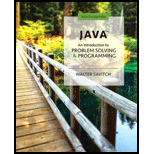
Explanation of Solution
Modified definition of “ListNode” class in Listing 12.4:
The modified definition of “ListNode” class in Listing 12.4 is given below:
//Define class "ListNode2"
public class ListNode2<E>
{
//Declare variable "data" and "link" in type parameter
private E data;
private ListNode2<E> link;
//Create default constructor for "ListNode2"
public ListNode2()
{
link = null;
data = null;
}
/* Create parameterized constructor for "ListNode2" */
public ListNode2(E newData, ListNode2<E> linkValue)
{
/* Assign value to "data" and "link" */
data = newData;
link = linkValue;
}
//Accessor method for assign value to data
public void setData(E newData)
{
data = newData;
}
//Mutator method for return data
public E getData( )
{
;&#x...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- 7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward
- 12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forwardYou are given a rope of length n meters and scissors that can cut the rope into any two pieces. For simplification, only consider cutting the rope at an integer position by the meter metric. Each cut has a cost associated with it, c(m), which is the cost of cutting the rope at position m. (You can call c(m) at any time to return the cost value.) The goal is to cut the rope into k smaller pieces, minimizing the total cost of cutting. B Provide the pseudo-code of your dynamic programming algorithm f(n,k) that will return the minimum cost of cutting the rope of length n into k pieces. Briefly explain your algorithm. What is the benefit of using dynamic programming for this problem? What are the key principles of dynamic programming used in your algorithm?arrow_forwardDetermine whether each of the problems below is NP-Complete or P A. 3-SAT B. Traveling Salesman Problem C. Minimum Spanning Tree D. Checking if a positive integer is prime or not. E. Given a set of linear inequalities with integer variables, finding a set of values for the variables that satisfies all inequalities and maximizes or minimizes a given linear objective function.arrow_forward
- 1. Based on our lecture on NP-Complete, can an NP-Complete problem not have a polynomial-time algorithm? Explain your answer. 2. Prove the conjecture that if any problem in NP is not polynomial-time solvable, then no NP-Complete problem is polynomial-time solvable. (You can't use Theorem 1 and 2 directly) 3. After you complete your proof in b), discuss how this conjecture can be used to solve the problem of whether P=NP.arrow_forwardBased on our lectures and the BELLMAN-FORD algorithm below, answer the following questions. BELLMAN-FORD (G, w, s) 1 INITIALIZE-SINGLE-SOURCE (G, s) 2 for i = 1 to |G. VI - 1 3 4 5 6 7 8 for each edge (u, v) = G.E RELAX(u, v, w) for each edge (u, v) = G.E if v.d> u.d+w(u, v) return FALSE return TRUE 1. What does the algorithm return? 2. Analyze the complexity of the algorithm.arrow_forward(Short-answer) b. Continue from the previous question. Suppose part of the data you extracted from the data warehouse is the following. Identify the missing values you think exist in the dataset. Use Column letter and Row number to refer to each missing value in the dataset. Please write down how you want to address each particular missing value (you can group them if they receive same treatment). For imputation, you do not need to calculate the exact imputed values but just describe what kind of value you want to use to impute.arrow_forward
- Please original work Locate data warehousing solutions offered by IBM, Oracle, Microsoft, and Amazon Compare and contrast the capabilities of each solution and provide several names of some organizations that utilize each of these solutions. Please cite in text references and add weblinksarrow_forwardNeed Help: Which of the following statements about confusion matrix is wrong A) Confusion matrix is a performance measure for probability prediction techniques B) Confusion matrix is derived based on classification rules with cut-off value 0.5 C) Confusion matrix is derived based on training partition to measure a model’s predictive performance D) None of the abovearrow_forwardI have a few questions I need help with Statement: When we build a nearest neighbor model, we shall not remove the redundant dummies when coding a categorical variable. True or False Statement: One reason why a neural network model often requires a significant number of data observations to train is that it often has a significant number of model parameters to estimate even if there are only a few predictors. True or False. Which of the following statements about confusion matrix is wrong A) Confusion matrix is a performance measure for probability prediction techniques B) Confusion matrix is derived based on classification rules with cut-off value 0.5 C) Confusion matrix is derived based on training partition to measure a model’s predictive performance D) None of the abovearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
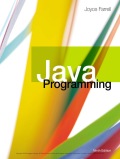