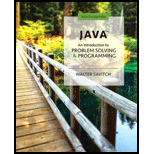
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 17E
Program Plan Intro
Revenue of Game Stadium
Program Plan:
- Import required package.
- Define “Main” class.
- Define main function.
- Create object “stadiumName” from “LinkedList” class.
- Create object “gameRevenue” from “LinkedList” class.
- Create object for scanner class.
- Display prompt statement.
- Assign “more_values” to “true”.
- Performs “while” loop.
- Read stadium name from user.
- If stadium name is not equal to “done”, then set “more_values” to “false”.
- Otherwise,
- Read game revenue from user.
- Add “st_name” to “stadiumName” by calling the method “addANodeToStart”.
- Add “game_revenue” to “gameRevenue” by calling the method “addANodeToStart”.
- Create array list for stadium names and game revenue.
- Display prompt statement.
- Read stadium name from user.
- Set “total” to “0.0”.
- Compute total amount of money for given stadium name.
- If “name” is equal to “stadium_name”, then compute total revenue for given name.
- Display total amount of money for given stadium name.
- Define main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A Maze Room :
In this lab, we will make a maze game. The maze is based on Linked Lists. Instead of having one possible direction (next), we will have 4 possible directions.
Rooms:
Use the below code as a basis to build your own room class. Implement this class in the file room.py
A room will be the basic object for our maze game. A room can have 4 doors (pertaining to north, south, east, and west). Attached to each these directions we have either another room or None (we could also imagine that the None doors are just walls).
We want the player to be able to tell what room they are in. Each room will have a unique description. When the player enters a room, the program will describe the room. This way the player will know if they went back to a room that have already been to.
You MAY NOT change the method's arguments/names in ANY way.
class Room():def __init__(self, descr):#Description of the room to print out#These should be unique so the player knows where they areself.descr =…
This is for python
For this assignment, you will write a program to simulate a payroll application. To that effect, you will also create an Employee class, according to the specifications below. Since an Employee list might be large, and individual Employee objects may contain significant information themselves, we store the Employee list as a linked list.
Employee class:
Attributes
• Employee ID: you can use a string – the ID will contain digits and/or hyphens. The ID must be provided during object construction and there should be no mechanism to change it later.
• Number of hours worked in a week: a floating-point number.
• Hourly pay rate: a floating-point number that represents how much the employee is paid for one hour of work.
• Gross wages: a floating-point number that stores the number of hours times the hourly rate.
Methods
• A constructor (__init__)
• Setter methods as needed.
• Getter methods as needed.
• This class should overload the __str__ or __repr__ methods so that…
Write a Java program that simulates a basic library delivery system. The user of the program will beable to add different types of items to library collection. In your program, as the main data structure,use the generic collection class ArrayList to store String references.Create three instances from ArrayList of String references. The first one will store the types, thesecond one will store the items, and the third one will store the owners of the items. The types areexactly the following: "Book", "DVD", "Magazine". The items and their owners will be read fromthe keyboard.Regarding the matching between an item and its owner, the location (index value) of an item in thelist of items will be the same with the location of its corresponding owner in the list of owners.As the next step, create the fourth instance from ArrayList of String references and call this list asitemsDeliveredByOwners. This last list will store String references to indicate the owners ofthe items (such as the…
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12.5 - What is the purpose of the FXML file?Ch. 12.5 - Prob. 32STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- Python For this assignment, you will write a program to allow the user to store the names and phone numbers of their contacts. Create the following classes: Contact: two attributes. One for the contact's name and the contact's phone number. Node: two attributes: _data and _next. For use in a linked list. ContactList: One attribute: _head, a reference to the head of an internal linked list of Node objects. Note: self._head is an attribute of the class, so any method which updates the internal linked list can simply update the list self._head references. There is no need to return anything like we did in methods such as add_to_end in the lecture. Note also that for the same reason, we do not have to pass a reference to the head of the list to any method, because each method should already have access to the self._head attribute. ContactList should also have the following methods: add(name, new_number). Creates a new Node with a Contact object as its _data. The new Node is then added…arrow_forwardImplement PokerValue as specified in textbook page 417, problem 33(a). Your implementation must include following: 1. At least one ADT type selected from textbook files such as Stack, Queue, Collection and List for storing the poker hand. 2. Use only CardDeck.java to hold deck of cards, and Card.java to represent each card. GUI is not required. 