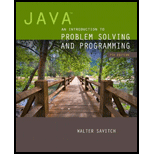
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 2E
Program Plan Intro
Count number of poor family using “ArrayList”
Program Plan:
“Family.java”:
- Declare required variables.
- Define constructor for “Family” class.
- Assign “income” to new variable “newIncome”.
- Assign “size” to new variable “nSize”.
- Define method “isPoor” with two arguments “housingCost” and “foodCost”.
- This method returns “true” if the value of “housingCost + foodCost * size” is greater than half of income. Otherwise returns “false”.
- Define method “toString” which is used to returns the given statement with “size” and “income”.
“CountPoor.java”:
- Import required package.
- Define “CountPoor” class.
- Define main function.
- Create object for scanner class.
- Create array for “incomeValues” using “ArrayList”.
- Display prompt statement for number of families.
- Assign “additionalData” to “true”.
- Set “index” to “0”.
- Read income and size for each family using “while” loop.
- Display prompt statement for each family.
- If user input has next double, then
- Read income and size of each family from user.
- Add income values and size to “Family” class using “add” method.
- Otherwise, assign “additionalData” to “false” and then finish reading data from user.
- Display prompt statement for average housing cost and food cost.
- Read the average house cost and food cost from user.
- Initializes the count value to “0”.
- Compute the number of poor family using “for” loop.
- Check if the given family is poor by using “isPoor” function.
- If it is, then increment the count value and display poor family details using “get” method.
- Finally display the count of poor family.
- Define main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
make corrections of this program based on the errors shown. this is CIS 227 .
Create 6 users: Don, Liz, Shamir, Jose, Kate, and Sal.
Create 2 groups: marketing and research.
Add Shamir, Jose, and Kate to the marketing group.
Add Don, Liz, and Sal to the research group.
Create a shared directory for each group.
Create two files to put into each directory:
spreadsheetJanuary.txt
meetingNotes.txt
Assign access permissions to the directories:
Groups should have Read+Write access
Leave owner permissions as they are
“Everyone else” should not have any access
Submit for grade:
Screenshot of /etc/passwd contents showing your new users
Screenshot of /etc/group contents showing new groups with their members
Screenshot of shared directories you created with files and permissions
⚫ your circuit diagrams for your basic bricks, such as AND, OR, XOR gates and 1 bit multiplexers,
⚫ your circuit diagrams for your extended full adder, designed in Section 1 and
⚫ your circuit diagrams for your 8-bit arithmetical-logical unit, designed in Section 2.
1 An Extended Full Adder
In this Section, we are going to design an extended full adder circuit (EFA). That EFA takes 6 one bit inputs: aj, bj,
Cin, Tin, t₁ and to. Depending on the four possible combinations of values on t₁ and to, the EFA produces 3 one bit
outputs: sj, Cout and rout.
The EFA can be specified in principle by a truth table with 26 = 64 entries and 3 outputs. However, as the EFA
ignores certain inputs in certain cases, it is easier to work with the following overview specification, depending only
on t₁ and to in the first place:
t₁ to Description
00
Output Relationship
Ignored
Inputs
Addition Mode
2 Coutsjaj + bj + Cin, Tout= 0
Tin
0 1
Shift Left Mode
Sj = Cin,
Cout=bj, rout = 0
rin, aj
10
1 1
Shift Right…
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- Show the correct stereochemistry when needed!! mechanism: mechanism: Show the correct stereochemistry when needed!! Br NaOPh diethyl ether substitutionarrow_forwardIn javaarrow_forwardKeanPerson #keanld:int #keanEmail:String #firstName:String #lastName: String KeanAlumni -yearOfGraduation: int - employmentStatus: String + KeanPerson() + KeanPerson(keanld: int, keanEmail: String, firstName: String, lastName: String) + getKeanld(): int + getKeanEmail(): String +getFirstName(): String + getLastName(): String + setFirstName(firstName: String): void + setLastName(lastName: String): void +toString(): String +getParkingRate(): double + KeanAlumni() + KeanAlumni(keanld: int, keanEmail: String, firstName: String, lastName: String, yearOfGraduation: int, employmentStatus: String) +getYearOfGraduation(): int + setYearOfGraduation(yearOfGraduation: int): void +toString(): String +getParkingRate(): double In this question, write Java code to Create and Test the superclass: Abstract KeanPerson and a subclass of the KeanPerson: KeanAlumni. Task 1: Implement Abstract Class KeanPerson using UML (10 points) • Four data fields • Two constructors (1 default and 1 constructor with all…arrow_forward
