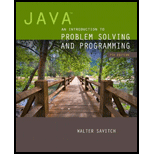
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12.2, Problem 13STQ
Program Plan Intro
“HashSet” class:
- This class is able to store a set of objects. It also implements the “Collection” interface.
- This class stores a set instead of list of items.
- It means that there can be no duplicate elements.
- The class is named “HashSet” since the
algorithm used to implement the set is called a hash table. - The package for “HashSet” class is “import java.util.HashSet;”
Syntax for defining “HashSet” class:
HashSet<Base_type> variable_name = new HashSet<Base_type>();
From the above syntax, “Base_type” represents “Integer”, “Double” or “String”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Java Code: Add a HashMap to your Lexer class and initialize all the keywords. Change your lexer so that it checks each string before making the WORD token and creates a token of the appropriate type if the work is a key word. When the exact type of a token is known, you should NOT fill in the value string, the type is enough. For tokens with no exact type we still need to fill in the token’s string. Rename “WORD” to “IDENTIFIER”.
Strings and characters will require some additions to your state machine. Create “STRINGLITERAL” and “CHARACTERLITERAL” token types. These cannot cross line boundaries.
Your lexer should throw an exception if it encounters a character that it doesn’t expect outside of a comment, string literal or character literal. Create a new exception type that includes a good error message and the token that failed. Ensure that the ToString method prints nicely.
Add “line number” to your Token class. Keep track of the current line number in your lexer and populate each…
In java please help with the following:
Create struct HashNode and class HashTable that will be used to store students' data. For each student,
it'll store the ID (string) as the key, and the first name (string), last name (string), and email (string) as
the value. You can imagine a single HashNode as follows:
"123456789"
"Jessica"
"Wolf"
"abcdef@bartleby.com"
HashTable should have the following attributes and methods:
Attributes
1. The dynamic array that stores the data.
2. The number of elements currently stored.
3. The maximum number of elements the container can store.
Methods
1. Constructor that takes from the user the maximum size of the container.
2. void insert(string ID, string firstName, string lastName, string email): uses the
following hash function h2(h1(ID)) to map the key to a specific index in the table:
• h1(ID):
ww
IDLength -1
Σ
ID
• h2(K) -> [0, N-1], where N is the table size
In case the index that the hash function generated was already full, the insert
function should add the student information in a…
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- Code for the demonstration application will generate a hash map of type hash map and read and write items to the data structure using overloaded array subscripts. The heavy work is done inside, and the data structure seems to be virtually like a typical array from the outside.arrow_forward1. The following interfaces are part of the Java Collections Framework except? a. SortedListb. SortedMapc. Setd. ArrayListe. HashSet 2. Which assertion about the HashMap class is not true? a. HashMap class is not synchronized.b. HashMap class extends AbstractMap and implements Map interface.c. HashMap maintain order of its element.d. HashMap uses a hashtable to store the map.arrow_forward. Write a code using the following hints and apply HashMap<K, V> and its methods get and put for public class CourseInformation (i) Create a HashMap object called Course to add a key and its value. //Key is your course name and value is your course code. (ii) Use put method to map a course name with the course code. // add any FOUR COURSE NAMES WITH ITS COURSE CODE which are represented as a String (iii) Use get method to find the course code for any one of the course.arrow_forward