3. Default constructor to create a five-card poker hand from CardDeck.java. Make sure the poker hand is implemented with one of the textbook ADT as set forth above. 4. Overloaded constructor accepts an array of five cards to initialize one poker hand. 5. toString() method outputs in the same format as shown in SampleActualOutput.txt 6. You may add other methods as necessary. 7. Test your PokerValue.java with supplied PokerGame.java. Submit PokerValue.javaarrow_forwardonly tell given statement is true or falsearrow_forward
- Java with screen shotarrow_forwardWrite a generic class that stores two elements of the same type and can tell the user which one is the larger between the two. The type of the two elements should be parameterized and the class should have a function called “maxElement” that returns the larger element. write this java codearrow_forwardWe have a parking office class for an object-oriented parking management system using java Implement the following methods for our class Add equals and hashCode methods to any class used in a List. Add a method to the Parking Office to return a collection of customer ids (getCustomerIds) Add a method to the Parking Office to return a collection of permit ids (getPermitIds) Add a method to the Parking Office to return the collection of permit ids for a specific customer (getPermitIds(Customer)) The above methods are not included in the parking office class of our class diagram. I have attached class diagrams with definitions of related classes in our system (i.e car, customer, .....)arrow_forward
- For this lab, you will need to use the files BagInterface.java, Item.java, and LinkedBag.java.arrow_forwardA business that sells dog food keeps information about its dog food products in a linked list. The list is named dogFoodList. (This means dogFoodList points to the first node in the list.) A node in the list contains the name of the dog food (a String), a dog food ID (also a String) and the price (a double.) a.) Create a class for a node in the list. b.) Use this class to write pseudocode or Java for a public method that prints the name of all dog foods in the list where the price is more than $20.00.arrow_forwardJava programarrow_forward
- Using javaarrow_forwardWriting a program that helps to enter patients information:● Write a class patientInfo which contains the following data and methods:• Data:PatientName (String).PatientID (int).• Methods:Getter and Setter for each data item.toString method.● Display this menu:1:Merging two Single Linked List structure type2:Merging two Stacks data structure type3:Merging two Queses data structure type4:Merging Single Linked List with Stack to Linked List5:Merging Single Linked List with Queue to Linked List6:Merging Singly Linked List with Stack only Patient’s name that start with S to Queue● Ask the user to choose the data structure type from a given list to be merged.If you choose any of the above options:1.You must request [the size of data structures and patient information] from the user.2. Print the data structure before the merge.3. Print data structure after the merge.arrow_forwardIn this assignment, you will write a program that readsa list of people from a file, create a HashSet of the people, and print the people in the HashSet.The input filename ispassed in as a command line argument.•The program must implement a main class, and a Person class.•A template for the Person class is provided.A Person has a name and an address, both Strings.•If the input file name is not provided, your program should an error message and exit, e.g., Usage: java FuAssignment10/FuMain inputfile•If the specified input file does not exist or cannot be opened, your program should an error message and exit, e.g.,File abc.txt does not existor cannot be opened!•The input file lists one person per line. Each line contains aname and an address, separated by a comma, “,”.For example, the following are in a sample file students.txt:James Bond, 111 Main StTayer Smoke, 2334 Murry LnDavid Jones, 302 Toast AveAbby Wasch, 84 Sunny PlJames Bond, 111 Main St•Yourprogram should read each line,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
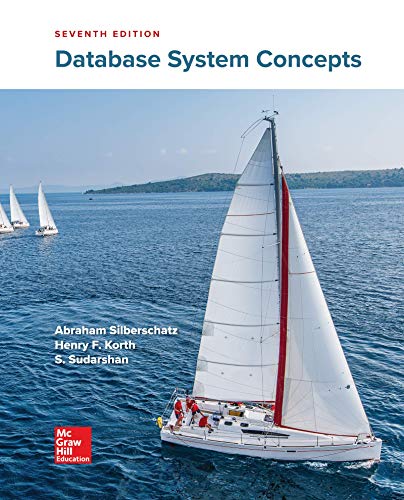
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
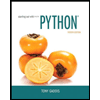
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
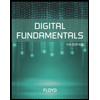
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
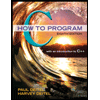
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
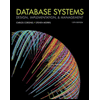
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
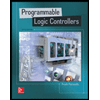
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education