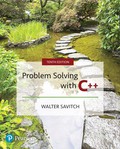
Solution to Programming Project 15.10
Listed below is code to play a guessing game. In the game two players
attempt to guess a number. Your task is to extend the program with objects that represent either a human player or a computer player. The rand() function requires you include cstdlib (see Appendix 4):
bool checkForWin(int guess, int answer) { cout<< "You guessed" << guess << "."; if (answer == guess) { cout<< "You're right! You win!" <<endl; return true; } else if (answer < guess) cout<< "Your guess is too high." <<endl; else cout<< "Your guess is too low." <<endl; return false; } void play(Player &player1, Player &player2) { int answer = 0, guess = 0; answer = rand() % 100; bool win = false; while (!win) { cout<< "Player 1's turn to guess." <<endl; guess = player1.getGuess(); win = checkForWin(guess, answer); if (win) return; cout<< "Player 2's turn to guess." <<endl; guess = player2.getGuess(); win = checkForWin(guess, answer); } } |
The play function takes as input two Player objects. Define the Player class with a virtual function named getGuess(). The implementation of Player::getGuess() can simply return 0. Next, define a class named HumanPlayer derived from Player. The implementation of HumanPlayer::getGuess() should prompt the user to enter a number and return the value entered from the keyboard. Next, define a class named ComputerPlayer derived from Player. The implementation of ComputerPlayer::getGuess() should randomly select a number between 0and 99 (see Appendix 4 for information on random number generation).Finally, construct a main function that invokes play(Player &player1, Player &player2) with two instances of a HumanPlayer (human versus human), an instance of a HumanPlayer and Computer Player (human versus computer),and two instances of ComputerPlayer (computer versus computer).

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- You are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following taking the database offline is not allowed since people are connected to it and how personal data might be bridged and not secured.Provide three references with you answer.arrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forwardYou are called by your supervisor to go and check a potential data bridge problem. What are the stepsyou will follow in order to check the database and fix any problems with it? Have in mind that youSHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answer from websitesarrow_forward
- Modern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardObjective: 1. Implement a custom Vector class in C++ that manages dynamic memory efficiently. 2. Demonstrate an understanding of the Big Five by managing deep copies, move semantics, and resource cleanup. 3. Explore the performance trade-offs between heap and stack allocation. Task Description: Part 1: Custom Vector Implementation 1. Create a Vector class that manages a dynamically allocated array. 。 Member Variables: ° T✶ data; // Dynamically allocated array for storage. std::size_t size; // Number of elements currently in the vector. std::size_t capacity; // Maximum number of elements before reallocation is required. 2. Implement the following core member functions: Default Constructor: Initialize an empty vector with no allocated storage. 。 Destructor: Free any dynamically allocated memory. 。 Copy Constructor: Perform a deep copy of the data array. 。 Copy Assignment Operator: Free existing resources and perform a deep copy. Move Constructor: Transfer ownership of the data array…arrow_forward2.68♦♦ Write code for a function with the following prototype: * Mask with least signficant n bits set to 1 * Examples: n = 6 -> 0x3F, n = 17-> 0x1FFFF * Assume 1 <= n <= w int lower_one_mask (int n); Your function should follow the bit-level integer coding rules Be careful of the case n = W.arrow_forward
- Hi-Volt Components You are the IT manager at Hi-Voltage Components, a medium-sized firm that makes specialized circuit boards. Hi-Voltage's largest customer, Green Industries, recently installed a computerized purchasing sys- tem. If Hi-Voltage connects to the purchasing system, Green Industries will be able to submit purchase orders electronically. Although Hi-Voltage has a computerized accounting system, that system is not capable of handling EDI. Tasks 1. What options does Hi-Voltage have for developing a system to connect with Green Industries' pur- chasing system? 2. What terms or concepts describe the proposed computer-to-computer relationship between Hi-Voltage and Green Industries? why not? 3. Would Hi-Voltage's proposed new system be a transaction processing system? Why or 4. Before Hi-Voltage makes a final decision, should the company consider an ERP system? Why or why not?arrow_forwardConsider the following expression in C: a/b > 0 && b/a > 0.What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answerarrow_forwardConsider the following expression in C: a/b > 0 && b/a > 0. What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answer.arrow_forward
- What are the major threats of using the internet? How do you use it? How do children use it? How canwe secure it? Provide four references with your answer. Two of the refernces can be from an article and the other two from websites.arrow_forwardAssume that a string of name & surname is saved in S. The alphabetical characters in S can be in lowercase and/or uppercase letters. Name and surname are assumed to be separated by a space character and the string ends with a full stop "." character. Write an assembly language program that will copy the name to NAME in lowercase and the surname to SNAME in uppercase letters. Assume that name and/or surname cannot exceed 20 characters. The program should be general and work with every possible string with name & surname. However, you can consider the data segment definition given below in your program. .DATA S DB 'Mahmoud Obaid." NAME DB 20 DUP(?) SNAME DB 20 DUP(?) Hint: Uppercase characters are ordered between 'A' (41H) and 'Z' (5AH) and lowercase characters are ordered between 'a' (61H) and 'z' (7AH) in the in the ASCII Code table. For lowercase letters, bit 5 (d5) of the ASCII code is 1 where for uppercase letters it is 0. For example, Letter 'h' Binary ASCII 01101000 68H 'H'…arrow_forwardWhat did you find most interesting or surprising about the scientist Lavoiser?arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
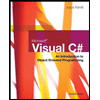
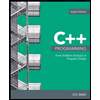
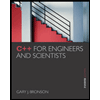
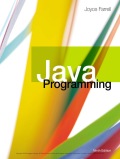