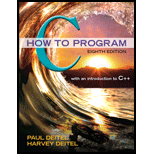
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 12.17E
Program Plan Intro
Program plan:
- line, token variables are used for input. There is structure Treenode having data, leftptr, rightptr member variables which represent the tree node.
- void insertnode(node **ptr, char *value) function inserts the node in the tree.
- void preorder(node *ptr) function displays the contents of the tree in a preorder fashion.
- void inorder(node *ptr) function displays the contents of the tree in an in-order fashion.
- void postorder(node *ptr) function display the contents of the tree in a post-order fashion.
Program description:
The main purpose of the program is to create a tree from the line entered by the user and split it into the word. Then these words are inserted into the tree. This created tree contents are displayed in preorder, inorder and postorder fashion.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The assignment here is to write an app using a database named CIT321 with a collection named
students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should
know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes
for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be
extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of
class.
The database contains 51 documents. The first rows of the CSV file look like this:
sid
last_name
1 Astaire
first_name
Humphrey CIT
major
hrs_attempted
gpa_points
10
34
2
Bacall
Katharine EET
40
128
3 Bergman
Bette
EET
42
97
4
Bogart
Cary
CIT
11
33
5 Brando
James
WEB
59
183
6 Cagney
Marlon
CIT
13
40
GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by
adding 4 points for each credit hour of A, 3 points for each credit hour of…
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Chapter 12 Solutions
C How to Program (8th Edition)
Ch. 12 - Prob. 12.6ECh. 12 - (Merging Ordered Lists) Write a program that...Ch. 12 - Prob. 12.8ECh. 12 - (Creating a Linked List, Then Reversing Its...Ch. 12 - Prob. 12.10ECh. 12 - Prob. 12.11ECh. 12 - Prob. 12.12ECh. 12 - Prob. 12.13ECh. 12 - Prob. 12.14ECh. 12 - (Supermarket Simulation) Write a program that...
Knowledge Booster
Similar questions
- Could you help me to know features of the following concepts: - defragmenting. - dynamic disk. - hardware RAIDarrow_forwardwhat is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forward
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
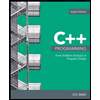
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
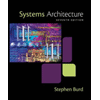
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
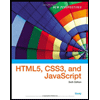
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
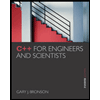
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
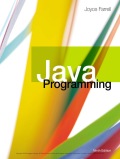
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT