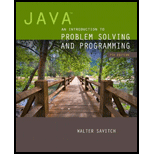
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 12, Problem 14E
Explanation of Solution
Creating the class “ListDemoTestOne.java”:
- Define “ListDemoTestOne” class.
- Define main function.
- Create object “list1” from “StringLinkedListWithIterator” class.
- Add node a, b, c and d to “list1” by calling the method “addANodeToStart”.
- Display the list by calling the method “showList”.
- Sets the iterator is at the front of the list by calling the method “resetIteration”.
- Finds the data at the current node by calling the method “getDataAtCurrent”.
- Deletes current node by calling the method “deleteCurrentNode”.
- Performs “for” loop. It is for iterator to next node by calling the method “goToNext”.
- Insert “nodeData” after the current node by calling the method “insertNodeAfterCurrent”.
- Display the list by calling the method “showList”.
- Define main function.
Program:
Filename: “StringLinkedListWithIterator.java”
//Define "StringLinkedListWithIterator" class
public class StringLinkedListWithIterator
{
/* It is same as "Listing 12.9" */
}
Filename: “ListDemoTestOne.java”
//Import required package
import java.io.*;
import java.util.*;
//Define "ListDemoTestOne" class
public class ListDemoTestOne
{
//Define main function
public static void main(String[] args)
{
//Create object "list1" from "StringLinkedListWithIterator" class
StringLinkedListWithIterator list1 = new StringLinkedListWithIterator();
//Display statement
System...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In this assignment, you will compare the performance of ArrayList and LinkedList. More specifically, your program should measure the time to “get” and “insert” an element in an ArrayList and a LinkedList.You program should 1. Initializei. create an ArrayList of Integers and populate it with 100,000 random numbersii. create a LinkedList of Integers and populate it with 100,000 random numbers2. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the ArrayList 3. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the LinkedList 4. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the ArrayList 5. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the LinkedList 6. You must print the time in milliseconds (1 millisecond is 1/1000000 second).A sample run will be like this:Time for get in ArrayList(ms): 1Time for get in…
Write a line (or lines) of code that uses a list that has been previously defined, named word_list, along with a string value entered by the user beforehand, named find, to print out the percent of the occurrence of that word in the list.
As an example, if your word_list looked like this: ['the', 'word', 'I', 'am', 'looking', 'for', 'is', 'called', 'my', 'word']
And find was the string 'word'
The Example Output would look like this: 20.00% of the list is word
Otherwise, if your word_list looked like this: ['another', 'word', 'that', 'is', 'being', 'found', 'is', 'terracotta']
And find was the string 'looking'
That Example Output would look like this: 0.00% of the list is looking
you are given a variable zipcode_list that contains a list.
Write some code that assigns True to the variable duplicates if the there are two adjacent elements in the list that have the same value, but that otherwise assigns False to duplicates otherwise.
use only variables k, zipcode_list, and duplicates
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- 1. Write a method called doubleListthat takes an ArrayList of strings as a parameter and replaces every string with two of that same string. For example, if the list stores the values ["how", "are", "you?"] before the method is called, it should store the values ["how", "how", "are", "are", "you?", "you?"] after the method finishes executing.In your program1, you also need to create a class namedProgram1.java, where you are going to construct some arralylists to test the doubleList within the static void main method. In the output, it will print out the original list and the new list after calling the doubleList method. 2.Write a method called filterRange that accepts an ArrayList of integers and two integer values min and max as parameters and removes all elements whose values are in the range min through max (inclusive). For example, if a variable calledlist stores the values [4, 7, 9, 2, 7, 7, 5, 3, 5, 1, 7, 8, 6, 7], the call of filterRange(list, 5, 7);should remove all values…arrow_forwardImport the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes. Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes. Create a List called phoneNumbers and use the add method to add several phone numbers to the list. List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234"); Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method. Finally, use the println method to print the prefixes set, which will contain all of…arrow_forwardThis problem has you write a nested loop to process a list of list of int, and accumulate a list of list of int. The starter code provides an accumulator for the result and a loop over the lists, and you need to write the code that checks whether sublist contains only even ints. You'll probably want a one-way flag: a Boolean variable that starts out as True and is set to False if you find an odd int in the sublist. You'll need to check the value of this variable to figure out whether to append sublist to the even_lists accumulator. 1 def only_evens (1st: list[list[int]]) -> list[list[int]]: """Return a list of the lists in 1st that contain only even integers. 4 >>> only_evens ([[1, 2, 4], [4, 0, 6], [22, 4, 3], [2]]) [[4, 0, 6], [2]] 6. 8. even_lists = [] 10 for sublist in 1st: 11 12 # write your code here (please read above for a suggested approach) 13 14 return even_listsarrow_forward
- This problem has you write a nested loop to process a list of list of int, and accumulate a list of list of int. The starter code provides an accumulator for the result and a loop over the lists, and you need to write the code that checks whether sublist contains only even ints. You'll probably want a one-way flag: a Boolean variable that starts out as True and is set to False if you find an odd int in the sublist. You'll need to check the value of this variable to figure out whether to append sublist to the even_lists accumulator. 1 def only evens (1st: list[list[int]]) -> list[list[int]]: **"Return a list of the lists in 1st that contain only even integers. 2. 3. >>> only_evens ([[1, 2, 4], [4, e, 6], [22, 4, 3], [2]]) 4. [[4, e, 6], [2]] 8. even_lists = [] 10 for sublist in 1st: 11 12 13 14 return even_lists History Submit P Type here to search 100% 1:1 8°C Cloudy A 4) ENG 10/2arrow_forward3. Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to avoid method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time. See Sample Output. SAMPLE OUTPUT Here is the list Lionel Messi Drake Adele Dwayne Johnson Beyonce Here is the new list * Lionel Messi * Drake * Taylor Swift * Adele * Beyonce Using an iterator, here is the list Lionel Messi Drake Taylor Swift Adele Beyoncearrow_forward7. You could create a circular list from a single- linked list by executing the statement. а. tail.next = head b. head. prev = next c. tail. head next d. head.tail = prev 8. How much time does an ArrayList add a new element to the beginning of the list if n is the size of the ArrayList? а. О(1) b. 0(n) c. O(n²) d. 0(log n) 9. How much time does an ArrayList add a new element to the end of the list if n is the size of the ArrayList? a. 0(1) b. 0(n) c. O(n2) d. 0(log n)arrow_forward
- In kotlin, write code of that creates a list of strings that can be null.arrow_forwardWhat happens when you remove the entry from position 4 from a List? Select one: a. All of these b. The entry in the 4th position is returned (if there was a 4th entry) c. If the List has 3 or fewer entries, an IndexOutOfBoundsException is thrown d. If the List has 5 or more entries, the entries in positions 5 and up will move up 1 spot earlier to close up the gap vacated by removing the 4th entryarrow_forwardWrite a Java application CountryList. In the main method, do the following:1. Create an array list of Strings called countries.2. Add "Canada", "India", "Mexico", "Peru" in that order.3. Use the enhanced for loop to print all countries in the array list, one per line.4. Add "Spain" at index 15. Replace the element at index 2 with "Vietnam". You must use the set method.6. Replace the next to the last element with "Brazil". You must use the set method. You willlose one point if you use 3 in the set method. Do this in a manner that would replace thenext to the last element, no matter the size of the array list.7. Remove the object "Canada" Do not remove at an index. Your code should work if"Canada" was at a different location. There is a version of remove method that willremove a specific object.8. Get and print the first element followed by "***"9. Call method toString() on countries to print all elements on one line.10. Use the enhanced for loop to print all countries in the array list,…arrow_forward
- Create a program that removes duplicate integer values from a list. For example if a list has these valuesList = [5, 7, 8, 10, 8, 5, 12, 15, 12]. After applying the removal function, you should have List = [5, 7, 8,10, 12, 15]. In your case you will get the numbers of list from the user.arrow_forwardA business that sells dog food keeps information about its dog food products in a linked list. The list is named dogFoodList. (This means dogFoodList points to the first node in the list.) A node in the list contains the name of the dog food (a String), a dog food ID (also a String) and the price (a double.) a.) Create a class for a node in the list. b.) Use this class to write pseudocode or Java for a public method that prints the name of all dog foods in the list where the price is more than $20.00.arrow_forwardCreate an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
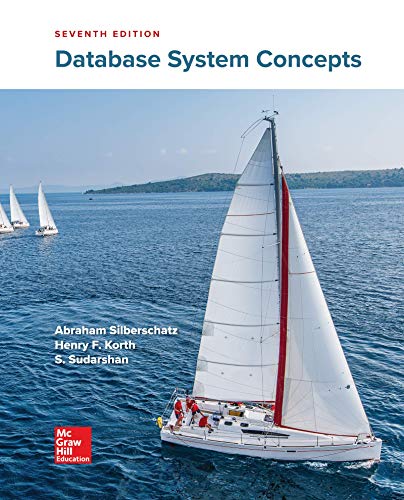
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
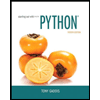
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
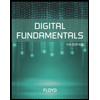
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
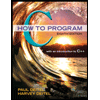
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
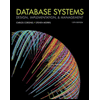
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
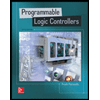
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education