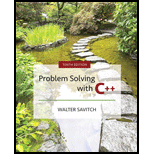
Concept explainers
Listed below are definitions of two classes that use inheritance, code for their implementation, and a main function. Put the code into appropriate files with the necessary include statements and preprocessor statements so that the program compiles and runs. It should output “Circle has radius 2 and area 12.5664”.
class Shape { public: Shape(); Shape(string name); string getName(); void setName(string newName); virtual double getArea() = 0; private: string name; }; Shape::Shape() { name=""; } Shape::Shape(string name) { this->name = name; } string Shape::getName() { return this->name; } void Shape::setName(string newName) { this->name = newName; } class Circle : public Shape { public: Circle(); Circle(int theRadius); void setRadius(int newRadius); double getRadius(); virtual double getArea(); private: int radius; }; Circle::Circle() : Shape("Circle"), radius(0) { } Circle::Circle(int theRadius) : Shape("Circle"), radius(theRadius) { } void Circle::setRadius(int newRadius) { this->radius = newRadius; } double Circle::getRadius() { return radius; } double Circle::getArea() { return 3.14159 * radius * radius; } int main() { Circle c(2); cout << c.getName() << " has radius " << c.getRadius() << " and area " << c.getArea() << endl; return 0; } |
Add another class, Rectangle, that is also derived from the Shape class. Modify the Rectangle class appropriately so it has private width and height variables, a constructor that allows the user to set the width and height, functions to retrieve the width and height, and an appropriately defined getArea function that calculates the area of the rectangle.
The following code added to main should output “Rectangle has width 3 has height 4 and area 12.0”.
Rectangle r(3,4); cout << r.getName() << " has width " << r.getWidth() << " has height " << r.getHeight() << " and area " << r.getArea() << endl; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Starting Out With Visual Basic (8th Edition)
Degarmo's Materials And Processes In Manufacturing
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Web Development and Design Foundations with HTML5 (8th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- Modern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forward
- (A) A cellular system has 12 microcells with 10 channels per cell. The microcells are split into 3 microcells, and each microcell is further split into 4 picocells. Determine the number of channels available in system after splitting into picocelles.arrow_forwardQuestion 8 (10 points) Produce a relational schema diagram that corresponds to the following ER diagram for a Vacation Property Rentals database. Your relational schema diagram should include primary & foreign keys. Upload your relational schema diagram as a PDF document. Don't forget that the relation schemas for "Beach Property" and "Mountain Property" should each have primary keys. FYI: "d" in this notation denotes a subclass. Figure 2: ER Diagram for Question 8 id first RENTER name middle last address phone email 1 signs N id begin date RENTAL AGREEMENT end date amount N street address books city id 1 state address num. rooms PROPERTY zip code base rate type propertyType blocks to beach activity "B" "M" BEACH PROPERTY MOUNTAIN PROPERTYarrow_forwardNotes: 1) Answer All Question, 2) 25 points for each question QI Figurel shows the creation of the Frequency Reuse Pattern Using the Cluster Size K: (A) illustrates how i and j can be used to locate a co-channel cell. huster 3 Cluster Cluster 2 X=7(i=2,j1)arrow_forward
- You are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following taking the database offline is not allowed since people are connected to it and how personal data might be bridged and not secured.Provide three references with you answer.arrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answer from websitesarrow_forward
- Modern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardObjective: 1. Implement a custom Vector class in C++ that manages dynamic memory efficiently. 2. Demonstrate an understanding of the Big Five by managing deep copies, move semantics, and resource cleanup. 3. Explore the performance trade-offs between heap and stack allocation. Task Description: Part 1: Custom Vector Implementation 1. Create a Vector class that manages a dynamically allocated array. 。 Member Variables: ° T✶ data; // Dynamically allocated array for storage. std::size_t size; // Number of elements currently in the vector. std::size_t capacity; // Maximum number of elements before reallocation is required. 2. Implement the following core member functions: Default Constructor: Initialize an empty vector with no allocated storage. 。 Destructor: Free any dynamically allocated memory. 。 Copy Constructor: Perform a deep copy of the data array. 。 Copy Assignment Operator: Free existing resources and perform a deep copy. Move Constructor: Transfer ownership of the data array…arrow_forward2.68♦♦ Write code for a function with the following prototype: * Mask with least signficant n bits set to 1 * Examples: n = 6 -> 0x3F, n = 17-> 0x1FFFF * Assume 1 <= n <= w int lower_one_mask (int n); Your function should follow the bit-level integer coding rules Be careful of the case n = W.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
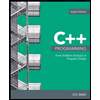
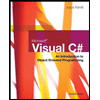
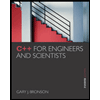
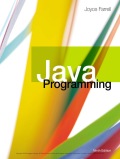
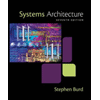