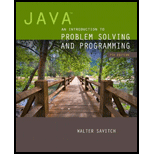
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12.1, Problem 7STQ
Program Plan Intro
“add” method in “ArrayList” class:
- • “add” method is used to add the particular element at the end of the list and increases the size of lists by one.
- • Users use the “add” method to set an element for the first time.
Example:
The example for adding string is given below:
Consider, “nameList” is an object of class “ArrayList<String>”. User can add the string “Rose” to the list “nameList” which is given below:
nameList.add("Rose");
User can also add the string for particular index on the given list. Suppose if user want to place a string at index “6” in “nameList”. The index size starts from “0” to “7”.
nameList.add(6, "Merry");
The above code snippet is used to insert the name “Merry” at index “6”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q8. Write a declaration of a private field named department that can hold an ArrayList. The elements
of the ArrayList are of type Student. Write an assignment to the department variable to create an
appropriate ArrayList object.
Q9. (A) If a collection stores 20 objects, what value would be returned from a call to its size method?
(B) Write a method call using get to return the fifth object stored in a collection called department.
Q10. Write a method call to add the object held in the variable student1 to a collection department
files.
2. The class PetShop has a single field as defined below: private ArrayList petList;
Define a method to print out the details of all cats in petList in PetShop.
Exercise 2.
Create a Book class where:
Each book contains the following information: book title book Author name, barcode (as
fong integers), and book topic.
o Implement an appropriate constructor(s) and all necessary get/set methods.
Test Book class:
o Create diffcrent book objects (at least 5 hooks) and store them in a LinkedList sorted by
book barcode value Generate a unique random integer value for the barcode.
Iterate through the LinkedList and print out the books' details
O Create a second LinkedList object containing a copy of the above LinkedList, but in
reverse order.
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- 2. Revise the genericStack class we did in the class to implement it using an array instead of ArrayList. You need to check the array size before adding a new element. If it is full, create a new array that double the current size and copy the elements from the current to the new array and then add the new array. (2 points) 3. Use the genericStack class we did the class (not the one in question 2), implement the binary search public static <E extends Comparable<E>) int binarySearch (E[] list, E key) and a method that returns the maximum value element public static <E extends Comparable<E>) E max(E[] list (2 points)arrow_forwardIf a method given an ArrayList as a parameter calls a second method giving it the same ArrayList, and that second method deletes one of the elements, this will: (produce a non-deterministic result? trigger an error? have an effect? have no effect?) on the first ArrayList. Java uses: (pass by reference? pass by value?) So when an object is passed to a method, what is actually passed is just : (the name of the object? a pointer to the object? the index of the object?) . Select the correct answerarrow_forwardA pet shop wants to give a discount to its clients if they buy one or more pets and at least five other items. The discount is equal to 20 percent of the cost of the other items, but not the pets. Use a class Item to describe an item, with any needed methods and a constructor public Item(double price, boolean isPet, int quantity) An invoice holds a collection of Item objects; use an arraylist to store them. In the Invoice class, implement methods public void add(Item anItem) public double getDiscount() Write a program that prompts a cashier to enter each price and quantity, and then a Y for a pet or N for another item. Use a price of -1 as a sentinel. In the loop, call the add method; after the loop, call the getDiscount method and display the returned value.arrow_forward