- Plz correct answer by best experts...??arrow_forwardQ3) using the following image matrix a- b- 12345 6 7 8 9 10 11 12 13 14 15 1617181920 21 22 23 24 25 Using direct chaotic one dimension method to convert the plain text to stego text (hello ahmed)? Using direct chaotic two-dimension method to convert the plain text to stego text?arrow_forward: The Multithreaded Cook In this lab, we'll practice multithreading. Using Semaphores for synchronization, implement a multithreaded cook that performs the following recipe, with each task being contained in a single Thread: 1. Task 1: Cut onions. a. Waits for none. b. Signals Task 4 2. Task 2: Mince meat. a. Waits for none b. Signals Task 4 3. Task 3: Slice aubergines. a. Waits for none b. Signals Task 6 4. Task 4: Make sauce. a. Waits for Task 1, and 2 b. Signals Task 6 5. Task 5: Finished Bechamel. a. Waits for none b. Signals Task 7 6. Task 6: Layout the layers. a. Waits for Task 3, and 4 b. Signals Task 7 7. Task 7: Put Bechamel and Cheese. a. Waits for Task 5, and 6 b. Signals Task 9 8. Task 8: Turn on oven. a. Waits for none b. Signals Task 9 9. Task 9: Cook. a. Waits for Task 7, and 8 b. Signals none At the start of each task (once all Semaphores have been acquired), print out a string of the task you are starting, sleep for 2-11 seconds, then print out a string saying that you…arrow_forward
- Programming Problems 9.28 Assume that a system has a 32-bit virtual address with a 4-KB page size. Write a C program that is passed a virtual address (in decimal) on the command line and have it output the page number and offset for the given address. As an example, your program would run as follows: ./addresses 19986 Your program would output: The address 19986 contains: page number = 4 offset = 3602 Writing this program will require using the appropriate data type to store 32 bits. We encourage you to use unsigned data types as well. Programming Projects Contiguous Memory Allocation In Section 9.2, we presented different algorithms for contiguous memory allo- cation. This project will involve managing a contiguous region of memory of size MAX where addresses may range from 0 ... MAX - 1. Your program must respond to four different requests: 1. Request for a contiguous block of memory 2. Release of a contiguous block of memory 3. Compact unused holes of memory into one single block 4.…arrow_forwardusing r languagearrow_forwardProgramming Problems 9.28 Assume that a system has a 32-bit virtual address with a 4-KB page size. Write a C program that is passed a virtual address (in decimal) on the command line and have it output the page number and offset for the given address. As an example, your program would run as follows: ./addresses 19986 Your program would output: The address 19986 contains: page number = 4 offset = 3602 Writing this program will require using the appropriate data type to store 32 bits. We encourage you to use unsigned data types as well. Programming Projects Contiguous Memory Allocation In Section 9.2, we presented different algorithms for contiguous memory allo- cation. This project will involve managing a contiguous region of memory of size MAX where addresses may range from 0 ... MAX - 1. Your program must respond to four different requests: 1. Request for a contiguous block of memory 2. Release of a contiguous block of memory 3. Compact unused holes of memory into one single block 4.…arrow_forward
- using r languagearrow_forwardWrite a function to compute a Monte Carlo estimate of the Beta(3, 3) cdf, and use the function to estimate F(x) for x = 0.1,0.2,...,0.9. Compare the estimates with the values returned by the pbeta function in R.arrow_forwardWrite a function to compute a Monte Carlo estimate of the Gamma(r = 3, λ = 2) cdf, and use the function to estimate F(x) for x = 0.2, 0.4, . . . , 2.0. Compare the estimates with the values returned by the pgamma function in R.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
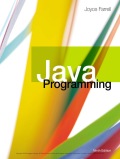
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
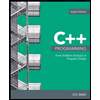
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
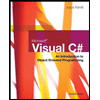
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
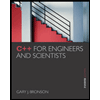
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr