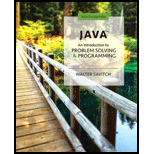
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 13PP
Program Plan Intro
Birds Survey
Program Plan:
- Import required package.
- Define “BirdSurvey” class.
- Create object “headNode” from “ListNode”.
- Create constructor for “BirdSurvey” class.
- Define the method “getReport()”.
- Compute position of node.
- Check condition using “while” loop.
- Display bird name and count.
- Define the method “computeLength()”.
- Set count to “0”.
- Set “position” to “headNode”.
- Check condition using “while” loop.
- Increment count
- Finally returns count value.
- Define the method “addANodeToStart” which is used to add bird name at start of list by using “ListNode” class.
- Define the method “add” with the argument of “bird”.
- If head node is null, then add bird to the front of list.
- Otherwise
- Compute given bird name by calling the method “find” and then store result to “n”.
- If “n” is null, then increment count value.
- Otherwise, find the last node in the list.
- Add in the node by using “ListNode” class.
- Define the method “getCount” with the argument of “bird”.
- Declare required variable.
- Create list for nodelist.
- If the bird is not on the list, then assign result of count to “0”.
- Otherwise, compute the result associated with bird species.
- Finally returns the value of result.
- Define the method “onList” with the argument of “t”.
- This method is used to check whether target “t” is on the list or not
- Define the method “find” with the argument of “t”.
- Assign required variables.
- Check condition using “while” loop.
- Define inner node class “ListNode”.
- Declare required variables.
- Create parameterized constructor for “ListNode” class.
- Assign values to required variables.
- Define main function.
- Create object “bs” from “BirdSurvey” class.
- Create object for scanner class.
- Display statement.
- Assign “moreValue” to “true”.
- Check condition using “while” loop.
- Read bird name from user.
- If the bird name is “done”, then set “moreValue” to “false”.
- Otherwise, add the bird name to “bs”.
- Display the birds report by calling the method “getReport”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
not sure how to do this problem output doesnt matter
You may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next.
We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as:
1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index()
Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null.
4. delete_at_start()
5. delete_at_end() .
6. display()
the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display().
hint on how to start thee code
# Initialize the Node
class Node:
def __init__(self, data):
self.item = data…
Implement class “LinkedList” which has two private data members head: A pointer to the Node class length: length of the linked listImplement the following private method:1. Node* GetNode(int index) const;A private function which is only accessible to the class methods. . For example, index 0 corresponds to the head and index length-1 corresponds to end node of the linked list. The function returns NULL if the index is out of bound.Implement the following public methods:2. LinkedList();Constructor that sets head to NULL and length equal to zero.3. bool InsertAt(int data, int index);Insert a new node at the index. Return true if successful, otherwise,return false. The new node should be at the position “index” in the linked list after inserting it. You might have to use GetNode private function.
