(Algebra: perfect square ) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, 5 in an array list. 2 and 5 appear an odd number of times in the array list. So, n is 10.) Sample Run 1 Enter an integer m: 1500 The smallest number n for m x n to be a perfect square is 15 m x n is 22500 Sample Run 2 Enter an integer m: 63 The smallest number n for m x n to be a perfect square is 7 m x n is 441 Class Name: Exercise11_17 Answer is : import java.util.Scanner; public class Squares { public static void main(String[] args) { Scanner scan = new Scanner(System.in); //instantiation of Scanner that will take inputs from the keyboard //the try catch block below is for trapping error with the input try { System.out.print("Enter an integer m: "); //prompts the user to enter a number int m = scan.nextInt(); //stores the user input to m int n = 0; //initializing n for(int i=1; ; i++) { //this loop only ends with the 'break keyword' which is why the 2nd argument is blank int product = i * m; double squareRoot = Math.sqrt(product); if(squareRoot - Math.floor(squareRoot) == 0) { //when the square root is just a whole number(when decimal part is just zero), it is a perfect square n = i; break; //loop ends here } } System.out.println("The smallest number n for m * n to be a perfect square is " + n); //prints out n System.out.println("m * n is " + (m*n)); //prints out product of m and n }catch(IllegalArgumentException e) { System.out.println("Invalid input!"); //prints out error message } } } But after that its showing the follwoing : Exercise11_17.java:5: error: class Squares is public, should be declared in a file named Squares.java public class Squares { 1 error What Am i doing wrong? Can you correct me? Thank You
(Algebra: perfect square
)
Write a program that prompts the user to enter an integer
m
and find the smallest integer
n
such that
m * n
is a perfect square.
(Hint: Store all smallest factors of
m
into an array list.
n
is the product of the factors that appear an odd number of times in the array list.
For example, consider
m
= 90, store the factors 2, 3, 3, 5 in an array list. 2 and 5 appear an odd number of times in the array list. So,
n
is 10.)
Sample Run 1
Enter an integer m: 1500
The smallest number n for m x n to be a perfect square is 15
m x n is 22500
Sample Run 2
Enter an integer m: 63
The smallest number n for m x n to be a perfect square is 7
m x n is 441
Class Name:
Exercise11_17
Answer is :
import java.util.Scanner;
public class Squares {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in); //instantiation of Scanner that will take inputs from the keyboard
//the try catch block below is for trapping error with the input
try {
System.out.print("Enter an integer m: "); //prompts the user to enter a number
int m = scan.nextInt(); //stores the user input to m
int n = 0; //initializing n
for(int i=1; ; i++) { //this loop only ends with the 'break keyword' which is why the 2nd argument is blank
int product = i * m;
double squareRoot = Math.sqrt(product);
if(squareRoot - Math.floor(squareRoot) == 0) {
//when the square root is just a whole number(when decimal part is just zero), it is a perfect square
n = i;
break; //loop ends here
}
}
System.out.println("The smallest number n for m * n to be a perfect square is " + n); //prints out n
System.out.println("m * n is " + (m*n)); //prints out product of m and n
}catch(IllegalArgumentException e) {
System.out.println("Invalid input!"); //prints out error message
}
}
}
But after that its showing the follwoing :
Exercise11_17.java:5: error: class Squares is public, should be declared in a file named Squares.java
public class Squares {
1 error
What Am i doing wrong? Can you correct me?
Thank You

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

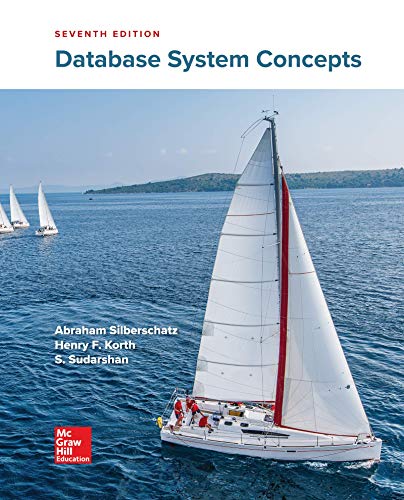
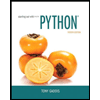
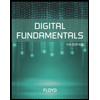
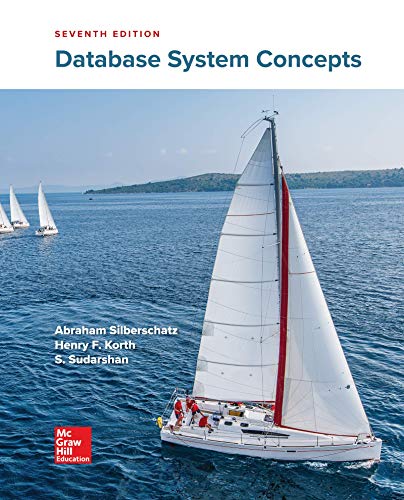
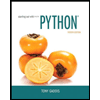
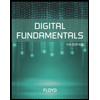
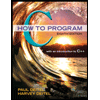
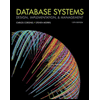
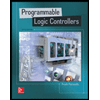