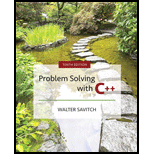
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 15, Problem 4PP
Program Plan Intro
Creation of program to create and display billing records for patients
Program Plan:
- Define a class “person” to define methods and operations in it.
- Define a constructor “person()” to create new instance of a person.
- Define a method “getName()” to get name of person.
- Define a method “operator=()” to define operation for “=” operator.
- Define a method “operator>>()” to define operation for “>>” operator.
- Define a method “operator<<()” to define operation for “<<” operator.
- Define a class “doctor” to define methods and operations in it.
- Define a constructor “doctor()” to create new instance of a doctor.
- Define a method “gtDctr()” to get name of doctor.
- Define a method “gtDsgntn()” to get designation of doctor.
- Define a method “operator=()” to define operation for “=” operator.
- Define a class “patient” to define methods and operations in it.
- Define a constructor “patient()” to create new instance of a patient.
- Define a method “getPtnt()” to get name of patient.
- Define a method “gtDctr()” to get name of doctor.
- Define a method “gtDsgntn()” to get designation of doctor.
- Define a method “operator=()” to define operation for “=” operator.
- Define a class “billing” to define methods and operations in it.
- Define a constructor “billingt()” to create new instance of billing.
- Define a method “getPtnt()” to get name of patient.
- Define a method “gtDctr()” to get name of doctor.
- Define a method “gtDsgntn()” to get designation of doctor.
- Define a method “gtBill()” to get bill for patient.
- Define a method “operator=()” to define operation for “=” operator.
- Define a main method
- Create instance for “doctor”, “patient” and “billing” classes.
- Call methods in classes to perform specific operations.
- Display the result.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
What is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).
What are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?
3. Problem Description: Define the Circle2D class that contains:
Two double data fields named x and y that specify the center of the circle with get
methods.
• A data field radius with a get method.
•
A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius.
•
A constructor that creates a circle with the specified x, y, and radius.
•
A method getArea() that returns the area of the circle.
•
A method getPerimeter() that returns the perimeter of the circle.
•
•
•
A method contains(double x, double y) that returns true if the specified point (x, y) is inside
this circle. See Figure (a).
A method contains(Circle2D circle) that returns true if the specified circle is inside this
circle. See Figure (b).
A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this
circle. See the figure below.
р
O
со
(a)
(b)
(c)<
Figure (a) A point is inside the circle. (b) A circle is inside another circle.
(c) A circle overlaps another…
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Ch. 15.1 - Is the following program legal (assuming...Ch. 15.1 - Prob. 2STECh. 15.1 - Is the following a legal definition of the member...Ch. 15.1 - The class SalariedEmployee inherits both of the...Ch. 15.1 - Give a definition for a class TitledEmployee that...Ch. 15.1 - Give the definitions of the constructors for the...Ch. 15.2 - You know that an overloaded assignment operator...Ch. 15.2 - Suppose Child is a class derived from the class...Ch. 15.2 - Give the definitions for the member function...Ch. 15.2 - Define a class called PartFilledArrayWMax that is...
Ch. 15.3 - Prob. 11STECh. 15.3 - Why cant we assign a base class object to a...Ch. 15.3 - What is the problem with the (legal) assignment of...Ch. 15.3 - Suppose the base class and the derived class each...Ch. 15 - Write a program that uses the class...Ch. 15 - Listed below are definitions of two classes that...Ch. 15 - Solution to Programming Project 15.1 Give the...Ch. 15 - Create a base class called Vehicle that has the...Ch. 15 - Define a Car class that is derived from the...Ch. 15 - Prob. 4PPCh. 15 - Consider a graphics system that has classes for...Ch. 15 - Flesh out Programming Project 5. Give new...Ch. 15 - Banks have many different types of accounts, often...Ch. 15 - Radio Frequency IDentification (RFID) chips are...Ch. 15 - The goal for this Programming Project is to create...Ch. 15 - Solution to Programming Project 15.10 Listed below...Ch. 15 - The computer player in Programming Project 10 does...Ch. 15 - Prob. 12PP
Knowledge Booster
Similar questions
- 1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forwardPlease include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forward
- Object-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forwardWhen using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forward
- Find the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forwardFind the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forward
- Please show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forwardGo to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
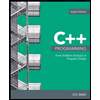
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
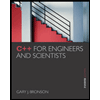
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
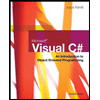
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
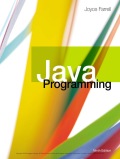
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
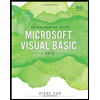
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning