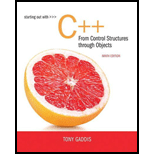
Concept explainers
What is the difference between a class and an instance of the class?

Class:
In object oriented programming, the concept of class is considered as an important feature.
- A class is a collection of data members and member functions, which are used to represent the overall structure of the project.
- The objects are created to access the data members and its functions of the class.
Explanation of Solution
Difference between class and instance of the class:
Some of the differences between class and instance of the class are as follows:
Class | Instance of the class |
A class is like a blueprint from which the object is created. | Instance of a class is called as an object. |
When a class is created, its memory space is not allocated. | After the creation of an instance for the class, memory space is allocated. |
Class is a logical entity. | Instance of the class is a physical entity. |
The definition for a class can be created only once. | The object can be created as many times as possible. |
Syntax: class Class_name { //class members } |
Syntax: Class_name object; |
Example Program for class and instance of a class:
//Include the required header files
#include<iostream>
#include<string>
using namespace std;
//class name
class Mobile
{
//Access specifier
public:
//Declare a variable
string mobile_name;
};
//Main Function
int main()
{
//create a first object for mobile
Mobile object1;
//Assign a string to the object
object1.mobile_name = "Samsung";
//print the name
cout << object1.mobile_name << endl;
//create a second object for mobile
Mobile object2;
//Assign a string to the object
object2.mobile_name = "Apple";
//print the name
cout << object2.mobile_name << endl;
}
Explanation:
- In the above program, inside a class “Mobile”, declare a variable named “mobile_name”.
- Inside a main function
- Create an object named “object1”.
- Access and assign a string “Samsung” to the data member.
- Print the string.
- Create an object named “object2”.
- Access and assign a string “Apple” to the data member.
- Print the string.
Samsung
Apple
Want to see more full solutions like this?
Chapter 13 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Software Engineering (10th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
- Describe the difference between making a class a member of another class (object aggregation), and making a class a friend of another class.arrow_forwardWhat is the difference between a class's static data member and a non-static data member?Give an example of why a static data member would be useful in the real world.arrow_forwardSo why is it important for a class to have its own destructor?arrow_forward
- is the term used to describe the process through which a member of one class becomes a member of another.arrow_forwardSo, what are "static members" of a class, exactly? When and why should you take use of them?arrow_forwardDistinguish between implementing an interface and implementing a derived class.arrow_forward
- The pillar that allows you to build a specialized version of a general class, but violates encapsulation principles isarrow_forwardis the word for the process of converting a member of one class into a member of another.arrow_forwardis the term used to describe the process of transforming a member of one class into a member of another.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
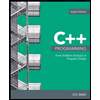