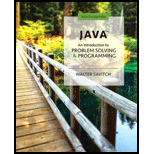
Concept explainers
Explanation of Solution
Given code:
The given code fragment is highlighted.
Filename: “ListNode.java”
//Define "ListNode" class
public class ListNode
{
/* Refer the textbook of Listing 12.4 */
}
Filename: “StringLinkedList.java”
//Define "StringLinkedList" class
public class StringLinkedList
{
/* Refer the textbook of Listing 12.5 */
}
Filename: “StringLinkedListDemo.java”
//Define "StringLinkedListDemo" class
public class StringLinkedListDemo
{
//Define main function
public static void main(String[] args)
{
/* Create object "list" from "StringLinkedList" class */
StringLinkedList list = new StringLinkedList();
/* Add value to node using "addANodeToStart" method */
list.addANodeToStart("A");
list.addANodeToStart("B");
list.addANodeToStart("C");
/* Call the method "showList()" to display the element on the list */
list.showList();
}
}
Reasons for displaying given output:
From the given code,
- The first line “StringLinkedList list = new StringLinkedList();” is used to Create object “list” from “StringLinkedList” class...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- According to the text's specifications, a collection is a list. True Falsearrow_forwardLab Goal : This lab was designed to teach you more about list processing and algorithms.Lab Description : Write a program that will search through a list to find the smallest number and the largest number. The program will return the average the largest and smallest numbers. You must combine variables, ifs, and a loop to create a working method. There will always be at least one item in the list.arrow_forwardTurn this into a flowchart/pseudo code. //This is where the functions go void insertfront(int data);void insert(int data);void display();void deletedata(int data);void reverselist();void searchdata(int data);void swap();void datasort();void deleteList(); #include <iostream>#include <stdlib.h>using namespace std; struct Node {int data;struct Node *next;}; struct Node* head = NULL;struct Node* rhead = NULL;int count1; //insert in front for reversedlistvoid insertfront(int data){Node* new_node = (Node*) malloc(sizeof(Node)); new_node->data = data; new_node->next = rhead; rhead=new_node;}//insert at endvoid insert(int new_data){Node* ptr;ptr = head;Node* new_node = (Node*) malloc(sizeof(Node)); new_node->data = new_data; new_node->next = NULL;if (head == NULL) { head = new_node; } else{while (ptr->next!= NULL) { ptr = ptr->next;}ptr->next=new_node;}count1++;}//display listvoid display() {struct Node* ptr;ptr = head;if(head==NULL){cout<<"Sorry the list…arrow_forward
- ID: A Name: Multiple Response Identify one or more choices that best complete the statement or answer the question. 10. The following code segment is intended to remove all duplicate elements in the list myList. The procedure does not work as intended. jt LENGTH(myList) REPEAT UNTIL(j = 1) %3D } IF(myList[j] = myList[j - 1]) %3D } REMOVE (myList, j) { j+j-1 { For which of the following contents of myList will the procedure NOT produce the intended results? a. [30, 30, 30, 10, 20, 20] b. [10, 10, 20, 20, 10, 10] c. [50, 50, 50, 50, 50, 50] d. [30, 50, 40, 10, 20, 40]arrow_forward#Hard-Coded board1_list = [['*','*','*'],['*','+','*'],['*','*','*']]print_board(board1_list)print() #just for a spacer lineboard2_list = [['*',' ',' ','*'], ['*',' ',' ','*'], ['*',' ',' ','*'], ['*',' ',' ','*']]print_board(board2_list)print() #just for a spacer lineboard3_list = [['*',' ','*',' ','*'], [' ','*',' ','*',' '], [' ',' ',' ',' ',' '], [' ','+',' ','+',' '], ['+',' ','+',' ','+']]print_board(board3_list)arrow_forward| Which data structure to use? Suppose you need to store a list of elements, if the number of elements in the program is fixed, what data structure should you use? (array, ArrayList, or LinkedList) If you have to add or delete the elements at the beginning of a list, should you use ArrayList or LinkedList? If most of operations on a list involve retrieving an element at a given index, should you use ArrayList or LinkedList?arrow_forward
- If you have downloaded the source code from this book’s companion Web site, you will find a file named USPopulation.txt in the Chapter 07 folder. The file contains the midyear population of the United States, in thousands, during the years 1950 through 1990. The first line in the file contains the population for 1950, the second line contains the population for 1951, and so forth.Write a program that reads the file’s contents into a list. The program should display the following data:• The average annual change in population during the time period• The year with the greatest increase in population during the time period• The year with the smallest increase in population during the time period(You can access the book’s companion Web site at www.pearsonglobaleditions.com/gaddis.)arrow_forwardC# Gas Station Program: Write a program that allows the users to select items that are for sale in a gas station. Candy, Soda, Pizza, Muffins and the list goes on. Keep a running total of the items ordered (loop until user is done ordering) when they are done ordering items, output the total salessales.arrow_forwardpython code easy way pleasethe one that i provide the image with code is just starting codearrow_forward
- Python write a program in python that plays the game of Hangman. When the user plays Hangman, the computer first selects a secret word at random from a list built into the program. The program then prints out a row of dashes asks the user to guess a letter. If the user guesses a letter that is in the word, the word is redisplayed with all instances of that letter shown in the correct positions, along with any letters correctly guessed on previous turns. If the letter does not appear in the word, the user is charged with an incorrect guess. The user keeps guessing letters until either: * the user has correctly guessed all the letters in the word or * the user has made eight incorrect guesses. one for each letter in the secret word and Hangman comes from the fact that incorrect guesses are recorded by drawing an evolving picture of the user being hanged at a scaffold. For each incorrect guess, a new part of a stick-figure body the head, then the body, then each arm, each leg, and finally…arrow_forwardLists and strings: Perform the following using the list and string defined below: Mylist = [“orange”,”banana”,”apple”,”grape”] Mystring = “314-800-2346” What does Mylist[2] equal? What does Mystring[2] equal? What does the len(Mylist) equal? What does len(Mystring) equal? Write a for loop that will display the strings in Mylist from last to first. Write a line of code that appends “pear” to Mylistarrow_forwarddef delete_item(...): """ param: info_list - a list from which to remove an item param: idx (str) - a string that is expected to contain an integer index of an item in the in_list param: start_idx (int) - an expected starting value for idx (default is 0); gets subtracted from idx for 0-based indexing The function first checks if info_list is empty. The function then calls is_valid_index() to verify that the provided index idx is a valid positive index that can access an element from info_list. On success, the function saves the item from info_list and returns it after it is deleted from info_list. returns: If info_list is empty, return 0. If is_valid_index() returns False, return -1. Otherwise, on success, the function returns the element that was just removed from info_list. Helper functions: - is_valid_index() """arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
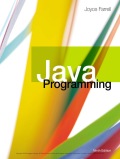