch. 15 - Flesh out Programming Project 5. Give new...Ch. 15 - Banks have many different types of accounts, often...Ch. 15 - Radio Frequency IDentification (RFID) chips are...Ch. 15 - The goal for this Programming Project is to create...Ch. 15 - Solution to Programming Project 15.10 Listed below...Ch. 15 - The computer player in Programming Project 10 does...Ch. 15 - Prob. 12PP
Knowledge Booster
Similar questions
- 8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forward
- Find the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forwardTask 2: Comparable Interface and Record (10 Points) 1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record should include the dog's name, breed, age, and weight. You are required to implement the Comparable interface for the Dog record so that you can sort the records based on the dogs' ages. Create a Java record named Dog.java. name: String breed: String age: int weight: double + toString(): String > Dog + compareTo(otherDog: Dog): int > Comparable 2. In the Dog record, establish a main method and proceed to generate an array named dogList containing three Dog objects, each with the following attributes: Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5 Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30 Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22 3. Print the dogs in dogList before sorting the dogList by age. (Please check the example output for the format). • 4. Sort the dogList using…arrow_forwardThe OSI (Open Systems Interconnection) model is a conceptual framework that standardises the functions of a telecommunication or computing system into seven distinct layers, facilitating communication and interoperability between diverse network protocols and technologies. Discuss the OSI model's physical layer specifications when designing the physical network infrastructure for a new office.arrow_forward
- In a network, information about how to reach other IP networks or hosts is stored in a device's routing table. Each entry in the routing table provides a specific path to a destination, enabling the router to forward data efficiently across the network. The routing table contains key parameters determining the available routes and how traffic is directed toward its destination. Briefly explain the main parameters that define a routing entry.arrow_forwardYou are troubleshooting a network issue where an employee's computer cannot connect to the corporate network. The computer is connected to the network via an Ethernet cable that runs to a switch. Suspecting a possible layer 1 or layer 2 problem, you decide to check the LED status indicators on both the computer's NIC and the corresponding port on the switch. Describe five LED link states and discuss what each indicates to you as a network technician.arrow_forwardYou are a network expert tasked with upgrading the network infrastructure for a growing company expanding its operations across multiple floors. The new network setup needs to support increased traffic and future scalability and provide flexibility for network management. The company is looking to implement Ethernet switches to connect various devices, including workstations, printers, and IP cameras. As part of your task, you must select the appropriate types of Ethernet switches to meet the company's needs. Evaluate the general Ethernet switch categories you would consider for this project, including their features and how they differ.arrow_forward
- You are managing a Small Office Home Office (SOHO) network connected to the Internet via a fibre link provided by your Internet Service Provider (ISP). Recently, you have noticed a significant decrease in Internet speed, and after contacting your ISP, they confirmed that no issues exist on their end. Considering that the problem may lie within your local setup, identify three potential causes of the slow Internet connection, focusing on physical factors affecting the fibre equipment.arrow_forwardYour organisation has recently installed a new network in a building it has acquired. As the network administrator, you have set up a dedicated telecommunications room to house all the rack-mounted servers, switches, and routers. To ensure optimal performance and longevity of the equipment, you need to monitor certain environmental factors in the room. Identify the environmental factors that should be monitored and explain why each is important to maintain the proper functioning of the telecommunications equipment.arrow_forwardYour organisation is preparing to move into a newly constructed office building that has never been occupied or wired for network services. As the network administrator, your manager has tasked you with designing a structured cabling plan that will support data, voice, and other network services across all building floors. The cabling plan must account for future expansion, efficient data transmission, and compliance with industry standards. Identify and explain the different subsystems you would include in the structured cabling scheme, following the ANSI/TIA/EIA 568 standard.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
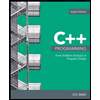
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
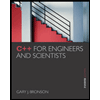
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
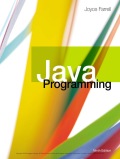
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
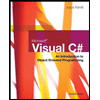
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
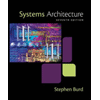
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning