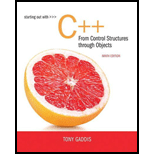
Concept explainers
Trivia Game
In this
The program will work like this:
• Starting with player 1, each player gets a turn at answering five trivia questions. (There are a total of 10 questions.) When a question is displayed, four possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point.
• After answers have been selected for all of the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner.
In this program, you will design a Question class to hold the data for a trivia question.
The Question class should have member variables for the following data:
• A trivia question
• Possible answer #1
• Possible answer #2
• Possible answer #3
• Possible answer #4
• The number of the correct answer (1, 2, 3, or 4)
The Question class should have appropriate constructor(s), accessor, and mutator functions.
The program should create an array of 10 Question objects, one for each trivia question. Make up your own trivia questions on the subject or subjects of your choice for the objects.

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Database Concepts (8th Edition)
Degarmo's Materials And Processes In Manufacturing
- https://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forwardWhy do we use NAT and PAT technologies? What happens without them? What is the major difference between NAT and PAT (Port Address Translation)? please answer it in the simplest way as possiblearrow_forwardWhat is the difference between physical connection (Physical topology) and logical connection (Logical topology)? Why are both necessary? Why do we need the Seven-Layer OSI model? What will happen If we don’t have it? Why do we need official standards for copper cable and fiber-optic cable? What happens without the standard? please answer in the simplest way as possiblearrow_forward
- Suppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardSuppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank(T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardhttps://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forward
- Highlight the main differences between Computer Assisted Coding and Alone Coding with their similaritiesarrow_forwardSuppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap ( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardSuppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank (T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forward
- Suppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank (T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardautomata theoryarrow_forwardI need help in construct a matlab code to find the voltage, the currents, and the watts based on that circuit.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
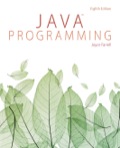
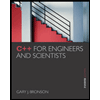
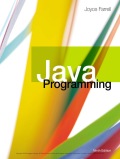
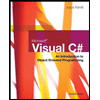
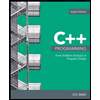