- A programmer is designing a program to catalog all books in a library. He plans to have a Book class that stores features of each book: author, title, isOnShelf, and so on, with operations like getAuthor, getTitle, getShelf Info, and set Shelf Info. Another class, LibraryList, will store an array of Book objects. The LibraryList class will include operations such as listAll Books, addBook, removeBook, and searchForBook. The programmer plans to implement and test the Book class first, before implementing the LibraryList class. The program- mer's plan to write the Book class first is an example of (A) top-down development. (B) bottom-up development. (C) procedural abstraction. (D) information hiding. (E) a driver program.arrow_forwardYou will be given an ArrayList of Integers. This ArrayList will have at least 2 items. Starting with the second string in the list, remove it if the string before it comes first alphabetically. Then print the ArrayList. You cannot change the method header! public ArrayList<String> removeStrings(ArrayList<String> arrList) { return null; // Return the ArrayList as well as printing it. }arrow_forwardjava Write a program to do the following: Create an ArrayList of Student. Add 7 elements to the ArrayList. Use for each loop to print all the elements of an ArrayList. Remove an element from an ArrayList using the index number. Remove the element from an ArrayList using the content (element name).arrow_forward
- The play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…arrow_forwardWrite Java code for the following: Create an Arraylist that can hold Integer objects. Check and display whether the Arraylist is empty or not. Add an element 50 into the Arraylist. Add another element 60 in the position of 0 in the Arraylist. Replace the element to 70 in index 1.arrow_forwardThis is needed in Java Given an existing ArrayList named friendList, find the first index of a friend named Sasha and store it in a new variable named index.arrow_forward
- Exercise 2: Create a Book class; where: lo Each book contains the following information: book title, book Author name, barcode (as long integers) and book topic. 6 Implement an appropriate constructor(s) and all necessary get/set methods. Test Book class: lo Create different book objects (at least 5 books) and store them in a LinkedList sorted by book barcode value. Generate a unique random integer value for the barcode. O Iterate through the LinkedList and print out the books' details o Create a second LinkedList object containing a copy of the above LinkedList, but in reverse orderarrow_forward1. Write a method called doubleListthat takes an ArrayList of strings as a parameter and replaces every string with two of that same string. For example, if the list stores the values ["how", "are", "you?"] before the method is called, it should store the values ["how", "how", "are", "are", "you?", "you?"] after the method finishes executing.In your program1, you also need to create a class namedProgram1.java, where you are going to construct some arralylists to test the doubleList within the static void main method. In the output, it will print out the original list and the new list after calling the doubleList method. 2.Write a method called filterRange that accepts an ArrayList of integers and two integer values min and max as parameters and removes all elements whose values are in the range min through max (inclusive). For example, if a variable calledlist stores the values [4, 7, 9, 2, 7, 7, 5, 3, 5, 1, 7, 8, 6, 7], the call of filterRange(list, 5, 7);should remove all values…arrow_forwardJava In this assignment, you will practice using an ArrayList. Your class will support a sample program that demonstrates some of the kinds of operations a windowing system must perform. Instead of windows, you will be manipulating “tiles.” A tile consists of a position (specified by the upper-left corner x and y), a width, a height, and color. Positions and distances are specified in terms of pixels, with the upper-left corner being (0, 0) and the x and y coordinate increasing as you move left and down, respectively. You won’t have to understand a lot about tiles and coordinates. That code has been written for you. You are writing a small part of the overall system that keeps track of the list of tiles. You are required to implement this using an ArrayList. The only method you need to use from the Tile class is the following: // returns true if the given (x, y) point falls inside this tile public boolean inside(int x, int y) Your class is to be called TileList and must…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
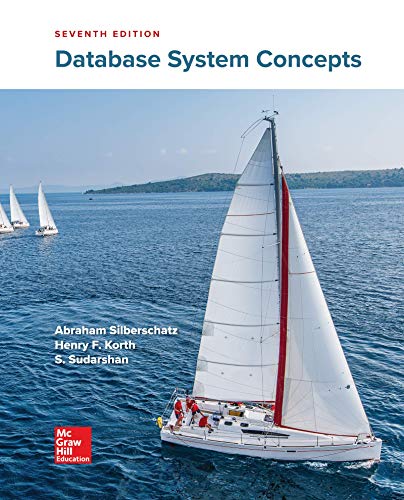
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
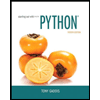
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
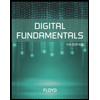
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
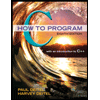
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
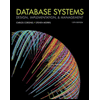
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
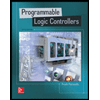
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education