these 3 parts
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12.5 - What is the purpose of the FXML file?Ch. 12.5 - Prob. 32STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- The implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forwardSend me your own work not AI generated response or plagiarised response. If I see these things, I'll give multiple downvotes and will definitely report.arrow_forwardImplement Two Way Linked List The MyLinkedList class is a one-way directional linked list that enables one-way traversal of the list. MyLinkedList.java is attached below. Modify the Node class to add the new field named previous to refer to the previous node in the list, as follows: public class Node <E> { E element; Node<E> next; Node<E> previous; public Node(E e) { element = e; } } Implement a new class named MyTwoWayLinkedList that uses a double linked list to store elements. The MyLinkedList class in the text extends MyList. Define MyTwoWayLinkedList to extend the java.util.AbstractSequentialList class. You can find methods of AbstractSequentialList from this article: https://www.geeksforgeeks.org/abstractsequentiallist-in-java-with-examples/. Study MyLinkedList.java carefully to understand how it is structured, and where and what you need to modify/implement for MyTwoWayLinkedList. Since MyTwoWayLinkedList extends java.util.AbstractSequentialList which implements…arrow_forward
- Implement a LinkedList class that stores integers using dynamic memory and a proper main program to test it. The following member functions need to be properly implemented and tested: 1. Default constructor. 2. Parmetrized Constructor. 3. Sum. 4. Average. 5. InsertAtHead. 6. InsertAtTail. 7. Delete. 8. Pop. 9. Circular. 10. Display. Sample answer is also provided.arrow_forwardA business that sells dog food keeps information about its dog food products in a linked list. The list is named dogFoodList. (This means dogFoodList points to the first node in the list.) A node in the list contains the name of the dog food (a String), a dog food ID (also a String) and the price (a double.) a.) Create a class for a node in the list. b.) Use this class to write pseudocode or Java for a public method that prints the name of all dog foods in the list where the price is more than $20.00.arrow_forwardIN JAVA: Use a singly-linked circular node class to implement the list. Refer to the illustration below:arrow_forward
- Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts public class ItemNode { private String item; private ItemNode nextNodeRef; // Reference to the next node public ItemNode() { item = ""; nextNodeRef = null; } // Constructor public ItemNode(String itemInit) { this.item = itemInit; this.nextNodeRef = null; } // Constructor public ItemNode(String itemInit, ItemNode nextLoc) {…arrow_forwardIn this task, a skip list data structure should be implemented. You can follow the followinginstructions:- Implement the class NodeSkipList with two components, namely key node and arrayof successors. You can also add a constructor, but you do not need to add anymethod.- In the class SkipList implement a constructor SkipList(long maxNodes). The parameter maxNodes determines the maximal number of nodes that can be added to askip list. Using this parameter we can determine the maximal height of a node, thatis, the maximal length of the array of successors. As the maximal height of a nodeit is usually taken the logarithm of the parameter. Further, it is useful to constructboth sentinels of maximal height.- In the class SkipList it is useful to write a method which simulates coin flip andreturns the number of all tosses until the first head comes up. This number representsthe height of a node to be inserted.- In the class SkipList implement the following methods:(i) insert, which accepts…arrow_forwardIN JAVA: Use a doubly-linked circular node class to implement the list. Refer to the illustration below.arrow_forward
- Make a doubly linked list and apply all the insertion, deletion and search cases. The node willhave an int variable in the data part. Your LinkedList will have a head and a tail pointer.Your LinkedList class must have the following functions: 1) insert a node1. void insertNodeAtBeginning(int data);2. void insertNodeInMiddle(int key, int data); //will search for keyand insert node after the node where a node’s data==key3. void insertNodeAtEnd(int data);2) delete a node1. bool deleteFirstNode(); //will delete the first node of the LL2. bool deleteNode(int key); //search for the node where its data==keyand delete that particular node3. bool deleteLastNode(); //will delete the last node of the LL3) Search a node1. Node* searchNodeRef(int key); //will search for the key in the datapart of the node2. bool searchNode(int key); The program must be completely generic, especially for deleting/inserting the middle nodes. Implement all the functions from the ABOVE STATEMENTS and make a login and…arrow_forwardJava Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Second image is ItemNodearrow_forwardComplete the implementation of the link-based set defined in the new class named LinkedSet that implements a set type using linked nodes. Hint: Much of the code in the LinkedBag class from your solution of the linkedbag.py file in Programming Exercise 5.5 can be reused. In the linkedset.py file, complete the following: Complete the accessor methods: isEmpty(), returns true if len(self) == 0, or false otherwise. __len__, returns the number of items in self. __str__, returns the string representation of self. __iter__, supports iteration over a view of self. __add__, returns anew set containing the contents of self and other clone, return a copy of self. __eq__, returns true if self equals other, or false otherwise count, return the numer of instance of item in self. Complete the mutator methods: clear, makes self become empty. add, adds item to self and ignores the item if it’s already in the set. Check array memory and increase it if necessary remove, removes item from self if…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
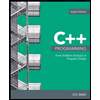
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning