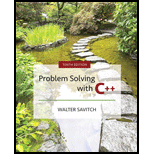
Solution to Programming Project 15.10
Listed below is code to play a guessing game. In the game two players
attempt to guess a number. Your task is to extend the program with objects that represent either a human player or a computer player. The rand() function requires you include cstdlib (see Appendix 4):
bool checkForWin(int guess, int answer) { cout<< "You guessed" << guess << "."; if (answer == guess) { cout<< "You're right! You win!" <<endl; return true; } else if (answer < guess) cout<< "Your guess is too high." <<endl; else cout<< "Your guess is too low." <<endl; return false; } void play(Player &player1, Player &player2) { int answer = 0, guess = 0; answer = rand() % 100; bool win = false; while (!win) { cout<< "Player 1's turn to guess." <<endl; guess = player1.getGuess(); win = checkForWin(guess, answer); if (win) return; cout<< "Player 2's turn to guess." <<endl; guess = player2.getGuess(); win = checkForWin(guess, answer); } } |
The play function takes as input two Player objects. Define the Player class with a virtual function named getGuess(). The implementation of Player::getGuess() can simply return 0. Next, define a class named HumanPlayer derived from Player. The implementation of HumanPlayer::getGuess() should prompt the user to enter a number and return the value entered from the keyboard. Next, define a class named ComputerPlayer derived from Player. The implementation of ComputerPlayer::getGuess() should randomly select a number between 0and 99 (see Appendix 4 for information on random number generation).Finally, construct a main function that invokes play(Player &player1, Player &player2) with two instances of a HumanPlayer (human versus human), an instance of a HumanPlayer and Computer Player (human versus computer),and two instances of ComputerPlayer (computer versus computer).

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
C++ How to Program (10th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++: Early Objects
Artificial Intelligence: A Modern Approach
- In C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none. Show how this function is being called and returning proper values. you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.arrow_forwardC++ Create a Blackjack (21) game. Your version of the game will imagine only a SINGLE suit of cards, so 13 unique cards, {2,3,4,5,6,7,8,9,10,J,Q,K,A}. Upon starting, you will be given two cards from the set, non-repeating. Your program MUST then tell you the odds of receiving a beneficial card (that would put your value at 21 or less), and the odds of receiving a detrimental card (that would put your value over 21). Recall that the J, Q, and K cards are worth ‘10’ points, the A card can be worth either ‘1’ or ‘11’ points, and the other cards are worth their numerical values. FOR YOUR ASSIGNMENT: Provide two screenshots, one in which the game suggests it’s a good idea to get an extra card and the result, and one in which the game suggests it’s a bad idea to get an extra card, and the result of taking that extra card.arrow_forwarddo in javaarrow_forward
- Give solution in C ++ Language with secreenshoot of source code. Part 01In this task, you need to do the following:• Write a function named displayMessage() that takes user name as input in character array and then shows greetings• Now take the name input in main() and pass the name as an argument to displayMessage() function• Change the displayMessage() method such that it returns the number of characters after displaying the greetings part 02Write a function power that takes two parameters a and b. And it returns the power as ab.arrow_forward3: The code on the right is supposed to be the ARM code for procA; however, there are problems with the ARM code. C code: int procA(int x, int y) { int perimeter = (x + y) * 2; I return perimeter; } ARM code: I procA: add rø, r1, r2 mov r1, rø lsl #1 bx lr push {lr} Give the corrected version of the ARM code for procA:arrow_forwardCode in python: A certain company has encoded the accounts of its customers and requires that you provide an algorithm that, given an account code, informs if it is valid according to the following description: The account codes are made up of 4 digits counted from right to left, plus the verification digit. The verifying digit is obtained by adding the digits of the account number of the even positions and multiplying the digits of the odd positions, from the new result the residue of the division is extracted for 10, which represents the verifying digit. Develop the code in python as a check digit class that returns 1 if it is correct or 0 if not Heading: Extract digits, verifier calculation, Account verification, Integral algorithmarrow_forward
- Write in C++ Alice is trying to monitor how much time she spends studying per week. She going through her logs, and wants to figure out which week she studied the least, her total time spent studying, and her average time spent studying per week. To help Alice work towards this goal, write three functions: min(), total(), and average(). All three functions take two parameters: an array of doubles and the number of elements in the array. Then, they make the following computations: min() - returns the minimum value in the array sum() - returns the sum of all the values in the array average() - returns the average of all the values in the array You may assume that the array will be non-empty. Function specifications: Function 1: Finding the minimum hours studied Name: min() Parameters (Your function should accept these parameters IN THIS ORDER): arr double: The input array containing Alice's study hours per week arr_size int: The number of elements stored in the array Return Value:…arrow_forwardX15: inOrder Write a function in Java that implements the following logic: Given three ints, a, b , and c,return true if b is greater than a, and c is greater than b.However, with the exception that if bok is true, b does not need to be greater than a.arrow_forwardCode Should Be In C++arrow_forward
- MindTap: In C#, Write a program named Averages that includes a method named Average that accepts any number of numeric parameters, displays them, and displays their average. Test your function in your Main(). Tests will be run against Average() to determine that it works correctly when passed one, two, or three numbers, or an array of numbers.arrow_forwardPrigraming in Python Problem 6.3 Purpose: Write array functions to solve a mathematical problem. I'm sure you have all heard of Euclidean distance. In two dimensions, the Euclidean distance between (x1, x2) and (y1, y2) is the square root of (x1-y1)2 + (x2-y2)2, or ((x1-y1)2 + (x2-y2)2)1/2. For example, the distance between (3,1) and (1,1) is ((3-1)2+(1-1)2)1/2 = 2 and the Euclidean distance between (1,1) and (0,0) is ((1-0)2+(1-0)2)1/2 ~= 1.4142. The Euclidean norm of a vector is just its Euclidean distance to the origin, (0,0). Therefore, the Euclidean norm of (1,1) is ~= 1.4142. This notion of Euclidean norm can easily be extended to vectors of arbitrary dimension. Indeed, if x is a vector of dimension n (versus dimension 2), then its Euclidean norm, which we will denote by |x|2, is given by |x|2 = ( Σ i = 1 to n |xi|2 )1/2 = (|x1|2+|x2|2+ ... + |xn|2 )1/2 There, Σ is a shorthand for "sum," as we saw in lecture and |xi| is just the absolute value of the real number xi. By the way,…arrow_forwardIn C#, Write a program named Averages that includes a method named Average that accepts any number of numeric parameters, displays them, and displays their average. Test your function in your Main(). Tests will be run against Average() to determine that it works correctly when passed one, two, or three numbers, or an array of numbers.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
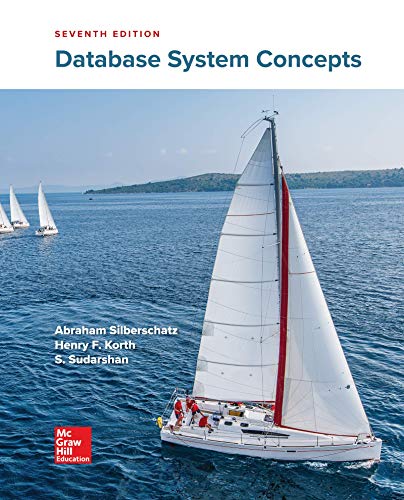
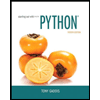
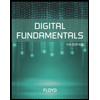
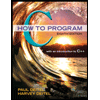
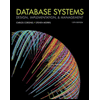
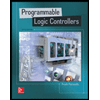