- Is the following declaration of a HashMap valid: HashMap nun new HashMap(); True Falsearrow_forwardJava Objects and Linked Data: Select all of the following statements that are true. The Java Class Library's class ArrayList implements the LinkedList interface. In Java, when one reference variable is assigned to another reference variable, both references then refer to the same object. In Java, the "equals"-method that any object inherits can be overwritten to compare objects field by field.arrow_forwardDefine a class named Element with the following private data fields: String name; // The name of the chemical element int atomicNumber; // The atomic number of the element double atomicWeight; // The atomic weight of the element Override the toString() and equals() methods for the class with methods you design. Define a Java HashMap named elements that uses the String value of a chemical symbol as the key and an instance of the Element class as the value. For example, the chemical symbol for Carbon is the letter “C”. The atomic number of Carbon is 6, and the atomic weight of Carbon is 12.011. To add Carbon to the HashMap named elements, first create an instance of the Element class using “Carbon”, 6, and 12.011. Then add an entry to the HashMap collection using “C” as the key and the Element object as the value. Write a menu-driven program that prompts your user with the following six choices: Select an option number from the following menu: Option Action ------…arrow_forward
- Java Code: Look through the Language Description and build a list of keywords. Add a HashMap to your Lexer class and initialize all the keywords. Change your lexer so that it checks each string before making the WORD token and creates a token of the appropriate type if the work is a key word. When the exact type of a token is known (like “WHILE”), you should NOT fill in the value string, the type is enough. For tokens with no exact type (like “hello”), we still need to fill in the token’s string. Finally, rename “WORD” to “IDENTIFIER”. Similarly, look through the Language Description for the list of punctuation. A hash map is not necessary or helpful for these – they need to be added to your state machine. Be particularly careful about the multi-character operators like := or >=. These require a little more complexity in your state machine. Strings and characters will require some additions to your state machine. Create “STRINGLITERAL” and “CHARACTERLITERAL” token types. These…arrow_forwardIs the following declaration of a HashMap valid: HashMap nums = new HashMap(); True False An enumeration can contain public constant variables True False An interface can extend as many interfaces as the programmer wants O True O Falsearrow_forwardDesign a class named Date that meets the followingrequirements:■■ Three data fields year, month, and day for representing a date■■ A constructor that constructs a date with the specified year, month, and day■■ Override the equals method■■ Override the hashCode method. (For reference, see the implementation of theDate class in the Java API)arrow_forward
- Create the PolynomialDemoClass: instantiate and initialize PolynomialDataStrucClass objects p1, p2, p3, p4 Add terms to the polynomials (pass 2 arguments to the method: coefficient and exponent- for example: addPolyNodeLast(4, 3);) Print out p1, p2 and sum of the polynomials AND p3, p4, and sum of the polynomials Use: p1= 4x^3 + 3x^2 – 5 ; p2 = 3x^5 + 4x^4 + x^3 – 4x^2 + 4x^1 + 2 AND p3= -5x^0 + 3x^2 + 4x^3 ; p4 = -4x^0 + 4x^3 + 5x^4arrow_forwardJAVAarrow_forwardA map has the form Map <k,v> where: K: specifies the type of keys maintained in this map.V: defines the type of mapped values.Furthermore, the Map interface provides a set of methods that must be implemented. In this section, we will discuss about the most famous methods: clear: Removes all the elements from the map.containsKey: Returns true if the map contains the requested key.containsValue: Returns true if the map contains the requested value.equals: Compares an Object with the map for equality.get: Retrieve the value of the requested key.entrySet: Returns a Set view of the mappings contained in this map.keySet: Returns a Set that contains all keys of the map.put: Adds the requested key-value pair in the map.remove: Removes the requested key and its value from the map, if the key exists.size: Returns the number of key-value pairs currently in the map. Here is an example of TreeMap with a Map: import java.util.Map; import java.util.TreeMap; public class TreeMapExample {…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
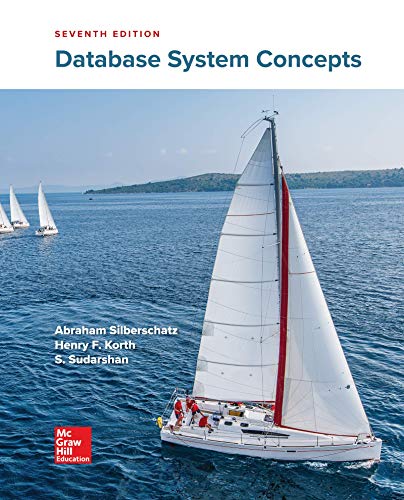
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
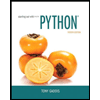
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
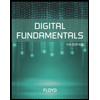
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
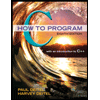
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
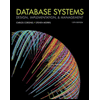
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
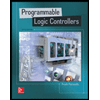
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education